
teste
Embed:
(wiki syntax)
Show/hide line numbers
TextDisplay.h
00001 // ST7735 8 Bit SPI Library 00002 00003 #ifndef MBED_TEXTDISPLAY_H 00004 #define MBED_TEXTDISPLAY_H 00005 00006 #include "mbed.h" 00007 00008 class TextDisplay : public Stream { 00009 public: 00010 00011 // functions needing implementation in derived implementation class 00012 /** Create a TextDisplay interface 00013 * 00014 * @param name The name used in the path to access the strean through the filesystem 00015 */ 00016 TextDisplay(const char *name = NULL); 00017 00018 /** output a character at the given position 00019 * 00020 * @param column column where charater must be written 00021 * @param row where character must be written 00022 * @param c the character to be written to the TextDisplay 00023 */ 00024 virtual void character(int column, int row, int c) = 0; 00025 00026 /** return number if rows on TextDisplay 00027 * @result number of rows 00028 */ 00029 virtual int rows() = 0; 00030 00031 /** return number if columns on TextDisplay 00032 * @result number of rows 00033 */ 00034 virtual int columns() = 0; 00035 00036 // functions that come for free, but can be overwritten 00037 00038 /** redirect output from a stream (stoud, sterr) to display 00039 * @param stream stream that shall be redirected to the TextDisplay 00040 */ 00041 virtual bool claim (FILE *stream); 00042 00043 /** clear screen 00044 */ 00045 virtual void cls(); 00046 virtual void locate(int column, int row); 00047 virtual void foreground(uint16_t colour); 00048 virtual void background(uint16_t colour); 00049 // putc (from Stream) 00050 // printf (from Stream) 00051 00052 protected: 00053 00054 virtual int _putc(int value); 00055 virtual int _getc(); 00056 00057 // character location 00058 uint16_t _column; 00059 uint16_t _row; 00060 00061 // colours 00062 uint16_t _foreground; 00063 uint16_t _background; 00064 char *_path; 00065 }; 00066 00067 #endif
Generated on Tue Jul 12 2022 23:14:00 by
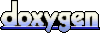