USB device stack - modified
Fork of USBDevice by
Embed:
(wiki syntax)
Show/hide line numbers
USBAudio.cpp
00001 /* Copyright (c) 2010-2011 mbed.org, MIT License 00002 * 00003 * Permission is hereby granted, free of charge, to any person obtaining a copy of this software 00004 * and associated documentation files (the "Software"), to deal in the Software without 00005 * restriction, including without limitation the rights to use, copy, modify, merge, publish, 00006 * distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the 00007 * Software is furnished to do so, subject to the following conditions: 00008 * 00009 * The above copyright notice and this permission notice shall be included in all copies or 00010 * substantial portions of the Software. 00011 * 00012 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING 00013 * BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND 00014 * NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, 00015 * DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00016 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE. 00017 */ 00018 00019 #include "stdint.h" 00020 #include "USBAudio.h" 00021 #include "USBAudio_Types.h" 00022 00023 00024 00025 USBAudio::USBAudio( uint32_t frequency_in, uint8_t channel_nb_in, uint32_t frequency_out, uint8_t channel_nb_out, uint16_t vendor_id, uint16_t product_id, uint16_t product_release ): USBDevice( vendor_id, product_id, product_release ) 00026 { 00027 mute = 0; 00028 volCur = 0x0080; 00029 volMin = 0x0000; 00030 volMax = 0x0100; 00031 volRes = 0x0004; 00032 available = false; 00033 FREQ_IN = frequency_in; 00034 FREQ_OUT = frequency_out; 00035 this->channel_nb_in = channel_nb_in; 00036 this->channel_nb_out = channel_nb_out; 00037 // stereo -> *2, mono -> *1 00038 PACKET_SIZE_ISO_IN = ( FREQ_IN / 500 ) * channel_nb_in; 00039 PACKET_SIZE_ISO_OUT = ( FREQ_OUT / 500 ) * channel_nb_out; 00040 // STEREO -> left and right 00041 channel_config_in = ( channel_nb_in == 1 ) ? CHANNEL_M : CHANNEL_L + CHANNEL_R; 00042 channel_config_out = ( channel_nb_out == 1 ) ? CHANNEL_M : CHANNEL_L + CHANNEL_R; 00043 SOF_handler = false; 00044 buf_stream_out = NULL; 00045 buf_stream_in = NULL; 00046 interruptOUT = false; 00047 writeIN = false; 00048 interruptIN = false; 00049 available = false; 00050 volume = 0; 00051 // connect the device 00052 USBDevice::connect(); 00053 } 00054 00055 bool USBAudio::read( uint8_t *buf ) 00056 { 00057 buf_stream_in = buf; 00058 SOF_handler = false; 00059 00060 while ( !available || !SOF_handler ); 00061 00062 available = false; 00063 return true; 00064 } 00065 00066 bool USBAudio::readNB( uint8_t *buf ) 00067 { 00068 buf_stream_in = buf; 00069 SOF_handler = false; 00070 00071 while ( !SOF_handler ); 00072 00073 if ( available ) 00074 { 00075 available = false; 00076 buf_stream_in = NULL; 00077 return true; 00078 } 00079 00080 return false; 00081 } 00082 00083 bool