
Dependencies: NetServices mbed
main.cpp
00001 #include "mbed.h" 00002 #include "EthernetNetIf.h" 00003 #include "NTPClient.h" 00004 #include "SMTPClient.h" 00005 00006 #define HOSTNAME "mbedSE" 00007 00008 // server is domain name eg. mail.authsmtp.com 00009 // port is smtp port eg. 25 or 23 00010 // domain must be acceptable to server (but often is not strictly enforced since does not affect FROM address) 00011 #define SERVER "server" 00012 #define PORT 25 00013 #define USER "username" 00014 #define PASSWORD "password" 00015 #define DOMAIN "mailnull.com" 00016 #define FROM_ADDRESS "segundo.smtpclient@mailnull.com" 00017 #define TO_ADDRESS "segundo.smtpclient@mailnull.com" 00018 00019 EthernetNetIf eth(HOSTNAME); 00020 DigitalOut led1(LED1, "led1"); 00021 00022 int main() { 00023 00024 printf("\n\n/* Segundo Equipo SMTPClient library demonstration */\n"); 00025 printf("Make sure you have modified the SERVER, PORT etc in the source code\n"); 00026 00027 EthernetErr ethErr; 00028 int count = 0; 00029 do { 00030 printf("Setting up %d...\n", ++count); 00031 ethErr = eth.setup(); 00032 if (ethErr) printf("Timeout\n", ethErr); 00033 } while (ethErr != ETH_OK); 00034 00035 printf("Connected OK\n"); 00036 const char* hwAddr = eth.getHwAddr(); 00037 printf("HW address : %02x:%02x:%02x:%02x:%02x:%02x\n", 00038 hwAddr[0], hwAddr[1], hwAddr[2], 00039 hwAddr[3], hwAddr[4], hwAddr[5]); 00040 00041 IpAddr ethIp = eth.getIp(); 00042 printf("IP address : %d.%d.%d.%d\n", ethIp[0], ethIp[1], ethIp[2], ethIp[3]); 00043 00044 NTPClient ntp; 00045 printf("NTP setTime...\n"); 00046 Host server(IpAddr(), 123, "pool.ntp.org"); 00047 printf("Result : %d\n", ntp.setTime(server)); 00048 00049 time_t ctTime = time(NULL); 00050 printf("\nTime is now (UTC): %d %s\n", ctTime, ctime(&ctTime)); 00051 00052 Host host(IpAddr(), PORT, SERVER); 00053 SMTPClient smtp(host, DOMAIN, USER, PASSWORD, SMTP_AUTH_PLAIN); 00054 00055 EmailMessage msg; 00056 msg.setFrom(FROM_ADDRESS); 00057 msg.addTo(TO_ADDRESS); 00058 msg.printf("Subject: mbed SMTPClient test at %s", ctime(&ctTime)); 00059 msg.printf("1234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890\n"); 00060 msg.printf(".\r\n"); 00061 msg.printf("1234567890123456789012345678901234567890123456789012345678901234567890123456789012345678901234567890\n"); 00062 00063 printf("Send result %d\n", smtp.send(&msg)); 00064 printf("Last response | %s", smtp.getLastResponse().c_str()); 00065 00066 Timer tm; 00067 tm.start(); 00068 00069 while (true) { 00070 if (tm.read() > 0.5) { 00071 led1 = !led1; 00072 tm.start(); 00073 } 00074 } 00075 }
Generated on Thu Jul 14 2022 02:13:35 by
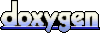