
Basic Read and Writer implementation for an OEM Mifare RWD Device.
RWDMifare Class Reference
Used to interface to a OEM-Mifare RFID card reader/writer. More...
#include <RWDMifare.h>
Inherits RWDModule.
Public Types | |
enum | RWDMifareErr { MIFARE_OK, MIFARE_HW, MIFARE_NOCARD, MIFARE_WRONGKEY, MIFARE_TRAIL } |
Error Codes: An enumerate of the possible error codes generated by the class. More... | |
enum | MifareCMD { CMD_Get_Status = 0x53, CMD_Prog_EEPROM = 0x50, CMD_Read_Block = 0x52, CMD_Write_Block = 0x57, CMD_Store_Key = 0x4b, CMD_Get_UID = 0x55 } |
Commands: An enumerate of the commands supported by this class. More... | |
enum | Ack_Code |
Acknowledge Codes: An enumerate of the masks of status code fields. More... | |
enum | EEPROM_ADDR |
EEPROM Address: Address constants for accessing the EEPROM Addresses. More... | |
enum | POLL_TIME |
Poll Times: Available values of the EEPROM location controlling Poll Time. More... | |
enum | Aux_Data |
Auxilary Data: Values to set the function of OP0 and OP1. More... | |
enum | Mifare_Icode |
Mode: Controls whether the device operates in MIFARE or ICODE mode (NB only Mifare supported, this is included for completion) More... | |
enum | Weigand_Parity |
Weigand Parity: Sets the Weigand Parity if the Weigand option is used NB not implemented. More... | |
enum | OP_Source_Data |
OP Source Sets the data output on OP0. More... | |
enum | Aux_Out_ReDir |
Aux Output Redirection Sets the output pin for OP0 data. More... | |
enum | OP_Fmt |
OP Format Controls the output format of data on OP. More... | |
enum | Byte_Order |
Aux output order Changes the byte order data is sent on the Aux output line. More... | |
Public Member Functions | |
RWDMifareErr | init () |
Initialises the device. | |
RWDMifareErr | getUID (uint8_t *pUID, size_t *pLen) |
Gets UID of card, as well as the length of. | |
RWDMifareErr | getStatus (uint8_t *Status) |
Gets the Reader Status. | |
RWDMifareErr | printStatus (uint8_t Status) |
Prints a description of status. | |
RWDMifareErr | Prog_EEPROM (uint8_t Address, uint8_t Value) |
Programs the EEPROM. | |
RWDMifareErr | StoreKey (uint8_t KeyNumber, uint8_t *Key) |
Stores an access key to the internal EEPROM Memory. | |
RWDMifareErr | ReadBlock (uint8_t Addr, uint8_t KeyNumber_Type, uint8_t *Data) |
Reads a block of data from the card. | |
RWDMifareErr | ReadBlock (uint8_t Addr, uint8_t KeyNumber, uint8_t Type, uint8_t *Data) |
Reads a block of data from the card. | |
RWDMifareErr | WriteBlock (uint8_t Addr, uint8_t KeyNumber, uint8_t Type, uint8_t *Data) |
Writes a block of data to the card. | |
RWDMifareErr | AuthAllCards () |
Authorises all cards for the RWD. | |
void | command (uint8_t cmd, const uint8_t *params, int paramsLen, uint8_t *resp, size_t respLen, uint8_t ackOk, size_t ackOkMask) |
Executes a command. | |
bool | ready () |
Ready for a command / response is available. | |
bool | result (uint8_t *pAck=NULL) |
Get whether last command was succesful, and complete ack byte if a ptr is provided. |
Detailed Description
Used to interface to a OEM-Mifare RFID card reader/writer.
Definition at line 53 of file RWDMifare.h.
Member Enumeration Documentation
enum Ack_Code |
Acknowledge Codes: An enumerate of the masks of status code fields.
Definition at line 87 of file RWDMifare.h.
enum Aux_Data |
Auxilary Data: Values to set the function of OP0 and OP1.
