Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
PortIn.h
00001 /* mbed Microcontroller Library - PortInOut 00002 * Copyright (c) 2006-2011 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_PORTIN_H 00006 #define MBED_PORTIN_H 00007 00008 #include "device.h" 00009 00010 #if DEVICE_PORTIN 00011 00012 #include "PortNames.h" 00013 #include "PinNames.h" 00014 00015 namespace mbed { 00016 00017 /** A multiple pin digital input 00018 * 00019 * Example: 00020 * @code 00021 * // Switch on an LED if any of mbed pins 21-26 is high 00022 * 00023 * #include "mbed.h" 00024 * 00025 * PortIn p(Port2, 0x0000003F); // p21-p26 00026 * DigitalOut ind(LED4); 00027 * 00028 * int main() { 00029 * while(1) { 00030 * int pins = p.read(); 00031 * if(pins) { 00032 * ind = 1; 00033 * } else { 00034 * ind = 0; 00035 * } 00036 * } 00037 * } 00038 * @endcode 00039 */ 00040 class PortIn { 00041 public: 00042 00043 /** Create an PortIn, connected to the specified port 00044 * 00045 * @param port Port to connect to (Port0-Port5) 00046 * @param mask A bitmask to identify which bits in the port should be included (0 - ignore) 00047 */ 00048 PortIn(PortName port, int mask = 0xFFFFFFFF); 00049 00050 /** Read the value currently output on the port 00051 * 00052 * @returns 00053 * An integer with each bit corresponding to associated port pin setting 00054 */ 00055 int read(); 00056 00057 /** Set the input pin mode 00058 * 00059 * @param mode PullUp, PullDown, PullNone, OpenDrain 00060 */ 00061 void mode(PinMode mode); 00062 00063 /** A shorthand for read() 00064 */ 00065 operator int() { 00066 return read(); 00067 } 00068 00069 private: 00070 #if defined(TARGET_LPC1768) || defined(TARGET_LPC2368) 00071 LPC_GPIO_TypeDef *_gpio; 00072 #endif 00073 PortName _port; 00074 uint32_t _mask; 00075 }; 00076 00077 } // namespace mbed 00078 00079 #endif 00080 00081 #endif
Generated on Tue Jul 12 2022 11:27:27 by
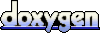