Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
vector_defns.h
00001 /* mbed Microcontroller Library - Vectors 00002 * Copyright (c) 2006-2009 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_VECTOR_DEFNS_H 00006 #define MBED_VECTOR_DEFNS_H 00007 00008 // Assember Macros 00009 #ifdef __ARMCC_VERSION 00010 #define EXPORT(x) EXPORT x 00011 #define WEAK_EXPORT(x) EXPORT x [WEAK] 00012 #define IMPORT(x) IMPORT x 00013 #define LABEL(x) x 00014 #else 00015 #define EXPORT(x) .global x 00016 #define WEAK_EXPORT(x) .weak x 00017 #define IMPORT(x) .global x 00018 #define LABEL(x) x: 00019 #endif 00020 00021 // RealMonitor 00022 // Requires RAM (0x40000040-0x4000011F) to be allocated by the linker 00023 00024 // RealMonitor entry points 00025 #define rm_init_entry 0x7fffff91 00026 #define rm_undef_handler 0x7fffffa0 00027 #define rm_prefetchabort_handler 0x7fffffb0 00028 #define rm_dataabort_handler 0x7fffffc0 00029 #define rm_irqhandler2 0x7fffffe0 00030 //#define rm_RunningToStopped 0x7ffff808 // ARM - MBED64 00031 #define rm_RunningToStopped 0x7ffff820 // ARM - PHAT40 00032 00033 // Unofficial RealMonitor entry points and variables 00034 #define RM_MSG_SWI 0x00940000 00035 #define StateP 0x40000040 00036 00037 // VIC register addresses 00038 #define VIC_Base 0xfffff000 00039 #define VICAddress_Offset 0xf00 00040 #define VICVectAddr2_Offset 0x108 00041 #define VICVectAddr3_Offset 0x10c 00042 #define VICIntEnClr_Offset 0x014 00043 #define VICIntEnClr (*(volatile unsigned long *)(VIC_Base + 0x014)) 00044 #define VICVectAddr2 (*(volatile unsigned long *)(VIC_Base + 0x108)) 00045 #define VICVectAddr3 (*(volatile unsigned long *)(VIC_Base + 0x10C)) 00046 00047 // ARM Mode bits and Interrupt flags in PSRs 00048 #define Mode_USR 0x10 00049 #define Mode_FIQ 0x11 00050 #define Mode_IRQ 0x12 00051 #define Mode_SVC 0x13 00052 #define Mode_ABT 0x17 00053 #define Mode_UND 0x1B 00054 #define Mode_SYS 0x1F 00055 #define I_Bit 0x80 // when I bit is set, IRQ is disabled 00056 #define F_Bit 0x40 // when F bit is set, FIQ is disabled 00057 00058 // MCU RAM 00059 #define LPC2368_RAM_ADDRESS 0x40000000 // RAM Base 00060 #define LPC2368_RAM_SIZE 0x8000 // 32KB 00061 00062 // ISR Stack Allocation 00063 #define UND_stack_size 0x00000040 00064 #define SVC_stack_size 0x00000040 00065 #define ABT_stack_size 0x00000040 00066 #define FIQ_stack_size 0x00000000 00067 #define IRQ_stack_size 0x00000040 00068 00069 #define ISR_stack_size (UND_stack_size + SVC_stack_size + ABT_stack_size + FIQ_stack_size + IRQ_stack_size) 00070 00071 // Full Descending Stack, so top-most stack points to just above the top of RAM 00072 #define LPC2368_STACK_TOP (LPC2368_RAM_ADDRESS + LPC2368_RAM_SIZE) 00073 #define USR_STACK_TOP (LPC2368_STACK_TOP - ISR_stack_size) 00074 00075 #endif
Generated on Tue Jul 12 2022 11:27:27 by
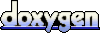