USBAudio::readWrite( uint8_t *buf_read, uint8_t *buf_write ) 00084 { 00085 buf_stream_in = buf_read; 00086 SOF_handler = false; 00087 writeIN = false; 00088 00089 if ( interruptIN ) 00090 { 00091 USBDevice::writeNB( EP3IN, buf_write, PACKET_SIZE_ISO_OUT, PACKET_SIZE_ISO_OUT ); 00092 } 00093 else 00094 { 00095 buf_stream_out = buf_write; 00096 } 00097 00098 while ( !available ); 00099 00100 if ( interruptIN ) 00101 { 00102 while ( !writeIN ); 00103 } 00104 00105 while ( !SOF_handler ); 00106 00107 return true; 00108 } 00109 00110 00111 bool USBAudio::write( uint8_t *buf ) 00112 { 00113 writeIN = false; 00114 SOF_handler = false; 00115 00116 if ( interruptIN ) 00117 { 00118 USBDevice::writeNB( EP3IN, buf, PACKET_SIZE_ISO_OUT, PACKET_SIZE_ISO_OUT ); 00119 } 00120 else 00121 { 00122 buf_stream_out = buf; 00123 } 00124 00125 while ( !SOF_handler ); 00126 00127 if ( interruptIN ) 00128 { 00129 while ( !writeIN ); 00130 } 00131 00132 return true; 00133 } 00134 00135 00136 float USBAudio::getVolume() 00137 { 00138 return ( mute ) ? 0.0 : volume; 00139 } 00140 00141 00142 bool USBAudio::EP3_OUT_callback() 00143 { 00144 uint32_t size = 0; 00145 interruptOUT = true; 00146 00147 if ( buf_stream_in != NULL ) 00148 { 00149 readEP( EP3OUT, ( uint8_t * )buf_stream_in, &size, PACKET_SIZE_ISO_IN ); 00150 available = true; 00151 buf_stream_in = NULL; 00152 } 00153 00154 readStart( EP3OUT, PACKET_SIZE_ISO_IN ); 00155 return false; 00156 } 00157 00158 00159 bool USBAudio::EP3_IN_callback() 00160 { 00161 interruptIN = true; 00162 writeIN = true; 00163 return true; 00164 } 00165 00166 00167 00168 // Called in ISR context on each start of frame 00169 void USBAudio::SOF( int frameNumber ) 00170 { 00171 uint32_t size = 0; 00172 00173 if ( !interruptOUT ) 00174 { 00175 // read the isochronous endpoint 00176 if ( buf_stream_in != NULL ) 00177 { 00178 if ( USBDevice::readEP_NB( EP3OUT, ( uint8_t * )buf_stream_in, &size, PACKET_SIZE_ISO_IN ) ) 00179 { 00180 if ( size ) 00181 { 00182 available = true; 00183 readStart( EP3OUT, PACKET_SIZE_ISO_IN ); 00184 buf_stream_in = NULL; 00185 } 00186 } 00187 } 00188 } 00189 00190 if ( !interruptIN ) 00191 { 00192 // write if needed 00193 if ( buf_stream_out != NULL ) 00194 { 00195 USBDevice::writeNB( EP3IN, ( uint8_t * )buf_stream_out, PACKET_SIZE_ISO_OUT, PACKET_SIZE_ISO_OUT ); 00196 buf_stream_out = NULL; 00197 } 00198 } 00199 00200 SOF_handler = true; 00201 } 00202 00203 00204 // Called in ISR context 00205 // Set configuration. Return false if the configuration is not supported. 