
Added for gyro and testing
Embed:
(wiki syntax)
Show/hide line numbers
MPU6050.h
00001 /*Use #define MPU6050_ES before you include this file if you have an engineering sample (older EVBs will have them), to find out if you have one, check your accelerometer output. 00002 If it is half of what you expected, and you still are on the correct planet, you got an engineering sample 00003 */ 00004 00005 00006 #ifndef MPU6050_H 00007 #define MPU6050_H 00008 00009 /** 00010 * Includes 00011 */ 00012 #include "mbed.h" 00013 00014 00015 /** 00016 * Defines 00017 */ 00018 #ifndef MPU6050_ADDRESS 00019 #define MPU6050_ADDRESS 0x68 // address pin low (GND), default for InvenSense evaluation board 00020 #endif 00021 00022 #ifdef MPU6050_ES 00023 #define DOUBLE_ACCELERO 00024 #endif 00025 00026 /** 00027 * Registers 00028 */ 00029 #define MPU6050_CONFIG_REG 0x1A 00030 #define MPU6050_GYRO_CONFIG_REG 0x1B 00031 #define MPU6050_ACCELERO_CONFIG_REG 0x1C 00032 00033 #define MPU6050_INT_PIN_CFG 0x37 00034 00035 #define MPU6050_ACCEL_XOUT_H_REG 0x3B 00036 #define MPU6050_ACCEL_YOUT_H_REG 0x3D 00037 #define MPU6050_ACCEL_ZOUT_H_REG 0x3F 00038 00039 #define MPU6050_TEMP_H_REG 0x41 00040 00041 #define MPU6050_GYRO_XOUT_H_REG 0x43 00042 #define MPU6050_GYRO_YOUT_H_REG 0x45 00043 #define MPU6050_GYRO_ZOUT_H_REG 0x47 00044 00045 00046 00047 #define MPU6050_PWR_MGMT_1_REG 0x6B 00048 #define MPU6050_WHO_AM_I_REG 0x75 00049 00050 00051 00052 /** 00053 * Definitions 00054 */ 00055 #define MPU6050_SLP_BIT 6 00056 #define MPU6050_BYPASS_BIT 1 00057 00058 #define MPU6050_BW_256 0 00059 #define MPU6050_BW_188 1 00060 #define MPU6050_BW_98 2 00061 #define MPU6050_BW_42 3 00062 #define MPU6050_BW_20 4 00063 #define MPU6050_BW_10 5 00064 #define MPU6050_BW_5 6 00065 00066 #define MPU6050_ACCELERO_RANGE_2G 0 00067 #define MPU6050_ACCELERO_RANGE_4G 1 00068 #define MPU6050_ACCELERO_RANGE_8G 2 00069 #define MPU6050_ACCELERO_RANGE_16G 3 00070 00071 #define MPU6050_GYRO_RANGE_250 0 00072 #define MPU6050_GYRO_RANGE_500 1 00073 #define MPU6050_GYRO_RANGE_1000 2 00074 #define MPU6050_GYRO_RANGE_2000 3 00075 00076 //interrupt address 00077 #define MPU6050_RA_INT_ENABLE 0x38 00078 //define how the accelerometer is placed on surface 00079 #define X_AXIS 1 00080 #define Y_AXIS 2 00081 #define Z_AXIS 0 00082 //translation from radians to degrees 00083 #define RADIANS_TO_DEGREES 180/3.1415926536 00084 //constant used for the complementary filter, which ranges usually from 0.9 to 1.0 00085 #define ALPHA 0.96 //filter constant 00086 //scale the gyro 00087 #define GYRO_SCALE 2.7176 00088 00089 /** MPU6050 IMU library. 00090 * 00091 * Example: 00092 * @code 00093 * Later, maybe 00094 * @endcode 00095 */ 00096 class MPU6050 { 00097 public: 00098 /** 00099 * Constructor. 00100 * 00101 * Sleep mode of MPU6050 is immediatly disabled 00102 * 00103 * @param sda - mbed pin to use for the SDA I2C line. 00104 * @param scl - mbed pin to use for the SCL I2C line. 00105 */ 00106 MPU6050(PinName sda, PinName scl); 00107 00108 00109 /** 00110 * Tests the I2C connection by reading the WHO_AM_I register. 