
This is a demonstration of two Choro Q Hybrid cars.
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /** 00002 * StarBoard Orange - Example application No.3 (Version 0.0.1) 00003 * Drive a CHORO Q HYBRID with wii nunchuk 00004 * 00005 * See also ... http://mbed.org/users/shintamainjp/notebook/starboard_example3_ja/ 00006 * See also ... http://mbed.org/users/shintamainjp/notebook/starboard_example3_en/ 00007 * 00008 * Copyright (C) 2010 Shinichiro Nakamura (CuBeatSystems) 00009 * http://shinta.main.jp/ 00010 */ 00011 00012 /* 00013 * Connection map. 00014 * 00015 * +---+----------------+---------+ 00016 * |Pin|Target |Direction| 00017 * +---+----------------+---------+ 00018 * |p21|IR transmitter |OUT | 00019 * +---+----------------+---------+ 00020 */ 00021 00022 /* 00023 * Include files. 00024 */ 00025 00026 #include <mbed.h> 00027 #include <algorithm> 00028 #include <ChoroQ.h> 00029 #include <I2CConfig.h> 00030 #include <WiiNunchuckReader.h> 00031 #include "appconf.h" 00032 00033 /* 00034 * Objects. 00035 */ 00036 00037 WiiNunchuckReader wn1(I2CPort_A::SDA, I2CPort_A::SCL); 00038 WiiNunchuckReader wn2(I2CPort_B::SDA, I2CPort_B::SCL); 00039 ChoroQ cq(p21); 00040 BusOut led(LED4, LED3, LED2, LED1); 00041 00042 /** 00043 * Display a splash screen. 00044 */ 00045 void splash(void) { 00046 // Do nothing. 00047 } 00048 00049 /** 00050 * Get an action from a coordinate. 00051 * 00052 * @param x X axis. 00053 * @param y Y axis. 00054 * @param dash State of dash. 00055 * 00056 * @return An action. 00057 */ 00058 ChoroQ::Action getAction(const int x, const int y, bool dash) { 00059 static const int MAX_X = 200; 00060 static const int MIN_X = 20; 00061 static const int MAX_Y = 200; 00062 static const int MIN_Y = 20; 00063 int px = ((x - MIN_X) * 100) / (MAX_X - MIN_X); 00064 int py = ((y - MIN_Y) * 100) / (MAX_Y - MIN_Y); 00065 px = std::max(0, std::min(100, px)) - 50; // Range of a value is -50 to +50 00066 py = std::max(0, std::min(100, py)) - 50; // Range of a value is -50 to +50 00067 00068 if ((std::abs(px) <= 10) && (std::abs(py) <= 10)) { 00069 return ChoroQ::Stop; 00070 } 00071 00072 if ((x == 0) && (y == 0)) { 00073 return ChoroQ::Stop; 00074 } 00075 00076 if (std::abs(px) < 10) { 00077 if (py < 0) { 00078 if (dash) { 00079 return ChoroQ::DownDash; 00080 } else { 00081 return ChoroQ::Down; 00082 } 00083 } else { 00084 if (dash) { 00085 return ChoroQ::UpDash; 00086 } else { 00087 return ChoroQ::Up; 00088 } 00089 } 00090 } 00091 if (std::abs(py) < 10) { 00092 if (px < -20) { 00093 return ChoroQ::Left; 00094 } 00095 if (20 < px) { 00096 return ChoroQ::Right; 00097 } 00098 } 00099 if ((10 < px) && (10 < py)) { 00100 if (dash) { 00101 return ChoroQ::UpRightDash; 00102 } else { 00103 return ChoroQ::UpRight; 00104 } 00105 } 00106 if ((px < -10) && (10 < py)) { 00107 if (dash) { 00108 return ChoroQ::UpLeftDash; 00109 } else { 00110 return ChoroQ::UpLeft; 00111 } 00112 } 00113 if ((px < -10) && (py < -10)) { 00114 if (dash) { 00115 return ChoroQ::DownLeftDash; 00116 } else { 00117 return ChoroQ::DownLeft; 00118 } 00119 } 00120 if ((10 < px) && (py < -10)) { 00121 if (dash) { 00122 return ChoroQ::DownRightDash; 00123 } else { 00124 return ChoroQ::DownRight; 00125 } 00126 } 00127 return ChoroQ::Stop; 00128 } 00129 00130 /** 00131 * Entry point. 00132 */ 00133 int main() { 00134 /* 00135 * Splash. 00136 */ 00137 splash(); 00138 00139 /* 00140 * Setup a configuration. 00141 */ 00142 appconf_t conf; 00143 appconf_init(&conf); 00144 if (appconf_read(&conf) != 0) { 00145 error("Please check the configuration."); 00146 } 00147 00148 /* 00149 * Application loop. 00150 */ 00151 while (true) { 00152 led = led + 1; 00153 wn1.RequestRead(); 00154 ChoroQ::Action ac1 = getAction(wn1.getJoyX(), wn1.getJoyY(), (wn1.getButtonZ() == 1) ? true : false); 00155 cq.execute(conf.ch1, ac1, false); 00156 wait_ms(25); 00157 00158 led = led + 1; 00159 wn2.RequestRead(); 00160 ChoroQ::Action ac2 = getAction(wn2.getJoyX(), wn2.getJoyY(), (wn2.getButtonZ() == 1) ? true : false); 00161 cq.execute(conf.ch2, ac2, false); 00162 wait_ms(25); 00163 } 00164 }
Generated on Wed Aug 3 2022 05:08:17 by
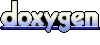