Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
Timeout.h
00001 /* mbed Microcontroller Library - Timeout 00002 * Copyright (c) 2007-2009 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_TIMEOUT_H 00006 #define MBED_TIMEOUT_H 00007 00008 #include "Ticker.h" 00009 00010 namespace mbed { 00011 00012 /** A Timeout is used to call a function at a point in the future 00013 * 00014 * You can use as many seperate Timeout objects as you require. 00015 * 00016 * Example: 00017 * @code 00018 * // Blink until timeout. 00019 * 00020 * #include "mbed.h" 00021 * 00022 * Timeout timeout; 00023 * DigitalOut led(LED1); 00024 * 00025 * int on = 1; 00026 * 00027 * void attimeout() { 00028 * on = 0; 00029 * } 00030 * 00031 * int main() { 00032 * timeout.attach(&attimeout, 5); 00033 * while(on) { 00034 * led = !led; 00035 * wait(0.2); 00036 * } 00037 * } 00038 * @endcode 00039 */ 00040 class Timeout : public Ticker { 00041 00042 #if 0 // For documentation 00043 00044 /** Attach a function to be called by the Timeout, specifiying the delay in seconds 00045 * 00046 * @param fptr pointer to the function to be called 00047 * @param t the time before the call in seconds 00048 */ 00049 void attach(void (*fptr)(void), float t) { 00050 attach_us(fptr, t * 1000000.0f); 00051 } 00052 00053 /** Attach a member function to be called by the Timeout, specifiying the delay in seconds 00054 * 00055 * @param tptr pointer to the object to call the member function on 00056 * @param mptr pointer to the member function to be called 00057 * @param t the time before the calls in seconds 00058 */ 00059 template<typename T> 00060 void attach(T* tptr, void (T::*mptr)(void), float t) { 00061 attach_us(tptr, mptr, t * 1000000.0f); 00062 } 00063 00064 /** Attach a function to be called by the Timeout, specifiying the delay in micro-seconds 00065 * 00066 * @param fptr pointer to the function to be called 00067 * @param t the time before the call in micro-seconds 00068 */ 00069 void attach_us(void (*fptr)(void), unsigned int t) { 00070 _function.attach(fptr); 00071 setup(t); 00072 } 00073 00074 /** Attach a member function to be called by the Timeout, specifiying the delay in micro-seconds 00075 * 00076 * @param tptr pointer to the object to call the member function on 00077 * @param mptr pointer to the member function to be called 00078 * @param t the time before the call in micro-seconds 00079 */ 00080 template<typename T> 00081 void attach_us(T* tptr, void (T::*mptr)(void), unsigned int t) { 00082 _function.attach(tptr, mptr); 00083 setup(t); 00084 } 00085 00086 /** Detach the function 00087 */ 00088 void detach(); 00089 00090 #endif 00091 00092 protected: 00093 00094 virtual void handler(); 00095 00096 }; 00097 00098 } // namespace mbed 00099 00100 #endif
Generated on Tue Jul 12 2022 11:27:27 by
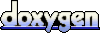