
teste
Embed:
(wiki syntax)
Show/hide line numbers
ST7735_TFT.h
00001 // ST7735 8 Bit SPI Library 00002 00003 #ifndef MBED_ST7735_TFT_H 00004 #define MBED_ST7735_TFT_H 00005 00006 #include "mbed.h" 00007 #include "GraphicsDisplay.h" 00008 #include "BurstSPI.h" 00009 00010 #define RGB(r,g,b) (((r&0xF8)<<8)|((g&0xFC)<<3)|((b&0xF8)>>3)) //5 red | 6 green | 5 blue 00011 00012 /*define ST7735 Commands */ 00013 00014 #define ST7735_NOP 0x0 00015 #define ST7735_SWRESET 0x01 00016 #define ST7735_RDDID 0x04 00017 #define ST7735_RDDST 0x09 00018 00019 #define ST7735_SLPIN 0x10 00020 #define ST7735_SLPOUT 0x11 00021 #define ST7735_PTLON 0x12 00022 #define ST7735_NORON 0x13 00023 00024 #define ST7735_INVOFF 0x20 00025 #define ST7735_INVON 0x21 00026 #define ST7735_DISPOFF 0x28 00027 #define ST7735_DISPON 0x29 00028 #define ST7735_CASET 0x2A 00029 #define ST7735_RASET 0x2B 00030 #define ST7735_RAMWR 0x2C 00031 #define ST7735_RAMRD 0x2E 00032 00033 #define ST7735_COLMOD 0x3A 00034 #define ST7735_MADCTL 0x36 00035 00036 00037 #define ST7735_FRMCTR1 0xB1 00038 #define ST7735_FRMCTR2 0xB2 00039 #define ST7735_FRMCTR3 0xB3 00040 #define ST7735_INVCTR 0xB4 00041 #define ST7735_DISSET5 0xB6 00042 00043 #define ST7735_PWCTR1 0xC0 00044 #define ST7735_PWCTR2 0xC1 00045 #define ST7735_PWCTR3 0xC2 00046 #define ST7735_PWCTR4 0xC3 00047 #define ST7735_PWCTR5 0xC4 00048 #define ST7735_VMCTR1 0xC5 00049 00050 #define ST7735_RDID1 0xDA 00051 #define ST7735_RDID2 0xDB 00052 #define ST7735_RDID3 0xDC 00053 #define ST7735_RDID4 0xDD 00054 00055 #define ST7735_PWCTR6 0xFC 00056 00057 #define ST7735_GMCTRP1 0xE0 00058 #define ST7735_GMCTRN1 0xE1 00059 00060 // some RGB color definitions RED GREEN BLUE 00061 00062 #define Black 0x0000 // 0, 0, 0 00063 #define Navy 0x8000 // 0, 0, 128 00064 #define DarkGreen 0x0400 // 0, 128, 0 00065 #define DarkCyan 0x8400 // 0, 128, 128 00066 #define Maroon 0x0010 // 128, 0, 0 00067 #define Purple 0x8010 // 128, 0, 128 00068 #define Olive 0x0410 // 128, 128, 0 00069 #define LightGrey 0xC618 // 192, 192, 192 00070 #define DarkGrey 0x7BEF // 128, 128, 128 00071 #define Red 0x001F // 255, 0, 0 00072 #define Green 0x07E0 // 0, 255, 0 00073 #define Yellow 0x07FF // 255, 255, 0 00074 #define Blue 0xF800 // 0, 0, 255 00075 #define Magenta 0xF81F // 255, 0, 255 00076 #define Cyan 0xFFE0 // 0, 255, 255 00077 #define White 0xFFFF // 255, 255, 255 00078 #define Orange 0x053F // 255, 165, 0 00079 #define GreenYellow 0x2FF5 // 173, 255, 47 00080 00081 /* Use this to Calculate and define any colour 00082 00083 #define RGB565CONVERT(red, green, blue) (uint16_t)( (( blue >> 3 ) << 11 ) | (( green >> 2 ) << 5 ) | ( red >> 3 )) 00084 lcd.printf("%4X ",RGB565CONVERT(red value 0-255,green value 0-255,blue value 0-255)); 00085 */ 00086 00087 class ST7735_TFT : public GraphicsDisplay { 00088 public: 00089 00090 /** Create a ST7735_TFT object connected to SPI and three pins. ST7735 requires rs pin to toggle between data/command 00091 * 00092 * @param mosi(SDA),miso(NC),sclk(SCK) SPI 00093 * @param cs pin connected to CS of display (called SS for 'Slave Select' in ST7735 datasheet) 00094 * @param rs pin connected to RS (A0)of display (called D/CX in ST7735 datasheet) 00095 * @param reset pin connected to RESET of display 00096 * 00097 */ 00098 ST7735_TFT(PinName mosi, PinName miso, PinName sclk, PinName cs, PinName rs, PinName reset,const char* name ="TFT"); 00099 00100 /** Get the width of the screen in pixel 00101 * 00102 * @param 00103 * @returns width of screen in pixel 00104 * 00105 */ 00106 virtual int width(); 00107 00108 /** Get the height of the screen in pixel 00109 * 00110 * @returns height of screen in pixel 00111 * 00112 */ 00113 virtual int height(); 00114 00115 /** Draw a pixel at x,y with color 00116 * 00117 * @param x horizontal position 00118 * @param y vertical