Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
Timer.h
00001 /* mbed Microcontroller Library - Timer 00002 * Copyright (c) 2007-2009 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_TIMER_H 00006 #define MBED_TIMER_H 00007 00008 #include "platform.h" 00009 #include "PinNames.h" 00010 #include "PeripheralNames.h" 00011 #include "Base.h" 00012 00013 namespace mbed { 00014 00015 /** A general purpose timer 00016 * 00017 * Example: 00018 * @code 00019 * // Count the time to toggle a LED 00020 * 00021 * #include "mbed.h" 00022 * 00023 * Timer timer; 00024 * DigitalOut led(LED1); 00025 * int begin, end; 00026 * 00027 * int main() { 00028 * timer.start(); 00029 * begin = timer.read_us(); 00030 * led = !led; 00031 * end = timer.read_us(); 00032 * printf("Toggle the led takes %d us", end - begin); 00033 * } 00034 * @endcode 00035 */ 00036 class Timer : public Base { 00037 00038 public: 00039 00040 Timer(const char *name = NULL); 00041 00042 /** Start the timer 00043 */ 00044 void start(); 00045 00046 /** Stop the timer 00047 */ 00048 void stop(); 00049 00050 /** Reset the timer to 0. 00051 * 00052 * If it was already counting, it will continue 00053 */ 00054 void reset(); 00055 00056 /** Get the time passed in seconds 00057 */ 00058 float read(); 00059 00060 /** Get the time passed in mili-seconds 00061 */ 00062 int read_ms(); 00063 00064 /** Get the time passed in micro-seconds 00065 */ 00066 int read_us(); 00067 00068 #ifdef MBED_OPERATORS 00069 operator float(); 00070 #endif 00071 00072 #ifdef MBED_RPC 00073 virtual const struct rpc_method *get_rpc_methods(); 00074 static struct rpc_class *get_rpc_class(); 00075 #endif 00076 00077 protected: 00078 00079 int slicetime(); 00080 int _running; // whether the timer is running 00081 unsigned int _start; // the start time of the latest slice 00082 int _time; // any accumulated time from previous slices 00083 00084 }; 00085 00086 } // namespace mbed 00087 00088 #endif 00089
Generated on Tue Jul 12 2022 11:27:27 by
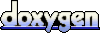