Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
wait_api.h
00001 /** Generic wait functions. 00002 * 00003 * These provide simple NOP type wait capabilities. 00004 * 00005 * Example: 00006 * @code 00007 * #include "mbed.h" 00008 * 00009 * DigitalOut heartbeat(LED1); 00010 * 00011 * int main() { 00012 * while (1) { 00013 * heartbeat = 1; 00014 * wait(0.5); 00015 * heartbeat = 0; 00016 * wait(0.5); 00017 * } 00018 * } 00019 */ 00020 00021 /* mbed Microcontroller Library - wait_api 00022 * Copyright (c) 2009 ARM Limited. All rights reserved. 00023 */ 00024 00025 #ifndef MBED_WAIT_API_H 00026 #define MBED_WAIT_API_H 00027 00028 #ifdef __cplusplus 00029 extern "C" { 00030 #endif 00031 00032 /** Waits for a number of seconds, with microsecond resolution (within 00033 * the accuracy of single precision floating point). 00034 * 00035 * @param s number of seconds to wait 00036 */ 00037 void wait(float s); 00038 00039 /** Waits a number of milliseconds. 00040 * 00041 * @param ms the whole number of milliseconds to wait 00042 */ 00043 void wait_ms(int ms); 00044 00045 /** Waits a number of microseconds. 00046 * 00047 * @param us the whole number of microseconds to wait 00048 */ 00049 void wait_us(int us); 00050 00051 #ifdef TARGET_LPC11U24 00052 /** Send the microcontroller to sleep 00053 * 00054 * The processor is setup ready for sleep, and sent to sleep using __WFI(). In this mode, the 00055 * system clock to the core is stopped until a reset or an interrupt occurs. This eliminates 00056 * dynamic power used by the processor, memory systems and buses. The processor, peripheral and 00057 * memory state are maintained, and the peripherals continue to work and can generate interrupts. 00058 * 00059 * The processor can be woken up by any internal peripheral interrupt or external pin interrupt. 00060 * 00061 * @note 00062 * The mbed interface semihosting is disconnected as part of going to sleep, and can not be restored. 00063 * Flash re-programming and the USB serial port will remain active, but the mbed program will no longer be 00064 * able to access the LocalFileSystem 00065 */ 00066 void sleep(void); 00067 00068 /** Send the microcontroller to deep sleep 00069 * 00070 * This processor is setup ready for deep sleep, and sent to sleep using __WFI(). This mode 00071 * has the same sleep features as sleep plus it powers down peripherals and clocks. All state 00072 * is still maintained. 00073 * 00074 * The processor can only be woken up by an external interrupt on a pin or a watchdog timer. 00075 * 00076 * @note 00077 * The mbed interface semihosting is disconnected as part of going to sleep, and can not be restored. 00078 * Flash re-programming and the USB serial port will remain active, but the mbed program will no longer be 00079 * able to access the LocalFileSystem 00080 */ 00081 void deepsleep(void); 00082 #endif 00083 00084 #ifdef __cplusplus 00085 } 00086 #endif 00087 00088 #endif
Generated on Tue Jul 12 2022 11:27:27 by
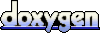