Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
PwmOut.h
00001 /* mbed Microcontroller Library - PwmOut 00002 * Copyright (c) 2007-2011 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_PWMOUT_H 00006 #define MBED_PWMOUT_H 00007 00008 #include "device.h" 00009 00010 #if DEVICE_PWMOUT 00011 00012 #include "platform.h" 00013 #include "PinNames.h" 00014 #include "PeripheralNames.h" 00015 #include "Base.h" 00016 00017 namespace mbed { 00018 00019 /** A pulse-width modulation digital output 00020 * 00021 * Example 00022 * @code 00023 * // Fade a led on. 00024 * #include "mbed.h" 00025 * 00026 * PwmOut led(LED1); 00027 * 00028 * int main() { 00029 * while(1) { 00030 * led = led + 0.01; 00031 * wait(0.2); 00032 * if(led == 1.0) { 00033 * led = 0; 00034 * } 00035 * } 00036 * } 00037 * @endcode 00038 * 00039 * @note 00040 * On the LPC1768 and LPC2368, the PWMs all share the same 00041 * period - if you change the period for one, you change it for all. 00042 * Although routines that change the period maintain the duty cycle 00043 * for its PWM, all other PWMs will require their duty cycle to be 00044 * refreshed. 00045 */ 00046 class PwmOut : public Base { 00047 00048 public: 00049 00050 /** Create a PwmOut connected to the specified pin 00051 * 00052 * @param pin PwmOut pin to connect to 00053 */ 00054 PwmOut(PinName pin, const char *name = NULL); 00055 00056 /** Set the ouput duty-cycle, specified as a percentage (float) 00057 * 00058 * @param value A floating-point value representing the output duty-cycle, 00059 * specified as a percentage. The value should lie between 00060 * 0.0f (representing on 0%) and 1.0f (representing on 100%). 00061 * Values outside this range will be saturated to 0.0f or 1.0f. 00062 */ 00063 void write(float value); 00064 00065 /** Return the current output duty-cycle setting, measured as a percentage (float) 00066 * 00067 * @returns 00068 * A floating-point value representing the current duty-cycle being output on the pin, 00069 * measured as a percentage. The returned value will lie between 00070 * 0.0f (representing on 0%) and 1.0f (representing on 100%). 00071 * 00072 * @note 00073 * This value may not match exactly the value set by a previous <write>. 00074 */ 00075 float read(); 00076 00077 /** Set the PWM period, specified in seconds (float), keeping the duty cycle the same. 00078 * 00079 * @note 00080 * The resolution is currently in microseconds; periods smaller than this 00081 * will be set to zero. 00082 */ 00083 void period(float seconds); 00084 00085 /** Set the PWM period, specified in milli-seconds (int), keeping the duty cycle the same. 00086 */ 00087 void period_ms(int ms); 00088 00089 /** Set the PWM period, specified in micro-seconds (int), keeping the duty cycle the same. 00090 */ 00091 void period_us(int us); 00092 00093 /** Set the PWM pulsewidth, specified in seconds (float), keeping the period the same. 00094 */ 00095 void pulsewidth(float seconds); 00096 00097 /** Set the PWM pulsewidth, specified in milli-seconds (int), keeping the period the same. 00098 */ 00099 void pulsewidth_ms(int ms); 00100 00101 /** Set the PWM pulsewidth, specified in micro-seconds (int), keeping the period the same. 00102 */ 00103 void pulsewidth_us(int us); 00104 00105 #ifdef MBED_OPERATORS 00106 /** A operator shorthand for write() 00107 */ 00108 PwmOut& operator= (float value); 00109 PwmOut& operator= (PwmOut& rhs); 00110 00111 /** An operator shorthand for read() 00112 */ 00113 operator float(); 00114 #endif 00115 00116 #ifdef MBED_RPC 00117 virtual const struct rpc_method *get_rpc_methods(); 00118 static struct rpc_class *get_rpc_class(); 00119 #endif 00120 00121 protected: 00122 00123 PWMName _pwm; 00124 00125 }; 00126 00127 } // namespace mbed 00128 00129 #endif 00130 00131 #endif
Generated on Tue Jul 12 2022 11:27:27 by
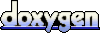