00206 bool USBAudio::USBCallback_setConfiguration( uint8_t configuration ) 00207 { 00208 if ( configuration != DEFAULT_CONFIGURATION ) 00209 { 00210 return false; 00211 } 00212 00213 // Configure isochronous endpoint 00214 realiseEndpoint( EP3OUT, PACKET_SIZE_ISO_IN, ISOCHRONOUS ); 00215 realiseEndpoint( EP3IN, PACKET_SIZE_ISO_OUT, ISOCHRONOUS ); 00216 // activate readings on this endpoint 00217 readStart( EP3OUT, PACKET_SIZE_ISO_IN ); 00218 return true; 00219 } 00220 00221 00222 // Called in ISR context 00223 // Set alternate setting. Return false if the alternate setting is not supported 00224 bool USBAudio::USBCallback_setInterface( uint16_t interface, uint8_t alternate ) 00225 { 00226 if ( interface == 0 && alternate == 0 ) 00227 { 00228 return true; 00229 } 00230 00231 if ( interface == 1 && ( alternate == 0 || alternate == 1 ) ) 00232 { 00233 return true; 00234 } 00235 00236 if ( interface == 2 && ( alternate == 0 || alternate == 1 ) ) 00237 { 00238 return true; 00239 } 00240 00241 return false; 00242 } 00243 00244 00245 00246 // Called in ISR context 00247 // Called by USBDevice on Endpoint0 request 00248 // This is used to handle extensions to standard requests and class specific requests. 00249 // Return true if class handles this request 00250 bool USBAudio::USBCallback_request() 00251 { 00252 bool success = false; 00253 CONTROL_TRANSFER *transfer = getTransferPtr(); 00254 00255 // Process class-specific requests 00256 if ( transfer->setup.bmRequestType.Type == CLASS_TYPE ) 00257 { 00258 // Feature Unit: Interface = 0, ID = 2 00259 if ( transfer->setup.wIndex == 0x0200 ) 00260 { 00261 // Master Channel 00262 if ( ( transfer->setup.wValue & 0xff ) == 0 ) 00263 { 00264 switch ( transfer->setup.wValue >> 8 ) 00265 { 00266 case MUTE_CONTROL: 00267 switch ( transfer->setup.bRequest ) 00268 { 00269 case REQUEST_GET_CUR: 00270 transfer->remaining = 1; 00271 transfer->ptr = &mute; 00272 transfer->direction = DEVICE_TO_HOST; 00273 success = true; 00274 break; 00275 00276 case REQUEST_SET_CUR: 00277 transfer->remaining = 1; 00278 transfer->notify = true; 00279 transfer->direction = HOST_TO_DEVICE; 00280 success = true; 00281 break; 00282 00283 default: 00284 break; 00285 } 00286 00287 break; 00288 00289 case VOLUME_CONTROL: 00290 switch ( transfer->setup.bRequest ) 00291 { 00292 case REQUEST_GET_CUR: 00293 transfer->remaining = 2; 00294 transfer->ptr = ( uint8_t * )&volCur; 00295 transfer->direction = DEVICE_TO_HOST; 00296 success = true; 00297 break; 00298 00299 case REQUEST_GET_MIN: 00300 transfer->remaining = 2; 00301 transfer->ptr = ( uint8_t * )&volMin; 00302 transfer->direction = DEVICE_TO_HOST; 00303 success = true; 00304 break; 00305 00306 case REQUEST_GET_MAX: 00307 transfer->remaining = 2; 00308 transfer->ptr = ( uint8_t * )&volMax; 00309 transfer->direction = DEVICE_TO_HOST; 00310 success = true; 00311 break; 00312 00313 case REQUEST_GET_RES: 00314 transfer->remaining = 2; 00315 transfer->ptr = ( uint8_t * )&volRes; 00316 transfer->direction = DEVICE_TO_HOST; 00317 success = true; 00318 break; 00319 00320 case REQUEST_SET_CUR: 00321 transfer->remaining = 2; 00322 transfer->notify = true; 00323 transfer->direction = HOST_TO_DEVICE; 00324 success = true; 00325 break; 00326 00327 case REQUEST_SET_MIN: 00328 transfer->remaining = 2; 00329 transfer->notify = true; 00330 transfer->direction = HOST_TO_DEVICE; 00331 success = true; 00332 break; 00333 00334 case REQUEST_SET_MAX: 00335 transfer->remaining = 2; 00336 transfer->notify = true; 00337 transfer->direction = HOST_TO_DEVICE; 00338 success = true; 00339 break; 00340 00341 case REQUEST_SET_RES: 00342 transfer->remaining = 2; 00343 transfer->notify = true; 00344 transfer->direction = HOST_TO_DEVICE; 00345 success = true; 00346 break; 00347 } 00348 00349 break; 00350 00351 default: 00352 break; 00353 } 00354 } 00355 } 00356 } 00357 00358 return success; 00359 } 00360 00361 00362 // Called in ISR context when a data OUT stage has been performed 00363 void USBAudio::USBCallback_requestCompleted( uint8_t *buf, uint32_t length ) 00364 { 00365 if ( ( length == 1 ) || ( length == 2 ) ) 00366 { 00367 uint16_t data = ( length == 1 ) ? *buf : *( ( uint16_t * )buf ); 00368 CONTROL_TRANSFER *transfer = getTransferPtr(); 00369 00370 switch ( transfer->setup.wValue >> 8 ) 00371 { 00372 case MUTE_CONTROL: 00373 switch ( transfer->setup.bRequest ) 00374 { 00375 case REQUEST_SET_CUR: 00376 mute = data & 0xff; 00377 updateVol.call(); 00378 break; 00379 00380 default: 00381 break; 00382 } 00383 00384 break; 00385 00386 case VOLUME_CONTROL: 00387 switch ( transfer->setup.bRequest ) 00388 { 00389 case REQUEST_SET_CUR: 00390 volCur = data; 00391 volume = ( float )volCur / ( float )volMax; 00392 updateVol.call(); 00393 break; 00394 00395 default: 00396 break; 00397 } 00398 00399 break; 00400 00401 default: 00402 break; 00403 } 00404 } 00405 } 00406 00407 00408 00409 #define TOTAL_DESCRIPTOR_LENGTH ((1 * CONFIGURATION_DESCRIPTOR_LENGTH) \ 00410 + (5 * INTERFACE_DESCRIPTOR_LENGTH) \ 00411 + (1 * CONTROL_INTERFACE_DESCRIPTOR_LENGTH + 1) \ 00412 + (2 * INPUT_TERMINAL_DESCRIPTOR_LENGTH) \ 00413 + (1 * FEATURE_UNIT_DESCRIPTOR_LENGTH) \ 00414 + (2 * OUTPUT_TERMINAL_DESCRIPTOR_LENGTH) \ 00415 + (2 * STREAMING_INTERFACE_DESCRIPTOR_LENGTH) \ 00416 + (2 * FORMAT_TYPE_I_DESCRIPTOR_LENGTH) \ 00417 + (2 * (ENDPOINT_DESCRIPTOR_LENGTH + 2)) \ 00418 + (2 * STREAMING_ENDPOINT_DESCRIPTOR_LENGTH) ) 00419 00420 #define TOTAL_CONTROL_INTF_LENGTH (CONTROL_INTERFACE_DESCRIPTOR_LENGTH + 1 + \ 00421 2*INPUT_TERMINAL_DESCRIPTOR_LENGTH + \ 00422 FEATURE_UNIT_DESCRIPTOR_LENGTH + \ 00423 2*OUTPUT_TERMINAL_DESCRIPTOR_LENGTH) 00424 00425 uint8_t *USBAudio::configurationDesc() 00426 { 00427 static uint8_t configDescriptor[] = 00428 { 00429 // Configuration 1 00430 CONFIGURATION_DESCRIPTOR_LENGTH, // bLength 00431 CONFIGURATION_DESCRIPTOR, // bDescriptorType 00432 LSB( TOTAL_DESCRIPTOR_LENGTH ), // wTotalLength (LSB) 00433 MSB( TOTAL_DESCRIPTOR_LENGTH ), // wTotalLength (MSB) 00434 0x03, // bNumInterfaces 00435 DEFAULT_CONFIGURATION, // bConfigurationValue 00436 0x00, // iConfiguration 00437 0x80, // bmAttributes 00438 50, // bMaxPower 00439 00440 // Interface 0, Alternate Setting 0, Audio Control 00441 INTERFACE_DESCRIPTOR_LENGTH, // bLength 