Definition at line 136 of file RWDMifare.h.
enum Aux_Out_ReDir |
Aux Output Redirection Sets the output pin for OP0 data.
Definition at line 169 of file RWDMifare.h.
enum Byte_Order |
Aux output order Changes the byte order data is sent on the Aux output line.
Definition at line 183 of file RWDMifare.h.
enum EEPROM_ADDR |
EEPROM Address: Address constants for accessing the EEPROM Addresses.
Definition at line 102 of file RWDMifare.h.
enum Mifare_Icode |
Mode: Controls whether the device operates in MIFARE or ICODE mode (NB only Mifare supported, this is included for completion)
Definition at line 147 of file RWDMifare.h.
enum MifareCMD |
Commands: An enumerate of the commands supported by this class.
- Enumerator:
Definition at line 74 of file RWDMifare.h.
enum OP_Fmt |
OP Format Controls the output format of data on OP.
Definition at line 176 of file RWDMifare.h.
enum OP_Source_Data |
OP Source Sets the data output on OP0.
Definition at line 162 of file RWDMifare.h.
enum POLL_TIME |
Poll Times: Available values of the EEPROM location controlling Poll Time.
Definition at line 119 of file RWDMifare.h.
enum RWDMifareErr |
Error Codes: An enumerate of the possible error codes generated by the class.
- Enumerator:
Definition at line 63 of file RWDMifare.h.
enum Weigand_Parity |
Weigand Parity: Sets the Weigand Parity if the Weigand option is used NB not implemented.
Definition at line 155 of file RWDMifare.h.
Member Function Documentation
RWDMifare::RWDMifareErr AuthAllCards | ( | ) |
Authorises all cards for the RWD.
The Mifare Reader can contain up to 60 internal UIDs to match to a read UID. This method will write 0xFFFFFFFF to the first field so that all cards brought into detect will be able to be read from the mbed
Definition at line 146 of file RWDMifare.cpp.
void command | ( | uint8_t | cmd, |
const uint8_t * | params, | ||
int | paramsLen, | ||
uint8_t * | resp, | ||
size_t | respLen, | ||
uint8_t | ackOk, | ||
size_t | ackOkMask | ||
) | [inherited] |
Executes a command.
Destroys instance.
Executes the command cmd on the reader, with parameters set in params buffer of paramsLen length. The acknowledge byte sent back by the reader masked with ackOkMask must be equal to ackOk for the command to be considered a success. If so, the result is stored in buffer resp of length respLen. This is a non-blocking function, and ready() should be called to check completion. Please note that the buffers references must remain valid until the command has been executed.
Definition at line 36 of file RWDModule.cpp.
RWDMifare::RWDMifareErr getStatus | ( | uint8_t * | Status ) |
Gets the Reader Status.
Gets the status of the RFID card reader.
- Parameters:
-
Status - location will be filled with the status value
- See also:
- Ack_Code
Definition at line 85 of file RWDMifare.cpp.
RWDMifare::RWDMifareErr getUID | ( | uint8_t * | pUID, |
size_t * | pLen | ||
) |
Gets UID of card, as well as the length of.
Reads and returns the UID and length of UID of a card if in detect
- Parameters:
-
pUID must be at least 10-bytes long pLen will contain the length of the UID
- Returns:
- Error code
- See also:
- RWDMifareErr
Definition at line 93 of file RWDMifare.cpp.
RWDMifare::RWDMifareErr init | ( | ) |
Initialises the device.
Programs the EEPROM to configure in Mifare Mode
- Returns:
- Error code
- See also:
- RWDMifareErr
Definition at line 35 of file RWDMifare.cpp.
RWDMifare::RWDMifareErr printStatus | ( | uint8_t | Status ) |
Prints a description of status.
Method prints lines giving a brief explanation of the Status bits
- Parameters:
-
Status is the status byte received from getStatus()
- See also:
- Ack_Code, getStatus()
Definition at line 74 of file RWDMifare.cpp.
