
This is a demonstration of two Choro Q Hybrid cars.
Embed:
(wiki syntax)
Show/hide line numbers
appconf.cpp
00001 #include "appconf.h" 00002 #include <ConfigFile.h> 00003 00004 LocalFileSystem fs_local("local"); 00005 00006 /* 00007 * Configuration File Example. 00008 * 00009 * Ch1=A 00010 * Ch2=B 00011 */ 00012 00013 #define CONFIG_FILENAME "/local/SETUP.CFG" 00014 00015 #define KEY_CH1 "Ch1" 00016 #define KEY_CH2 "Ch2" 00017 #define VALUE_CHANNEL_A "A" 00018 #define VALUE_CHANNEL_B "B" 00019 #define VALUE_CHANNEL_C "C" 00020 #define VALUE_CHANNEL_D "D" 00021 00022 /** 00023 * Initialize a configuration. 00024 * 00025 * @param p A pointer to a configuration structure. 00026 */ 00027 void appconf_init(appconf_t *p) { 00028 p->ch1 = ChoroQ::ChA; 00029 p->ch2 = ChoroQ::ChB; 00030 } 00031 00032 /** 00033 * Get a channel from the key. 00034 * 00035 * @param cf A pointer to a config file object. 00036 * @param p A pointer to a application config. 00037 * @param key The key. 00038 * @param ch A pointer to the channel. 00039 * 00040 * @return Return 0 if it succeed. 00041 */ 00042 static int getChannel(ConfigFile *cf, appconf_t *p, char *key, ChoroQ::Channel *ch) { 00043 char value[64]; 00044 if (!cf->getValue(key, value, sizeof(value))) { 00045 return -1; 00046 } 00047 00048 if (strcmp(value, VALUE_CHANNEL_A) == 0) { 00049 *ch = ChoroQ::ChA; 00050 return 0; 00051 } else if (strcmp(value, VALUE_CHANNEL_B) == 0) { 00052 *ch = ChoroQ::ChB; 00053 return 0; 00054 } else if (strcmp(value, VALUE_CHANNEL_C) == 0) { 00055 *ch = ChoroQ::ChC; 00056 return 0; 00057 } else if (strcmp(value, VALUE_CHANNEL_D) == 0) { 00058 *ch = ChoroQ::ChD; 00059 return 0; 00060 } else { 00061 return -2; 00062 } 00063 } 00064 00065 /** 00066 * Read a configuration. 00067 * 00068 * @param p A pointer to a configuration structure. 00069 * 00070 * @return Return 0 if read succeed. 00071 */ 00072 int appconf_read(appconf_t *p) { 00073 ConfigFile cfg; 00074 00075 if (!cfg.read(CONFIG_FILENAME)) { 00076 return -1; 00077 } 00078 00079 if (getChannel(&cfg, p, KEY_CH1, &p->ch1) != 0) { 00080 return -2; 00081 } 00082 if (getChannel(&cfg, p, KEY_CH2, &p->ch2) != 0) { 00083 return -3; 00084 } 00085 00086 return 0; 00087 }
Generated on Wed Aug 3 2022 05:08:17 by
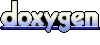