
Basic Read and Writer implementation for an OEM Mifare RWD Device.
Embed:
(wiki syntax)
Show/hide line numbers
RWDModule.h
00001 /* 00002 Copyright (c) 2010 Donatien Garnier (donatiengar [at] gmail [dot] com) 00003 00004 Permission is hereby granted, free of charge, to any person obtaining a copy 00005 of this software and associated documentation files (the "Software"), to deal 00006 in the Software without restriction, including without limitation the rights 00007 to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00008 copies of the Software, and to permit persons to whom the Software is 00009 furnished to do so, subject to the following conditions: 00010 00011 The above copyright notice and this permission notice shall be included in 00012 all copies or substantial portions of the Software. 00013 00014 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00015 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00016 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00017 AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00018 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00019 OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00020 THE SOFTWARE. 00021 */ 00022 00023 #ifndef RWD_MODULE_H 00024 #define RWD_MODULE_H 00025 00026 #include "mbed.h" 00027 00028 //!Generic driver for the RWD RFID Modules from IB Technology. 00029 /*! 00030 The RWD modules from IB Technology are RFID readers working with different frequencies and protocols but with a common instructions set and pinout. 00031 */ 00032 class RWDModule 00033 { 00034 public: 00035 //!Creates an instance of the class. 00036 /*! 00037 Connect module using serial port pins tx, rx and DigitalIn pin cts (clear-to-send). 00038 */ 00039 RWDModule(PinName tx, PinName rx, PinName cts); 00040 00041 /*! 00042 Destroys instance. 00043 */ 00044 //virtual (); 00045 00046 //!Executes a command. 00047 /*! 00048 Executes the command cmd on the reader, with parameters set in params buffer of paramsLen length. The acknowledge byte sent back by the reader masked with ackOkMask must be equal to ackOk for the command to be considered a success. If so, the result is stored in buffer resp of length respLen. 00049 This is a non-blocking function, and ready() should be called to check completion. 00050 Please note that the buffers references must remain valid until the command has been executed. 00051 */ 00052 void command(uint8_t cmd, const uint8_t* params, int paramsLen, uint8_t* resp, size_t respLen, uint8_t ackOk, size_t ackOkMask); //Ack Byte is not included in the resp buf 00053 00054 //!Ready for a command / response is available. 00055 /*! 00056 Returns true if the previous command has been executed and an other command is ready to be sent. 00057 */ 00058 bool ready(); 00059 00060 //!Get whether last command was succesful, and complete ack byte if a ptr is provided. 00061 /*! 00062 Returns true if the previous command was successful. If pAck is provided, the actual acknowledge byte returned by the reader is stored in that variable. 00063 */ 00064 bool result(uint8_t* pAck = NULL); 00065 00066 private: 00067 void intClearToSend(); //Called on interrupt when CTS line falls 00068 void intTx(); //Called on interrupt when TX buffer is not full anymore (bytes sent) 00069 void intRx(); //Called on interrrupt when RX buffer is not empty anymore (bytes received) 00070 00071 Serial m_serial; 00072 InterruptIn m_cts; 00073 00074 uint8_t m_cmd; 00075 uint8_t* m_paramsBuf; 00076 uint8_t* m_respBuf; 00077 size_t m_pos; 00078 size_t m_paramsLen; 00079 size_t m_respLen; 00080 00081 uint8_t m_ackOk; 00082 uint8_t m_ackOkMask; 00083 00084 uint8_t m_ack; 00085 00086 enum 00087 { 00088 READY, 00089 CMD_QUEUED, 00090 SENDING_CMD, 00091 WAITING_FOR_ACK, 00092 RECEIVING_ACK 00093 } m_state; 00094 00095 }; 00096 00097 #endif
Generated on Wed Jul 13 2022 03:38:17 by
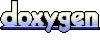