Grove - Serial LCD
Embed:
(wiki syntax)
Show/hide line numbers
SerialLCD.cpp
00001 /* 00002 SerialLCD.h - Serial LCD driver Library 00003 00004 2010-2013 Copyright (c) Seeed Technology Inc (www.seeedstudio.com) 00005 Authors: Jimbo.We, Visweswara R and Frankie.Chu (Orignially written for Seeeduino) 00006 00007 This library can be used under Apache License 2.0 or MIT License. 00008 */ 00009 00010 #include "SerialLCD.h" 00011 00012 //Initialization Commands or Responses 00013 00014 #define SLCD_INIT 0xA3 00015 #define SLCD_INIT_ACK 0xA5 00016 #define SLCD_INIT_DONE 0xAA 00017 00018 //WorkingMode Commands or Responses 00019 #define SLCD_CONTROL_HEADER 0x9F 00020 #define SLCD_CHAR_HEADER 0xFE 00021 #define SLCD_CURSOR_HEADER 0xFF 00022 #define SLCD_CURSOR_ACK 0x5A 00023 00024 #define SLCD_RETURN_HOME 0x61 00025 #define SLCD_DISPLAY_OFF 0x63 00026 #define SLCD_DISPLAY_ON 0x64 00027 #define SLCD_CLEAR_DISPLAY 0x65 00028 #define SLCD_CURSOR_OFF 0x66 00029 #define SLCD_CURSOR_ON 0x67 00030 #define SLCD_BLINK_OFF 0x68 00031 #define SLCD_BLINK_ON 0x69 00032 #define SLCD_SCROLL_LEFT 0x6C 00033 #define SLCD_SCROLL_RIGHT 0x72 00034 #define SLCD_NO_AUTO_SCROLL 0x6A 00035 #define SLCD_AUTO_SCROLL 0x6D 00036 #define SLCD_LEFT_TO_RIGHT 0x70 00037 #define SLCD_RIGHT_TO_LEFT 0x71 00038 #define SLCD_POWER_ON 0x83 00039 #define SLCD_POWER_OFF 0x82 00040 #define SLCD_INVALIDCOMMAND 0x46 00041 #define SLCD_BACKLIGHT_ON 0x81 00042 #define SLCD_BACKLIGHT_OFF 0x80 00043 00044 SerialLCD::SerialLCD(PinName rx, PinName tx) : Serial(rx,tx) 00045 { 00046 Serial::baud(9600); 00047 } 00048 00049 /********** High level commands, for the user! **********/ 00050 00051 /// Initialize the Serial LCD Driver. 00052 void SerialLCD::begin() 00053 { 00054 wait_ms(2); 00055 Serial::putc(SLCD_CONTROL_HEADER); 00056 Serial::putc(SLCD_POWER_OFF); 00057 wait_ms(1); 00058 Serial::putc(SLCD_CONTROL_HEADER); 00059 Serial::putc(SLCD_POWER_ON); 00060 wait_ms(1); 00061 Serial::putc(SLCD_INIT_ACK); 00062 while(1) { 00063 if (Serial::readable() > 0 &&Serial::getc()==SLCD_INIT_DONE) 00064 break; 00065 } 00066 wait_ms(2); 00067 } 00068 /// Clear the display 00069 void SerialLCD::clear() 00070 { 00071 Serial::putc(SLCD_CONTROL_HEADER); 00072 Serial::putc(SLCD_CLEAR_DISPLAY); 00073 } 00074 /// Return to home(top-left corner of LCD) 00075 void SerialLCD::home() 00076 { 00077 Serial::putc(SLCD_CONTROL_HEADER); 00078 Serial::putc(SLCD_RETURN_HOME); 00079 wait_ms(2); //this command needs more time; 00080 } 00081 /// Set Cursor to (Column,Row) Position 00082 void SerialLCD::setCursor(uint8_t column, uint8_t row) 00083 { 00084 Serial::putc(SLCD_CONTROL_HEADER); 00085 Serial::putc(SLCD_CURSOR_HEADER); //cursor header command 00086 Serial::putc(column); 00087 Serial::putc(row); 00088 } 00089 /// Switch the display off without clearing RAM 00090 void SerialLCD::noDisplay() 00091 { 00092 Serial::putc(SLCD_CONTROL_HEADER); 00093 Serial::putc(SLCD_DISPLAY_OFF); 00094 } 00095 /// Switch the display