position 00119 * @param color 16 bit pixel color 00120 */ 00121 virtual void pixel(int x, int y, int colour); 00122 00123 /** Get colour of pixel at x,y 00124 * 00125 * @param x horizontal position 00126 * @param y vertical position 00127 */ 00128 00129 int getpixel(unsigned int x, unsigned int y); 00130 00131 /** draw a circle 00132 * 00133 * @param x0,y0 center 00134 * @param r radius 00135 * @param color 16 bit color * 00136 * 00137 */ 00138 00139 void circle(int x, int y, int r, int colour); 00140 00141 /** draw a filled circle 00142 * 00143 * @param x0,y0 center 00144 * @param r radius 00145 * @param color 16 bit color * 00146 * 00147 * use circle with different radius, 00148 * can miss some pixel 00149 */ 00150 void fillcircle(int x, int y, int r, int colour); 00151 00152 /** draw a 1 pixel line 00153 * 00154 * @param x0,y0 start point 00155 * @param x1,y1 stop point 00156 * @param color 16 bit color 00157 * 00158 */ 00159 void line(int x0, int y0, int x1, int y1, int colour); 00160 00161 /** draw a rect 00162 * 00163 * @param x0,y0 top left corner 00164 * @param x1,y1 down right corner 00165 * @param color 16 bit color 00166 * * 00167 */ 00168 void rect(int x0, int y0, int x1, int y1, int colour); 00169 00170 /** draw a filled rect 00171 * 00172 * @param x0,y0 top left corner 00173 * @param x1,y1 down right corner 00174 * @param color 16 bit color 00175 * 00176 */ 00177 void fillrect(int x0, int y0, int x1, int y1, int colour); 00178 00179 /** setup cursor position 00180 * 00181 * @param x x-position (top left) 00182 * @param y y-position 00183 */ 00184 virtual void locate(int x, int y); 00185 00186 /** Fill the screen with _backgroun color 00187 * 00188 */ 00189 virtual void cls (void); 00190 00191 00192 /** calculate the max number of char in a line 00193 * 00194 * @returns max columns 00195 * depends on actual font size 00196 * 00197 */ 00198 virtual int columns(void); 00199 00200 /** calculate the max number of columns 00201 * 00202 * @returns max column 00203 * depends on actual font size 00204 * 00205 */ 00206 virtual int rows(void); 00207 00208 /** put a char on the screen 00209 * 00210 * @param value char to print 00211 * @returns printed char 00212 * 00213 */ 00214 virtual int _putc(int value); 00215 00216 /** draw a character on given position out of the active font to the TFT 00217 * 00218 * @param x x-position of char (top left) 00219 * @param y y-position 00220 * @param c char to print 00221 * 00222 */ 00223 virtual void character(int x, int y, int c); 00224 00225 /** paint a bitmap on the TFT 00226 * 00227 * @param x,y : upper left corner 00228 * @param w width of bitmap 00229 * @param h high of bitmap 00230 * @param *bitmap pointer to the bitmap data 00231 * 00232 * bitmap format: 16 bit R5 G6 B5 00233 * 00234 * use Gimp to create / load , save as BMP, option 16 bit R5 G6 B5 00235 * use winhex to load this file and mark data stating at offset 0x46 to end 00236 * use edit -> copy block -> C Source to export C array 00237 * paste this array into your program 00238 * 00239 * define the array as static const unsigned char to put it into flash memory 00240 * cast the pointer to (unsigned char *) : 00241 * tft.Bitmap(10,40,309,50,(unsigned char *)scala); 00242 */ 00243 void Bitmap(unsigned int x, unsigned int y, unsigned int w, unsigned int h,unsigned char *bitmap); 00244 00245 00246 /** paint a 16 bit BMP from local filesytem on the TFT (slow) 00247 * 00248 * @param x,y : upper left corner 00249 * @param *Name_BMP name of the BMP file 00250 * @returns 1 if bmp file was found and painted 00251 * @returns -1 if bmp file was found not found 00252 * @returns -2 if bmp file is not 16bit 00253 * @returns -3 if bmp file is to big for screen 00254 * @returns -4 if buffer malloc go wrong 00255 * 00256 * bitmap format: 16 bit R5 G6 B5 00257 * 00258 * use