Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
PeripheralNames.h
00001 /* mbed Microcontroller Library - PeripheralNames 00002 * Copyright (C) 2008-2011 ARM Limited. All rights reserved. 00003 * 00004 * Provides the mappings for peripherals 00005 */ 00006 00007 #ifndef MBED_PERIPHERALNAMES_H 00008 #define MBED_PERIPHERALNAMES_H 00009 00010 #include "cmsis.h" 00011 00012 #ifdef __cplusplus 00013 extern "C" { 00014 #endif 00015 00016 #if defined(TARGET_LPC1768) || defined(TARGET_LPC2368) 00017 00018 enum UARTName { 00019 UART_0 = (int)LPC_UART0_BASE 00020 , UART_1 = (int)LPC_UART1_BASE 00021 , UART_2 = (int)LPC_UART2_BASE 00022 , UART_3 = (int)LPC_UART3_BASE 00023 }; 00024 typedef enum UARTName UARTName; 00025 00026 enum ADCName { 00027 ADC0_0 = 0 00028 , ADC0_1 00029 , ADC0_2 00030 , ADC0_3 00031 , ADC0_4 00032 , ADC0_5 00033 , ADC0_6 00034 , ADC0_7 00035 }; 00036 typedef enum ADCName ADCName; 00037 00038 enum DACName { 00039 DAC_0 = 0 00040 }; 00041 typedef enum DACName DACName; 00042 00043 enum SPIName { 00044 SPI_0 = (int)LPC_SSP0_BASE 00045 , SPI_1 = (int)LPC_SSP1_BASE 00046 }; 00047 typedef enum SPIName SPIName; 00048 00049 enum I2CName { 00050 I2C_0 = (int)LPC_I2C0_BASE 00051 , I2C_1 = (int)LPC_I2C1_BASE 00052 , I2C_2 = (int)LPC_I2C2_BASE 00053 }; 00054 typedef enum I2CName I2CName; 00055 00056 enum PWMName { 00057 PWM_1 = 1 00058 , PWM_2 00059 , PWM_3 00060 , PWM_4 00061 , PWM_5 00062 , PWM_6 00063 }; 00064 typedef enum PWMName PWMName; 00065 00066 enum TimerName { 00067 TIMER_0 = (int)LPC_TIM0_BASE 00068 , TIMER_1 = (int)LPC_TIM1_BASE 00069 , TIMER_2 = (int)LPC_TIM2_BASE 00070 , TIMER_3 = (int)LPC_TIM3_BASE 00071 }; 00072 typedef enum TimerName TimerName; 00073 00074 enum CANName { 00075 CAN_1 = (int)LPC_CAN1_BASE, 00076 CAN_2 = (int)LPC_CAN2_BASE 00077 }; 00078 typedef enum CANName CANName; 00079 00080 #define US_TICKER_TIMER TIMER_3 00081 #define US_TICKER_TIMER_IRQn TIMER3_IRQn 00082 00083 #elif defined(TARGET_LPC11U24) 00084 00085 enum UARTName { 00086 UART_0 = (int)LPC_USART_BASE 00087 }; 00088 typedef enum UARTName UARTName; 00089 00090 enum I2CName { 00091 I2C_0 = (int)LPC_I2C_BASE 00092 }; 00093 typedef enum I2CName I2CName; 00094 00095 enum TimerName { 00096 TIMER_0 = (int)LPC_CT32B0_BASE 00097 , TIMER_1 = (int)LPC_CT32B1_BASE 00098 }; 00099 typedef enum TimerName TimerName; 00100 00101 enum ADCName { 00102 ADC0_0 = 0 00103 , ADC0_1 00104 , ADC0_2 00105 , ADC0_3 00106 , ADC0_4 00107 , ADC0_5 00108 , ADC0_6 00109 , ADC0_7 00110 }; 00111 typedef enum ADCName ADCName; 00112 00113 enum SPIName { 00114 SPI_0 = (int)LPC_SSP0_BASE 00115 , SPI_1 = (int)LPC_SSP1_BASE 00116 }; 00117 typedef enum SPIName SPIName; 00118 00119 #define US_TICKER_TIMER TIMER_1 00120 #define US_TICKER_TIMER_IRQn TIMER_32_1_IRQn 00121 00122 typedef enum PWMName { 00123 PWM_1 = 0 00124 , PWM_2 00125 , PWM_3 00126 , PWM_4 00127 , PWM_5 00128 , PWM_6 00129 , PWM_7 00130 , PWM_8 00131 , PWM_9 00132 , PWM_10 00133 , PWM_11 00134 } PWMName; 00135 00136 #endif 00137 00138 #define STDIO_UART_TX USBTX 00139 #define STDIO_UART_RX USBRX 00140 #define STDIO_UART UART_0 00141 00142 #ifdef __cplusplus 00143 } 00144 #endif 00145 00146 #endif
Generated on Tue Jul 12 2022 11:27:26 by
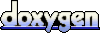