
For test
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 #include "mbed.h" 00002 00003 #define EXAMPLE_TCPCLIENT 00004 //#define EXAMPLE_TCPSERVER 00005 //#define EXAMPLE_UDPTEST 00006 //#define EXAMPLE_WIFITEST 00007 00008 //Serial pc(USBTX, USBRX); 00009 extern void setup(void); 00010 extern void loop(void); 00011 00012 int main () { 00013 setup(); 00014 while(1) { 00015 loop(); 00016 } 00017 } 00018 00019 #ifdef EXAMPLE_TCPCLIENT 00020 00021 #define SSID "ITEAD" 00022 #define PASSWORD "12345678" 00023 00024 00025 #include "ESP8266.h " 00026 00027 ESP8266 wifi(p28, p27); 00028 const char *server = "www.163.com"; 00029 char message[4096]; 00030 00031 void setup() 00032 { 00033 printf("setup begin\r\n"); 00034 00035 if(wifi.reset()) { 00036 printf("reset ok\r\n"); 00037 } else { 00038 printf("reset err\r\n"); 00039 } 00040 00041 if(wifi.setStationMode(SSID, PASSWORD)) { 00042 printf("setStationMode ok\r\n"); 00043 printf("AP:%s\r\n", wifi.showJAP().c_str()); 00044 printf("IP:%s\r\n", wifi.showIP().c_str()); 00045 } else { 00046 printf("setStationMode err\r\n"); 00047 } 00048 00049 printf("setup end\r\n"); 00050 } 00051 00052 void loop() 00053 { 00054 unsigned long t; 00055 if (wifi.ipConfig(ESP8266_COMM_TCP, server, 80)) { 00056 printf("connected and get /\r\n"); 00057 wifi.send("GET / HTTP/1.1\r\n\r\n"); 00058 t = millis(); 00059 printf("Recv:\r\n["); 00060 while(millis() - t <= 10000) { 00061 if(wifi.recvData(message)) { 00062 printf("%s", message); 00063 message[0] = 0; 00064 } 00065 } 00066 printf("]\r\n"); 00067 00068 } else { 00069 printf("connect failed\r\n"); 00070 } 00071 while(1); 00072 } 00073 00074 #endif 00075 00076 #ifdef EXAMPLE_TCPSERVER 00077 #define SSID "ITEAD" 00078 #define PASSWORD "12345678" 00079 00080 #include "ESP8266.h " 00081 00082 00083 ESP8266 wifi(p28, p27); 00084 00085 00086 void setup() 00087 { 00088 wifi.reset(); 00089 if(!wifi.setStationMode(SSID, PASSWORD)) { 00090 printf("init error\r\n"); 00091 } 00092 delay(8000); 00093 printf("IP address:%s\r\n", wifi.showIP().c_str()); 00094 printf("Access Point:%s\r\n", wifi.showJAP().c_str()); 00095 00096 delay(1000); 00097 wifi.confMux(1); 00098 delay(100); 00099 if(wifi.confServer(1, 80)) { 00100 printf("Server is set up\r\n"); 00101 } 00102 } 00103 00104 void loop() 00105 { 00106 char buf[500]; 00107 int iLen = wifi.recvData(buf); 00108 if(iLen > 0) { 00109 00110 printf("Recv:[%s]\r\n", buf); 00111 delay(100); 00112 00113 String cmd; 00114 cmd = "HTTP/1.1 200 OK\r\n"; 00115 cmd += "Content-Type: text/html\r\n"; 00116 cmd += "Connection: close\r\n"; 00117 cmd += "\r\n"; 00118 cmd += "This is a test server."; 00119 00120 wifi.send(wifi.getMuxID(),cmd); 00121 delay(300); 00122 wifi.closeMux(wifi.getMuxID()); 00123 delay(1000); 00124 } 00125 } 00126 00127 #endif 00128 00129 #ifdef EXAMPLE_UDPTEST 00130 #define SSID "ITEAD" 00131 #define PASSWORD "12345678" 00132 00133 00134 #include "ESP8266.h " 00135 00136 ESP8266 wifi(p28, p27); 00137 DigitalOut led1(LED1); 00138 00139 void setup() 00140 { 00141 wifi.reset(); 00142 if(!wifi.setStationMode(SSID, PASSWORD)) { 00143 printf("init error\r\n"); 00144 } 00145 delay(8000); 00146 printf("IP address:%s\r\n", wifi.showIP().c_str()); 00147 printf("Access Point:%s\r\n", wifi.showJAP().c_str()); 00148 00149 if (wifi.ipConfig(ESP8266_UDP, "172.16.5.12", 5416)) { //Connect to your server 00150 printf("Register UDP ok\r\n"); 00151 } else { 00152 printf("Register UDP err\r\n"); 00153 } 00154 wifi.send("setup done\r\n"); 00155 printf("setup done\r\n"); 00156 } 00157 00158 00159 00160 void loop() { 00161 char buf[10]; 00162 int iLen = 0; 00163 iLen = wifi.recvData(buf); 00164 if(iLen > 0) { 00165 //if you receive "HIGH" message, set the D13 to high voltage; and vice versa 00166 if (strcmp(buf, "HIGH") == 0) { 00167 printf("Set HIGH\r\n"); 00168 led1 = 1; 00169 } 00170 else if (strcmp(buf, "LOW") == 0) { 00171 printf("Set LOW\r\n"); 00172 led1 = 0; 00173 } 00174 } 00175 } 00176 00177 #endif 00178 00179 #ifdef EXAMPLE_WIFITEST 00180 #include "ESP8266.h " 00181 00182 #define SSID "ITEAD" 00183 #define PASSWORD "12345678" 00184 00185 ESP8266 wifi(p28, p27); 00186 00187 void setup(void) { 00188 wifi.reset(); 00189 if(!wifi.setStationMode(SSID, PASSWORD)) { 00190 printf("init error\r\n"); 00191 } 00192 delay(8000); 00193 printf("IP address:%s\r\n", wifi.showIP().c_str()); 00194 printf("Access Point:%s\r\n", wifi.showJAP().c_str()); 00195 } 00196 00197 void loop(void) { 00198 } 00199 00200 #endif
Generated on Tue Jul 12 2022 18:59:49 by
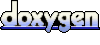