00442 INTERFACE_DESCRIPTOR, // bDescriptorType 00443 0x00, // bInterfaceNumber 00444 0x00, // bAlternateSetting 00445 0x00, // bNumEndpoints 00446 AUDIO_CLASS, // bInterfaceClass 00447 SUBCLASS_AUDIOCONTROL, // bInterfaceSubClass 00448 0x00, // bInterfaceProtocol 00449 0x00, // iInterface 00450 00451 00452 // Audio Control Interface 00453 CONTROL_INTERFACE_DESCRIPTOR_LENGTH + 1,// bLength 00454 INTERFACE_DESCRIPTOR_TYPE, // bDescriptorType 00455 CONTROL_HEADER, // bDescriptorSubtype 00456 LSB( 0x0100 ), // bcdADC (LSB) 00457 MSB( 0x0100 ), // bcdADC (MSB) 00458 LSB( TOTAL_CONTROL_INTF_LENGTH ), // wTotalLength 00459 MSB( TOTAL_CONTROL_INTF_LENGTH ), // wTotalLength 00460 0x02, // bInCollection 00461 0x01, // baInterfaceNr 00462 0x02, // baInterfaceNr 00463 00464 // Audio Input Terminal (Speaker) 00465 INPUT_TERMINAL_DESCRIPTOR_LENGTH, // bLength 00466 INTERFACE_DESCRIPTOR_TYPE, // bDescriptorType 00467 CONTROL_INPUT_TERMINAL, // bDescriptorSubtype 00468 0x01, // bTerminalID 00469 LSB( TERMINAL_USB_STREAMING ), // wTerminalType 00470 MSB( TERMINAL_USB_STREAMING ), // wTerminalType 00471 0x00, // bAssocTerminal 00472 channel_nb_in, // bNrChannels 00473 LSB( channel_config_in ), // wChannelConfig 00474 MSB( channel_config_in ), // wChannelConfig 00475 0x00, // iChannelNames 00476 0x00, // iTerminal 00477 00478 // Audio Feature Unit (Speaker) 00479 FEATURE_UNIT_DESCRIPTOR_LENGTH, // bLength 00480 INTERFACE_DESCRIPTOR_TYPE, // bDescriptorType 00481 CONTROL_FEATURE_UNIT, // bDescriptorSubtype 00482 0x02, // bUnitID 00483 0x01, // bSourceID 00484 0x01, // bControlSize 00485 CONTROL_MUTE | 00486 CONTROL_VOLUME, // bmaControls(0) 00487 0x00, // bmaControls(1) 00488 0x00, // iTerminal 00489 00490 // Audio Output Terminal (Speaker) 00491 OUTPUT_TERMINAL_DESCRIPTOR_LENGTH, // bLength 00492 INTERFACE_DESCRIPTOR_TYPE, // bDescriptorType 00493 CONTROL_OUTPUT_TERMINAL, // bDescriptorSubtype 00494 0x03, // bTerminalID 00495 LSB( TERMINAL_SPEAKER ), // wTerminalType 00496 MSB( TERMINAL_SPEAKER ), // wTerminalType 00497 0x00, // bAssocTerminal 00498 0x02, // bSourceID 00499 0x00, // iTerminal 00500 00501 00502 // Audio Input Terminal (Microphone) 00503 INPUT_TERMINAL_DESCRIPTOR_LENGTH, // bLength 00504 INTERFACE_DESCRIPTOR_TYPE, // bDescriptorType 00505 CONTROL_INPUT_TERMINAL, // bDescriptorSubtype 00506 0x04, // bTerminalID 00507 LSB( TERMINAL_MICROPHONE ), // wTerminalType 00508 MSB( TERMINAL_MICROPHONE ), // wTerminalType 00509 0x00, // bAssocTerminal 00510 channel_nb_out, // bNrChannels 00511 LSB( channel_config_out ), // wChannelConfig 00512 MSB( channel_config_out ), // wChannelConfig 00513 0x00, // iChannelNames 00514 0x00, // iTerminal 00515 00516 // Audio Output Terminal (Microphone) 00517 OUTPUT_TERMINAL_DESCRIPTOR_LENGTH, // bLength 00518 INTERFACE_DESCRIPTOR_TYPE, // bDescriptorType 00519 CONTROL_OUTPUT_TERMINAL, // bDescriptorSubtype 00520 0x05, // bTerminalID 