USB device stack - modified
Fork of USBDevice by
Embed:
(wiki syntax)
Show/hide line numbers
USBMouse.cpp
00001 /* Copyright (c) 2010-2011 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "stdint.h" 00020 #include "USBMouse.h" 00021 00022 bool USBMouse::update( int16_t x, int16_t y, uint8_t button, int8_t z ) 00023 { 00024 switch ( mouse_type ) 00025 { 00026 case REL_MOUSE: 00027 while ( x > 127 ) 00028 { 00029 if ( !mouseSend( 127, 0, button, z ) ) 00030 { 00031 return false; 00032 } 00033 00034 x = x - 127; 00035 } 00036 00037 while ( x < -128 ) 00038 { 00039 if ( !mouseSend( -128, 0, button, z ) ) 00040 { 00041 return false; 00042 } 00043 00044 x = x + 128; 00045 } 00046 00047 while ( y > 127 ) 00048 { 00049 if ( !mouseSend( 0, 127, button, z ) ) 00050 { 00051 return false; 00052 } 00053 00054 y = y - 127; 00055 } 00056 00057 while ( y < -128 ) 00058 { 00059 if ( !mouseSend( 0, -128, button, z ) ) 00060 { 00061 return false; 00062 } 00063 00064 y = y + 128; 00065 } 00066 00067 return mouseSend( x, y, button, z ); 00068 00069 case ABS_MOUSE: 00070 HID_REPORT report; 00071 report.data[0] = x & 0xff; 00072 report.data[1] = ( x >> 8 ) & 0xff; 00073 report.data[2] = y & 0xff; 00074 report.data[3] = ( y >> 8 ) & 0xff; 00075 report.data[4] = -z; 00076 report.data[5] = button & 0x07; 00077 report.length = 6; 00078 return send( &report ); 00079 00080 default: 00081 return false; 00082 } 00083 } 00084 00085 bool USBMouse::mouseSend( int8_t x, int8_t y, uint8_t buttons, int8_t z ) 00086 { 00087 HID_REPORT report; 00088 report.data[0] = buttons & 0x07; 00089 report.data[1] = x; 00090 report.data[2] = y; 00091 report.data[3] = -z; // >0 to scroll down, <0 to scroll up 00092 report.length = 4; 00093 return send( &report ); 00094 } 00095 00096 bool USBMouse::move( int16_t x, int16_t y ) 00097 { 00098 return update( x, y, button, 0 ); 00099 } 00100 00101 bool USBMouse::scroll( int8_t z ) 00102 { 00103 return update( 0, 0, button, z ); 00104 } 00105 00106 00107 bool USBMouse::doubleClick() 00108 { 00109 if ( !click( MOUSE_LEFT ) ) 00110 { 00111 return false; 00112 } 00113 00114 wait( 0.1 ); 00115 return click( MOUSE_LEFT ); 00116 } 00117 00118 bool USBMouse::click( uint8_t button ) 00119 { 00120 if ( !update( 0, 0, button, 0 ) ) 00121 { 00122 return false; 00123 } 00124 00125 wait( 0.01 ); 00126 return update( 0, 0, 0, 0 ); 00127 } 00128 00129 bool USBMouse::press( uint8_t button_ ) 00130 { 00131 button = button_ & 0x07; 00132 return update( 0, 0, button, 0 ); 00133 } 00134 00135 bool USBMouse::release( uint8_t button_ ) 00136 { 00137 button = ( button & ( ~button_ ) ) & 0x07; 00138 return update( 0, 0, button, 0 ); 00139 } 00140 00141 00142 uint8_t *USBMouse::reportDesc() 00143 { 00144 if ( mouse_type == REL_MOUSE ) 00145 { 00146 static uint8_t reportDescriptor[] = 00147 { 00148 USAGE_PAGE( 1 ), 0x01, // Genric Desktop 00149 USAGE( 1 ), 0x02, // Mouse 00150 COLLECTION( 1 ), 0x01, // Application 00151 USAGE( 1 ), 0x01, // Pointer 00152 COLLECTION( 1 ), 0x00, // Physical 00153 00154 REPORT_COUNT( 1 ), 0x03, 00155 REPORT_SIZE( 1 ), 0x01, 00156 USAGE_PAGE( 1 ), 0x09, // Buttons 00157 USAGE_MINIMUM( 1 ), 0x1, 00158 USAGE_MAXIMUM( 1 ), 0x3, 00159 LOGICAL_MINIMUM( 1 ), 0x00, 00160 LOGICAL_MAXIMUM( 1 ), 0x01, 00161 INPUT( 1 ), 0x02, 00162 REPORT_COUNT( 1 ), 0x01, 00163 REPORT_SIZE( 1 ), 0x05, 00164 INPUT( 1 ), 0x01, 00165 00166 REPORT_COUNT( 1 ), 0x03, 00167 REPORT_SIZE( 1 ), 0x08, 00168 USAGE_PAGE( 1 ), 0x01, 00169 USAGE( 1 ), 0x30, // X 00170 USAGE( 1 ), 0x31, // Y 00171 USAGE( 1 ), 0x38, // scroll 00172 LOGICAL_MINIMUM( 1 ), 0x81, 00173 LOGICAL_MAXIMUM( 1 ), 0x7f, 00174 INPUT( 1 ), 0x06, // Relative data 00175 00176 END_COLLECTION( 0 ), 00177 END_COLLECTION( 0 ), 00178 }; 00179 reportLength = sizeof( reportDescriptor ); 00180 return reportDescriptor; 00181 } 00182 else if ( mouse_type == ABS_MOUSE ) 00183 { 00184 static uint8_t reportDescriptor[] = 00185 { 00186 00187 USAGE_PAGE( 1 ), 0x01, // Generic Desktop 00188 USAGE( 1 ), 0x02, // Mouse 00189 COLLECTION( 1 ), 0x01, // Application 00190 USAGE( 1 ), 0x01, // Pointer 00191 COLLECTION( 1 ), 0x00, // Physical 00192 00193 USAGE_PAGE( 1 ), 0x01, // Generic Desktop 00194 USAGE( 1 ), 0x30, // X 00195 USAGE( 1 ), 0x31, // Y 00196 LOGICAL_MINIMUM( 1 ), 0x00, // 0 00197 LOGICAL_MAXIMUM( 2 ), 0xff, 0x7f, // 32767 00198 REPORT_SIZE( 1 ), 0x10, 00199 REPORT_COUNT( 1 ), 0x02, 00200 INPUT( 1 ), 0x02, // Data, Variable, Absolute 00201 00202 USAGE_PAGE( 1 ), 0x01, // Generic Desktop 00203 USAGE( 1 ), 0x38, // scroll 00204 LOGICAL_MINIMUM( 1 ), 0x81, // -127 00205 LOGICAL_MAXIMUM( 1 ), 0x7f, // 127 00206 REPORT_SIZE( 1 ), 0x08, 00207 REPORT_COUNT( 1 ), 0x01, 00208 INPUT( 1 ), 0x06, // Data, Variable, Relative 00209 00210 USAGE_PAGE( 1 ), 0x09, // Buttons 00211 USAGE_MINIMUM( 1 ), 0x01, 00212 USAGE_MAXIMUM( 1 ), 0x03, 00213 LOGICAL_MINIMUM( 1 ), 0x00, // 0 00214 LOGICAL_MAXIMUM( 1 ), 0x01, // 1 00215 REPORT_COUNT( 1 ), 0x03, 00216 REPORT_SIZE( 1 ), 0x01, 00217 INPUT( 1 ), 0x02, // Data, Variable, Absolute 00218 REPORT_COUNT( 1 ), 0x01, 00219 REPORT_SIZE( 1 ), 0x05, 00220 INPUT( 1 ), 