
Added for gyro and testing
Embed:
(wiki syntax)
Show/hide line numbers
IMUWheelchair.h
00001 #ifndef IMUWheelchair_H 00002 #define IMUWheelchair_H 00003 00004 #include "filter.h" 00005 //#include "mbed.h" 00006 #include "math.h" 00007 #include <MPU6050.h> 00008 00009 #define PI 3.141593 00010 00011 /*#define SDA D14 00012 #define SCL D15*/ 00013 #define SDA PB_9 00014 #define SCL PB_8 00015 #define SAMPLEFREQ 50 00016 #define CAL_TIME 3 00017 00018 class IMUWheelchair { 00019 public: 00020 //The constructor for this class 00021 IMUWheelchair(Serial* out, Timer* time); 00022 IMUWheelchair(PinName sda_pin, PinName scl_pin, Serial* out, Timer* time); 00023 00024 //Set up the IMU, check if it connects 00025 void setup(); 00026 00027 //Get the x-component of the angular acceleration 00028 double accel_x(); 00029 00030 //Get the y-component of the angular acceleration 00031 double accel_y(); 00032 00033 //Get the z-component of the angular acceleration 00034 double accel_z(); 00035 00036 //Get the x-component of gyro, angular velocity 00037 double gyro_x(); 00038 00039 //Get the y-component of gyro, angular velocity 00040 double gyro_y(); 00041 00042 //Get the z-component of gyro, angular velocity 00043 double gyro_z(); 00044 00045 //Magnometer to find angle relative to North to compare to gyroscope 00046 //double angle_north(); 00047 00048 //Get the YAW, or angle (theta), direction facing 00049 double yaw(); 00050 00051 //Get the pitch, (Up and down component) 00052 double pitch(); 00053 00054 //Get the roll, the tilt 00055 double roll(); 00056 00057 MPU6050* imu; //The IMU we're testing from, MPU6050 00058 00059 private: 00060 Serial* usb; //the connection port 00061 Timer* t;//to calculate the time 00062 float accelData[3]; // stores the angular acceleration component 00063 float gyroData[3]; //stores the gyro data x,y,z 00064 float* accelD; //Pointer that points to either accelData 00065 float* gyroD; //Ptr to the gyroData array 00066 00067 float angleData[3]; //Contains the pitch, roll, yaw angle 00068 float* angleD;//Ptr to angleData array 00069 00070 void calibrate_yaw(); 00071 00072 bool start; 00073 00074 }; 00075 00076 #endif
Generated on Sat Jul 16 2022 12:46:53 by
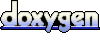