00111 * 00112 * @return True for a working connection, false for an error 00113 */ 00114 bool testConnection( void ); 00115 00116 /** 00117 * Sets the bandwidth of the digital low-pass filter 00118 * 00119 * Macros: MPU6050_BW_256 - MPU6050_BW_188 - MPU6050_BW_98 - MPU6050_BW_42 - MPU6050_BW_20 - MPU6050_BW_10 - MPU6050_BW_5 00120 * Last number is the gyro's BW in Hz (accelero BW is virtually identical) 00121 * 00122 * @param BW - The three bits that set the bandwidth (use the predefined macros) 00123 */ 00124 void setBW( char BW ); 00125 00126 /** 00127 * Sets the auxiliary I2C bus in bypass mode to read the sensors behind the MPU6050 (useful for eval board, otherwise just connect them to primary I2C bus) 00128 * 00129 * @param state - Enables/disables the I2C bypass mode 00130 */ 00131 void setI2CBypass ( bool state ); 00132 00133 /** 00134 * Sets the Accelero full-scale range 00135 * 00136 * Macros: MPU6050_ACCELERO_RANGE_2G - MPU6050_ACCELERO_RANGE_4G - MPU6050_ACCELERO_RANGE_8G - MPU6050_ACCELERO_RANGE_16G 00137 * 00138 * @param range - The two bits that set the full-scale range (use the predefined macros) 00139 */ 00140 void setAcceleroRange(char range); 00141 00142 /** 00143 * Reads the accelero x-axis. 00144 * 00145 * @return 16-bit signed integer x-axis accelero data 00146 */ 00147 int getAcceleroRawX( void ); 00148 00149 /** 00150 * Reads the accelero y-axis. 00151 * 00152 * @return 16-bit signed integer y-axis accelero data 00153 */ 00154 int getAcceleroRawY( void ); 00155 00156 /** 00157 * Reads the accelero z-axis. 00158 * 00159 * @return 16-bit signed integer z-axis accelero data 00160 */ 00161 int getAcceleroRawZ( void ); 00162 00163 /** 00164 * Reads all accelero data. 00165 * 00166 * @param data - pointer to signed integer array with length three: data[0] = X, data[1] = Y, data[2] = Z 00167 */ 00168 void getAcceleroRaw( int *data ); 00169 00170 /** 00171 * Reads all accelero data, gives the acceleration in m/s2 00172 * 00173 * Function uses the last setup value of the full scale range, if you manually set in another range, this won't work. 00174 * 00175 * @param data - pointer to float array with length three: data[0] = X, data[1] = Y, data[2] = Z 00176 */ 00177 void getAccelero( float *data ); 00178 00179 /** 00180 * Sets the Gyro full-scale range 00181 * 00182 * Macros: MPU6050_GYRO_RANGE_250 - MPU6050_GYRO_RANGE_500 - MPU6050_GYRO_RANGE_1000 - MPU6050_GYRO_RANGE_2000 00183 * 00184 * @param range - The two bits that set the full-scale range (use the predefined macros) 00185 */ 00186 void setGyroRange(char range); 00187 00188 /** 00189 * Reads the gyro x-axis. 00190 * 00191 * @return 16-bit signed integer x-axis gyro data 00192 */ 00193 int getGyroRawX( void ); 00194 00195 /** 00196 * Reads the gyro y-axis. 00197 * 00198 * @return 16-bit signed integer y-axis gyro data 00199 */ 00200 int getGyroRawY( void ); 00201 00202 /** 00203 * Reads the gyro z-axis. 00204 * 00205 * @return 16-bit signed integer z-axis gyro data 00206 */ 00207 int getGyroRawZ( void ); 00208 00209 /** 00210 * Reads all gyro data. 00211 * 00212 * @param data - pointer to signed integer array with length three: data[0] = X, data[1] = Y, data[2] = Z 00213 */ 00214 void getGyroRaw( int *data ); 00215 00216 /** 00217 * Reads all gyro data, gives the gyro in rad/s 00218 * 00219 * Function uses the last setup value of the full scale range, if you manually set in another range, this won't work. 00220 * 00221 * @param data - pointer to float array with length three: data[0] = X, data[1] = Y, data[2] = Z 00222 */ 00223 void getGyro( float *data); 00224 00225 /** 00226 * Reads temperature data. 00227 * 00228 * @return 16 bit signed integer with the raw temperature register value 00229 */ 00230 int getTempRaw( void ); 00231 00232 /** 00233 * Returns current temperature 00234 * 00235 * @returns float with the current temperature 00236 */ 00237 float getTemp( void ); 00238 00239 /** 00240 * Sets the sleep mode of the MPU6050 00241 * 00242 * @param