0x01, // Constant 00221 00222 END_COLLECTION( 0 ), 00223 END_COLLECTION( 0 ) 00224 }; 00225 reportLength = sizeof( reportDescriptor ); 00226 return reportDescriptor; 00227 } 00228 00229 return NULL; 00230 } 00231 00232 #define DEFAULT_CONFIGURATION (1) 00233 #define TOTAL_DESCRIPTOR_LENGTH ((1 * CONFIGURATION_DESCRIPTOR_LENGTH) \ 00234 + (1 * INTERFACE_DESCRIPTOR_LENGTH) \ 00235 + (1 * HID_DESCRIPTOR_LENGTH) \ 00236 + (2 * ENDPOINT_DESCRIPTOR_LENGTH)) 00237 00238 uint8_t *USBMouse::configurationDesc() 00239 { 00240 static uint8_t configurationDescriptor[] = 00241 { 00242 CONFIGURATION_DESCRIPTOR_LENGTH,// bLength 00243 CONFIGURATION_DESCRIPTOR, // bDescriptorType 00244 LSB( TOTAL_DESCRIPTOR_LENGTH ), // wTotalLength (LSB) 00245 MSB( TOTAL_DESCRIPTOR_LENGTH ), // wTotalLength (MSB) 00246 0x01, // bNumInterfaces 00247 DEFAULT_CONFIGURATION, // bConfigurationValue 00248 0x00, // iConfiguration 00249 C_RESERVED | C_SELF_POWERED, // bmAttributes 00250 C_POWER( 0 ), // bMaxPowerHello World from Mbed 00251 00252 INTERFACE_DESCRIPTOR_LENGTH, // bLength 00253 INTERFACE_DESCRIPTOR, // bDescriptorType 00254 0x00, // bInterfaceNumber 00255 0x00, // bAlternateSetting 00256 0x02, // bNumEndpoints 00257 HID_CLASS, // bInterfaceClass 00258 1, // bInterfaceSubClass 00259 2, // bInterfaceProtocol (mouse) 00260 0x00, // iInterface 00261 00262 HID_DESCRIPTOR_LENGTH, // bLength 00263 HID_DESCRIPTOR, // bDescriptorType 00264 LSB( HID_VERSION_1_11 ), // bcdHID (LSB) 00265 MSB( HID_VERSION_1_11 ), // bcdHID (MSB) 00266 0x00, // bCountryCode 00267 0x01, // bNumDescriptors 00268 REPORT_DESCRIPTOR, // bDescriptorType 00269 LSB( reportDescLength() ), // wDescriptorLength (LSB) 00270 MSB( reportDescLength() ), // wDescriptorLength (MSB) 00271 00272 ENDPOINT_DESCRIPTOR_LENGTH, // bLength 00273 ENDPOINT_DESCRIPTOR, // bDescriptorType 00274 PHY_TO_DESC( EPINT_IN ), // bEndpointAddress 00275 E_INTERRUPT, // bmAttributes 00276 LSB( MAX_PACKET_SIZE_EPINT ), // wMaxPacketSize (LSB) 00277 MSB( MAX_PACKET_SIZE_EPINT ), // wMaxPacketSize (MSB) 00278 1, // bInterval (milliseconds) 00279 00280 ENDPOINT_DESCRIPTOR_LENGTH, // bLength 00281 ENDPOINT_DESCRIPTOR, // bDescriptorType 00282 PHY_TO_DESC( EPINT_OUT ), // bEndpointAddress 00283 E_INTERRUPT, // bmAttributes 00284 LSB( MAX_PACKET_SIZE_EPINT ), // wMaxPacketSize (LSB) 00285 MSB( MAX_PACKET_SIZE_EPINT ), // wMaxPacketSize (MSB) 00286 1, // bInterval (milliseconds) 00287 }; 00288 return configurationDescriptor; 00289 } 00290
Generated on Sat Jul 16 2022 03:17:41 by
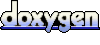