Gimp to create / load , save as BMP, option 16 bit R5 G6 B5 00259 * copy to internal file system 00260 * 00261 */ 00262 00263 int BMP_16(unsigned int x, unsigned int y, const char *Name_BMP); 00264 00265 /** Read an area from the LCD RAM to MCU RAM 00266 * 00267 * @param x,y : upper left corner 00268 * @param w width of bitmap 00269 * @param h high of bitmap 00270 * @param *buffer pointer to the buffer 00271 */ 00272 00273 void read_area(unsigned int x, unsigned int y, unsigned int w, unsigned int h,unsigned char *buffer); 00274 00275 /** select the font to use 00276 * 00277 * @param f pointer to font array 00278 * 00279 * font array can created with GLCD Font Creator from http://www.mikroe.com 00280 * you have to add 4 parameter at the beginning of the font array to use: 00281 * - the number of byte / char 00282 * - the vertial size in pixel 00283 * - the horizontal size in pixel 00284 * - the number of byte per vertical line 00285 * you also have to change the array to char[] 00286 * 00287 */ 00288 void set_font(unsigned char* f); 00289 00290 /** Set the orientation of the screen 00291 * x,y: 0,0 is always top left 00292 * 00293 * @param o direction to use the screen (0-3) 90� Steps 00294 * 00295 */ 00296 void set_orientation(unsigned int o); 00297 00298 //SPI _spi; 00299 BurstSPI _spi; 00300 DigitalOut _cs; 00301 DigitalOut _rs; 00302 DigitalOut _reset; 00303 unsigned char* font; 00304 00305 //protected: 00306 00307 /** draw a horizontal line 00308 * 00309 * @param x0 horizontal start 00310 * @param x1 horizontal stop 00311 * @param y vertical position 00312 * @param color 16 bit color 00313 * 00314 */ 00315 void hline(int x0, int x1, int y, int colour); 00316 00317 /** draw a vertical line 00318 * 00319 * @param x horizontal position 00320 * @param y0 vertical start 00321 * @param y1 vertical stop 00322 * @param color 16 bit color 00323 */ 00324 void vline(int y0, int y1, int x, int colour); 00325 00326 /** Set draw window region 00327 * 00328 * @param x horizontal position 00329 * @param y vertical position 00330 * @param w window width in pixel 00331 * @param h window height in pixels 00332 */ 00333 protected: 00334 void window (unsigned int x, unsigned int y, unsigned int w, unsigned int h); 00335 00336 /** Set draw window region to whole screen 00337 * 00338 */ 00339 void WindowMax (void); 00340 00341 /** Init the ST7735 controller 00342 * 00343 */ 00344 00345 void tft_reset(); 00346 00347 /** Write data to the LCD controller 00348 * 00349 * @param dat data written to LCD controller 00350 * 00351 */ 00352 void wr_dat(int value); 00353 00354 /** Write a command the LCD controller 00355 * 00356 * @param cmd: command to be written 00357 * 00358 */ 00359 void wr_cmd(int value); 00360 00361 /** Start data sequence to the LCD controller 00362 * 00363 */ 00364 void wr_dat_start(); 00365 00366 /** Stop of data writing to the LCD controller 00367 * 00368 */ 00369 void wr_dat_stop(); 00370 00371 /** write data to the LCD controller 00372 * 00373 * @param data to be written 00374 * * 00375 */ 00376 void wr_dat_only(unsigned short dat); 00377 00378 /** Read data from the LCD controller 00379 * 00380 * @returns data from LCD controller 00381 * 00382 */ 00383 unsigned short rd_dat(void); 00384 00385 /** Write a value to the to a LCD register 00386 * 00387 * @param reg register to be written 00388 * @param val data to be written 00389 */ 00390 void wr_reg (unsigned char reg, unsigned short val); 00391 00392 /** Read a LCD register 00393 * 00394 * @param reg register to be read 00395 * @returns value of the register 00396 */ 00397 unsigned short rd_reg (unsigned char reg); 00398 00399 unsigned int orientation; 00400 unsigned int char_x; 00401 unsigned int char_y; 00402 }; 00403 #endif
Generated on Tue Jul 12 2022 23:14:00 by
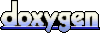