00521 LSB( TERMINAL_USB_STREAMING ), // wTerminalType 00522 MSB( TERMINAL_USB_STREAMING ), // wTerminalType 00523 0x00, // bAssocTerminal 00524 0x04, // bSourceID 00525 0x00, // iTerminal 00526 00527 00528 00529 00530 00531 00532 // Interface 1, Alternate Setting 0, Audio Streaming - Zero Bandwith 00533 INTERFACE_DESCRIPTOR_LENGTH, // bLength 00534 INTERFACE_DESCRIPTOR, // bDescriptorType 00535 0x01, // bInterfaceNumber 00536 0x00, // bAlternateSetting 00537 0x00, // bNumEndpoints 00538 AUDIO_CLASS, // bInterfaceClass 00539 SUBCLASS_AUDIOSTREAMING, // bInterfaceSubClass 00540 0x00, // bInterfaceProtocol 00541 0x00, // iInterface 00542 00543 // Interface 1, Alternate Setting 1, Audio Streaming - Operational 00544 INTERFACE_DESCRIPTOR_LENGTH, // bLength 00545 INTERFACE_DESCRIPTOR, // bDescriptorType 00546 0x01, // bInterfaceNumber 00547 0x01, // bAlternateSetting 00548 0x01, // bNumEndpoints 00549 AUDIO_CLASS, // bInterfaceClass 00550 SUBCLASS_AUDIOSTREAMING, // bInterfaceSubClass 00551 0x00, // bInterfaceProtocol 00552 0x00, // iInterface 00553 00554 // Audio Streaming Interface 00555 STREAMING_INTERFACE_DESCRIPTOR_LENGTH, // bLength 00556 INTERFACE_DESCRIPTOR_TYPE, // bDescriptorType 00557 STREAMING_GENERAL, // bDescriptorSubtype 00558 0x01, // bTerminalLink 00559 0x00, // bDelay 00560 LSB( FORMAT_PCM ), // wFormatTag 00561 MSB( FORMAT_PCM ), // wFormatTag 00562 00563 // Audio Type I Format 00564 FORMAT_TYPE_I_DESCRIPTOR_LENGTH, // bLength 00565 INTERFACE_DESCRIPTOR_TYPE, // bDescriptorType 00566 STREAMING_FORMAT_TYPE, // bDescriptorSubtype 00567 FORMAT_TYPE_I, // bFormatType 00568 channel_nb_in, // bNrChannels 00569 0x02, // bSubFrameSize 00570 16, // bBitResolution 00571 0x01, // bSamFreqType 00572 LSB( FREQ_IN ), // tSamFreq 00573 ( FREQ_IN >> 8 ) & 0xff, // tSamFreq 00574 ( FREQ_IN >> 16 ) & 0xff, // tSamFreq 00575 00576 // Endpoint - Standard Descriptor 00577 ENDPOINT_DESCRIPTOR_LENGTH + 2, // bLength 00578 ENDPOINT_DESCRIPTOR, // bDescriptorType 00579 PHY_TO_DESC( EPISO_OUT ), // bEndpointAddress 00580 E_ISOCHRONOUS, // bmAttributes 00581 LSB( PACKET_SIZE_ISO_IN ), // wMaxPacketSize 00582 MSB( PACKET_SIZE_ISO_IN ), // wMaxPacketSize 00583 0x01, // bInterval 00584 0x00, // bRefresh 00585 0x00, // bSynchAddress 00586 00587 // Endpoint - Audio Streaming 00588 STREAMING_ENDPOINT_DESCRIPTOR_LENGTH, // bLength 00589 ENDPOINT_DESCRIPTOR_TYPE, // bDescriptorType 00590 ENDPOINT_GENERAL, // bDescriptor 00591 0x00, // bmAttributes 00592 0x00, // bLockDelayUnits 00593 LSB( 0x0000 ), // wLockDelay 00594 MSB( 0x0000 ), // wLockDelay 00595 00596 00597 00598 00599 00600 00601 00602 // Interface 1, Alternate Setting 0, Audio Streaming - Zero Bandwith 00603 INTERFACE_DESCRIPTOR_LENGTH, // bLength 00604 INTERFACE_DESCRIPTOR, // bDescriptorType 00605 0x02, // bInterfaceNumber 00606 0x00, // bAlternateSetting 00607 0x00, // bNumEndpoints 00608 AUDIO_CLASS, // bInterfaceClass 00609 