Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
Stream.h
00001 /* mbed Microcontroller Library - Stream 00002 * Copyright (c) 2007-2009 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_STREAM_H 00006 #define MBED_STREAM_H 00007 00008 #include "FileLike.h" 00009 #include "platform.h" 00010 #include <cstdio> 00011 00012 namespace mbed { 00013 00014 class Stream : public FileLike { 00015 00016 public: 00017 00018 Stream(const char *name = NULL); 00019 virtual ~Stream(); 00020 00021 int putc(int c) { 00022 fflush(_file); 00023 return std::fputc(c, _file); 00024 } 00025 int puts(const char *s) { 00026 fflush(_file); 00027 return std::fputs(s, _file); 00028 } 00029 int getc() { 00030 fflush(_file); 00031 return std::fgetc(_file); 00032 } 00033 char *gets(char *s, int size) { 00034 fflush(_file); 00035 return std::fgets(s,size,_file);; 00036 } 00037 int printf(const char* format, ...); 00038 int scanf(const char* format, ...); 00039 00040 operator std::FILE*() { return _file; } 00041 00042 #ifdef MBED_RPC 00043 virtual const struct rpc_method *get_rpc_methods(); 00044 #endif 00045 00046 protected: 00047 00048 virtual int close(); 00049 virtual ssize_t write(const void* buffer, size_t length); 00050 virtual ssize_t read(void* buffer, size_t length); 00051 virtual off_t lseek(off_t offset, int whence); 00052 virtual int isatty(); 00053 virtual int fsync(); 00054 virtual off_t flen(); 00055 00056 virtual int _putc(int c) = 0; 00057 virtual int _getc() = 0; 00058 00059 std::FILE *_file; 00060 00061 }; 00062 00063 } // namespace mbed 00064 00065 #endif 00066
Generated on Tue Jul 12 2022 11:27:27 by
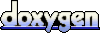