Programme+libraires
Dependents: Serveur_web_pt2 Serveur_web_pt2_V2_avec_index_html Serveur_web_pt2_index_commenter Serveur_web_pt2_index_commenter
Fork of HTTPServer by
MyOwnHandler.h
00001 #ifndef MYOWNHANDLER_H 00002 #define MYOWNHANDLER_H 00003 00004 #include "../HTTPRequestHandler.h" 00005 float x,y; 00006 00007 class MyOwnHandler : public HTTPRequestHandler 00008 { 00009 public: 00010 MyOwnHandler(const char* rootPath, const char* path, TCPSocket* pTCPSocket) 00011 : HTTPRequestHandler(rootPath, path, pTCPSocket) {} 00012 virtual ~MyOwnHandler() {} 00013 00014 //protected: 00015 static inline HTTPRequestHandler* inst(const char* rootPath, 00016 const char* path, TCPSocket* pTCPSocket) 00017 { return new MyOwnHandler(rootPath, path, pTCPSocket); } 00018 00019 virtual void doGet(); 00020 virtual void doPost() {} 00021 virtual void doHead() {} 00022 00023 virtual void onReadable() {} 00024 virtual void onWriteable() { close(); } // Data has been written, 00025 // we can close the connection 00026 virtual void onClose() {} 00027 }; 00028 00029 void MyOwnHandler::doGet() 00030 { 00031 // To get request data, we use the method path() 00032 // We assume that path() will be: 00033 // /variable 00034 // or /variable value 00035 // Get variable string 00036 int endVar = path().find_first_of('%', 1); 00037 string variable = path().substr(1, endVar-1); 00038 // Get value string 00039 string value; 00040 if (endVar!=string::npos) value = path().substr(endVar+3, string::npos); 00041 // Get a pointer on the variable 00042 // The type of the variable must be float 00043 float *ptr = NULL; 00044 if (variable.compare("x")==0) ptr=&x; 00045 else if (variable.compare("y")==0) ptr=&y; 00046 // else if (variable.compare("variable")==0) ptr=&variable; 00047 // Get the float value and set the variable value 00048 if ((ptr!=NULL)&&(!value.empty())) { 00049 float v; 00050 if (sscanf(value.c_str(), "%f", &v)!=EOF) *ptr=v; 00051 } 00052 // Server response will be the value of the variable 00053 char resp[20]; 00054 if (ptr!=NULL) { 00055 sprintf(resp, "%f", *ptr); 00056 } else { 00057 sprintf(resp, "Unknown variable"); 00058 } 00059 setContentLen( strlen(resp) ); 00060 respHeaders()["Connection"] = "close"; 00061 writeData(resp, strlen(resp)); 00062 } 00063 #endif
Generated on Sun Jul 17 2022 17:21:51 by
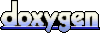