state - true for sleeping, false for wake up 00243 */ 00244 void setSleepMode( bool state ); 00245 00246 00247 /** 00248 * Writes data to the device, could be private, but public is handy so you can transmit directly to the MPU. 00249 * 00250 * @param adress - register address to write to 00251 * @param data - data to write 00252 */ 00253 void write( char address, char data); 00254 00255 /** 00256 * Read data from the device, could be private, but public is handy so you can transmit directly to the MPU. 00257 * 00258 * @param adress - register address to write to 00259 * @return - data from the register specified by RA 00260 */ 00261 char read( char adress); 00262 00263 /** 00264 * Read multtiple regigsters from the device, more efficient than using multiple normal reads. 00265 * 00266 * @param adress - register address to write to 00267 * @param length - number of bytes to read 00268 * @param data - pointer where the data needs to be written to 00269 */ 00270 void read( char adress, char *data, int length); 00271 00272 /** 00273 * function for calculating the angle from the accelerometer. 00274 * it takes 3 values which correspond acceleration in X, Y and Z direction and calculates angles in degrees 00275 * for pitch, roll and one more direction.. (NOT YAW!) 00276 * 00277 * @param data - angle calculated using only accelerometer 00278 * 00279 */ 00280 void getAcceleroAngle( float *data ); 00281 00282 00283 /**function which allows to produce the offset values for gyro and accelerometer. 00284 * offset for gyro is simply a value, which needs to be substracted from original gyro rad/sec speed 00285 * but offset for accelerometer is calculated in angles... later on might change that 00286 * function simply takes the number of samples to be taken and calculated the average 00287 * 00288 * @param accOffset - accelerometer offset in angle 00289 * @param gyroOffset - gyroscope offset in rad/s 00290 * @param sampleSize - number of samples to be taken for calculating offsets 00291 * 00292 */ 00293 void getOffset(float *accOffset, float *gyroOffset, int sampleSize); 00294 00295 /** 00296 * function for computing the angle, when accelerometer angle offset and gyro offset are both known. 00297 * we also need to know how much time passed from previous calculation to now 00298 * it produces the angle in degrees. However angles are produced from -90.0 to 90.0 degrees 00299 * if anyone need smth different, they can update this library... 00300 * 00301 * @param angle - calculated accurate angle from complemetary filter 00302 * @param accOffset - offset in angle for the accelerometer 00303 * @param gyroOffset - offset in rad/s for the gyroscope 00304 * @param interval - time before previous angle calculation and now 00305 * 00306 */ 00307 void computeAngle (float *angle, float *accOffset, float *gyroOffset, float interval); 00308 00309 ///function, which enables interrupts on MPU6050 INT pin 00310 void enableInt( void ); 00311 00312 ///disables interrupts 00313 void disableInt( void ); 00314 00315 /**function which sets the alpha value - constant for the complementary filter. default alpha = 0.97 00316 * 00317 * @param val - value the alpha (complementary filter constant) should be set to 00318 * 00319 */ 00320 void setAlpha(float val); 00321 00322 private: 00323 00324 I2C connection; 00325 char currentAcceleroRange; 00326 char currentGyroRange; 00327 float alpha; 00328 00329 }; 00330 00331 00332 00333 #endif
Generated on Sat Jul 16 2022 12:46:53 by
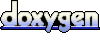