SUBCLASS_AUDIOSTREAMING, // bInterfaceSubClass 00610 0x00, // bInterfaceProtocol 00611 0x00, // iInterface 00612 00613 // Interface 1, Alternate Setting 1, Audio Streaming - Operational 00614 INTERFACE_DESCRIPTOR_LENGTH, // bLength 00615 INTERFACE_DESCRIPTOR, // bDescriptorType 00616 0x02, // bInterfaceNumber 00617 0x01, // bAlternateSetting 00618 0x01, // bNumEndpoints 00619 AUDIO_CLASS, // bInterfaceClass 00620 SUBCLASS_AUDIOSTREAMING, // bInterfaceSubClass 00621 0x00, // bInterfaceProtocol 00622 0x00, // iInterface 00623 00624 // Audio Streaming Interface 00625 STREAMING_INTERFACE_DESCRIPTOR_LENGTH, // bLength 00626 INTERFACE_DESCRIPTOR_TYPE, // bDescriptorType 00627 SUBCLASS_AUDIOCONTROL, // bDescriptorSubtype 00628 0x05, // bTerminalLink (output terminal microphone) 00629 0x01, // bDelay 00630 0x01, // wFormatTag 00631 0x00, // wFormatTag 00632 00633 // Audio Type I Format 00634 FORMAT_TYPE_I_DESCRIPTOR_LENGTH, // bLength 00635 INTERFACE_DESCRIPTOR_TYPE, // bDescriptorType 00636 SUBCLASS_AUDIOSTREAMING, // bDescriptorSubtype 00637 FORMAT_TYPE_I, // bFormatType 00638 channel_nb_out, // bNrChannels 00639 0x02, // bSubFrameSize 00640 0x10, // bBitResolution 00641 0x01, // bSamFreqType 00642 LSB( FREQ_OUT ), // tSamFreq 00643 ( FREQ_OUT >> 8 ) & 0xff, // tSamFreq 00644 ( FREQ_OUT >> 16 ) & 0xff, // tSamFreq 00645 00646 // Endpoint - Standard Descriptor 00647 ENDPOINT_DESCRIPTOR_LENGTH + 2, // bLength 00648 ENDPOINT_DESCRIPTOR, // bDescriptorType 00649 PHY_TO_DESC( EPISO_IN ), // bEndpointAddress 00650 E_ISOCHRONOUS, // bmAttributes 00651 LSB( PACKET_SIZE_ISO_OUT ), // wMaxPacketSize 00652 MSB( PACKET_SIZE_ISO_OUT ), // wMaxPacketSize 00653 0x01, // bInterval 00654 0x00, // bRefresh 00655 0x00, // bSynchAddress 00656 00657 // Endpoint - Audio Streaming 00658 STREAMING_ENDPOINT_DESCRIPTOR_LENGTH, // bLength 00659 ENDPOINT_DESCRIPTOR_TYPE, // bDescriptorType 00660 ENDPOINT_GENERAL, // bDescriptor 00661 0x00, // bmAttributes 00662 0x00, // bLockDelayUnits 00663 LSB( 0x0000 ), // wLockDelay 00664 MSB( 0x0000 ), // wLockDelay 00665 00666 // Terminator 00667 0 // bLength 00668 }; 00669 return configDescriptor; 00670 } 00671 00672 uint8_t *USBAudio::stringIinterfaceDesc() 00673 { 00674 static uint8_t stringIinterfaceDescriptor[] = 00675 { 00676 0x0c, //bLength 00677 STRING_DESCRIPTOR, //bDescriptorType 0x03 00678 'A', 0, 'u', 0, 'd', 0, 'i', 0, 'o', 0 //bString iInterface - Audio 00679 }; 00680 return stringIinterfaceDescriptor; 00681 } 00682 00683 uint8_t *USBAudio::stringIproductDesc() 00684 { 00685 static uint8_t stringIproductDescriptor[] = 00686 { 00687 0x16, //bLength 00688 STRING_DESCRIPTOR, //bDescriptorType 0x03 00689 'M', 0, 'b', 0, 'e', 0, 'd', 0, ' ', 0, 'A', 0, 'u', 0, 'd', 0, 'i', 0, 'o', 0 //bString iProduct - Mbed Audio 00690 }; 00691 return stringIproductDescriptor; 00692 } 00693
Generated on Sat Jul 16 2022 03:17:41 by
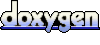