RWDMifare::RWDMifareErr Prog_EEPROM | ( | uint8_t | Address, |
uint8_t | Value | ||
) |
Programs the EEPROM.
Programs the EEPROM of the Card Reader
- Parameters:
-
Address to program. Enumerated by EEPROM_ADDR Value to write. Documented by Enums, depending on field writing to. Sets the internal value to change reader behaviour
- See also:
- EEPROM_ADDR, POLL_TIME, Aux_Data, Mifare_Icode, Weigand_Parity, OP_Source_Data, Aux_Out_ReDir, OP_Fmt, Byte_Order
Definition at line 54 of file RWDMifare.cpp.
RWDMifare::RWDMifareErr ReadBlock | ( | uint8_t | Addr, |
uint8_t | KeyNumber, | ||
uint8_t | Type, | ||
uint8_t * | Data | ||
) |
Reads a block of data from the card.
Reads a 16 byte block of memory from the card
- Parameters:
-
Addr is the block address (0-63 for 1k cards, 0-255 for 4k cards) to read from. Keynumber Key Number to use to access the card, between 0 and 31. Type is the key type to use. 0 = A, !0 = B. Data to store the data read back in. Should be at least 16 bytes in length.
Definition at line 174 of file RWDMifare.cpp.
RWDMifare::RWDMifareErr ReadBlock | ( | uint8_t | Addr, |
uint8_t | KeyNumber_Type, | ||
uint8_t * | Data | ||
) |
Reads a block of data from the card.
Reads a 16 byte block of memory from the card
- Parameters:
-
Addr is the block address (0-63 for 1k cards, 0-255 for 4k cards) to read from. Keynumber_Tpye is the combined Key Number and card type of the form 0xTxxKKKKK - T => A = 0; B = 1. K is 5 bit key number Data is the location that the block data is stored at. Should be at least 16 bytes in length.
Definition at line 159 of file RWDMifare.cpp.
bool ready | ( | ) | [inherited] |
Ready for a command / response is available.
Returns true if the previous command has been executed and an other command is ready to be sent.
Definition at line 62 of file RWDModule.cpp.
bool result | ( | uint8_t * | pAck = NULL ) |
[inherited] |
Get whether last command was succesful, and complete ack byte if a ptr is provided.
Returns true if the previous command was successful. If pAck is provided, the actual acknowledge byte returned by the reader is stored in that variable.
Definition at line 67 of file RWDModule.cpp.
RWDMifare::RWDMifareErr StoreKey | ( | uint8_t | KeyNumber, |
uint8_t * | Key | ||
) |
Stores an access key to the internal EEPROM Memory.
Writes a key to the internal memory to be used to access secured cards
- Parameters:
-
KeyNumber is the location the key is stored at and is used to reference the key in other operations Key is a 6 byte key to be written
Definition at line 122 of file RWDMifare.cpp.
RWDMifare::RWDMifareErr WriteBlock | ( | uint8_t | Addr, |
uint8_t | KeyNumber, | ||
uint8_t | Type, | ||
uint8_t * | Data | ||
) |
Writes a block of data to the card.
Writes an entire block of data to the RFID card WILL NOT WRITE TO A SECTION TRAILER. Incorrect writing to trailers can render the card useless. Therefore this method WILL NOT ALLOW writing to these to avoid accidentally bricking the card. Section trailers are located at Blocks 3, 7, 11 ... 63 for the 1k cards and lower part of 4k cards, and also at 15, 31, 47 ... Writing to these sections is the same protocol, but it is necessary to understand the Section Trailers before attempting to do so.
- Parameters:
-
Addr is the Block Address of a data field to write to. Keynumber Key Number to use to access the card, between 0 and 31. Type is the key type to use. 0 = A, !0 = B. Data is the source of the data to be written. Should be at least 16 bytes in length.
Definition at line 182 of file RWDMifare.cpp.
Generated on Wed Jul 13 2022 03:38:17 by
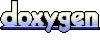