Grove - Serial LCD
Embed:
(wiki syntax)
Show/hide line numbers
SerialLCD.h
00001 /* 00002 SerialLCD.h - Serial LCD driver Library 00003 00004 2010-2013 Copyright (c) Seeed Technology Inc (www.seeedstudio.com) 00005 Authors: Jimbo.We, Visweswara R and Frankie.Chu (Orignially written for Seeeduino) 00006 00007 This library can be used under Apache License 2.0 or MIT License. 00008 */ 00009 00010 #ifndef __SERIAL_LCD_H__ 00011 #define __SERIAL_LCD_H__ 00012 00013 #include "mbed.h" 00014 00015 /** Grove Serial LCD libray. The Serial LCD is connected to mbed through a UART port. 00016 */ 00017 00018 class SerialLCD : public Serial { 00019 public: 00020 00021 SerialLCD(PinName, PinName); 00022 void begin(); 00023 void clear(); 00024 void home(); 00025 00026 void noDisplay(); 00027 void display(); 00028 void noBlink(); 00029 void blink(); 00030 void noCursor(); 00031 void cursor(); 00032 void scrollDisplayLeft(); 00033 void scrollDisplayRight(); 00034 void leftToRight(); 00035 void rightToLeft(); 00036 void autoscroll(); 00037 void noAutoscroll(); 00038 00039 void setCursor(uint8_t, uint8_t); 00040 void noPower(void); 00041 void Power(void); 00042 void noBacklight(void); 00043 void backlight(void); 00044 void print(uint8_t b); 00045 void print(const char[]); 00046 00047 }; 00048 00049 #endif
Generated on Tue Jul 12 2022 23:34:42 by
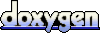