on 00096 void SerialLCD::display() 00097 { 00098 Serial::putc(SLCD_CONTROL_HEADER); 00099 Serial::putc(SLCD_DISPLAY_ON); 00100 } 00101 /// Switch the underline cursor off 00102 void SerialLCD::noCursor() 00103 { 00104 Serial::putc(SLCD_CONTROL_HEADER); 00105 Serial::putc(SLCD_CURSOR_OFF); 00106 } 00107 /// Switch the underline cursor on 00108 void SerialLCD::cursor() 00109 { 00110 Serial::putc(SLCD_CONTROL_HEADER); 00111 Serial::putc(SLCD_CURSOR_ON); 00112 } 00113 00114 /// Switch off the blinking cursor 00115 void SerialLCD::noBlink() 00116 { 00117 Serial::putc(SLCD_CONTROL_HEADER); 00118 Serial::putc(SLCD_BLINK_OFF); 00119 } 00120 /// Switch on the blinking cursor 00121 void SerialLCD::blink() 00122 { 00123 Serial::putc(SLCD_CONTROL_HEADER); 00124 Serial::putc(SLCD_BLINK_ON); 00125 } 00126 /// Scroll the display left without changing the RAM 00127 void SerialLCD::scrollDisplayLeft(void) 00128 { 00129 Serial::putc(SLCD_CONTROL_HEADER); 00130 Serial::putc(SLCD_SCROLL_LEFT); 00131 } 00132 /// Scroll the display right without changing the RAM 00133 void SerialLCD::scrollDisplayRight(void) 00134 { 00135 Serial::putc(SLCD_CONTROL_HEADER); 00136 Serial::putc(SLCD_SCROLL_RIGHT); 00137 } 00138 /// Set the text flow "Left to Right" 00139 void SerialLCD::leftToRight(void) 00140 { 00141 Serial::putc(SLCD_CONTROL_HEADER); 00142 Serial::putc(SLCD_LEFT_TO_RIGHT); 00143 } 00144 /// Set the text flow "Right to Left" 00145 void SerialLCD::rightToLeft(void) 00146 { 00147 Serial::putc(SLCD_CONTROL_HEADER); 00148 Serial::putc(SLCD_RIGHT_TO_LEFT); 00149 } 00150 /// This will 'right justify' text from the cursor 00151 void SerialLCD::autoscroll(void) 00152 { 00153 Serial::putc(SLCD_CONTROL_HEADER); 00154 Serial::putc(SLCD_AUTO_SCROLL); 00155 } 00156 /// This will 'left justify' text from the cursor 00157 void SerialLCD::noAutoscroll(void) 00158 { 00159 Serial::putc(SLCD_CONTROL_HEADER); 00160 Serial::putc(SLCD_NO_AUTO_SCROLL); 00161 } 00162 /// Switch on the LCD power 00163 void SerialLCD::Power(void) 00164 { 00165 Serial::putc(SLCD_CONTROL_HEADER); 00166 Serial::putc(SLCD_POWER_ON); 00167 } 00168 /// Switch off the LCD power 00169 void SerialLCD::noPower(void) 00170 { 00171 Serial::putc(SLCD_CONTROL_HEADER); 00172 Serial::putc(SLCD_POWER_OFF); 00173 } 00174 /// Switch off the back light 00175 void SerialLCD::noBacklight(void) 00176 { 00177 Serial::putc(SLCD_CONTROL_HEADER); 00178 Serial::putc(SLCD_BACKLIGHT_OFF); 00179 } 00180 /// Switch on the back light 00181 void SerialLCD::backlight(void) 00182 { 00183 Serial::putc(SLCD_CONTROL_HEADER); 00184 Serial::putc(SLCD_BACKLIGHT_ON); 00185 } 00186 /// Print char 00187 void SerialLCD::print(uint8_t b) 00188 { 00189 Serial::putc(SLCD_CHAR_HEADER); 00190 Serial::putc(b); 00191 } 00192 /// Print char 00193 void SerialLCD::print(const char b[]) 00194 { 00195 Serial::putc(SLCD_CHAR_HEADER); 00196 Serial::puts(b); 00197 }
Generated on Tue Jul 12 2022 23:34:42 by
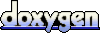