Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
LocalFileSystem.h
00001 /* mbed Microcontroller Library - LocalFileSystem 00002 * Copyright (c) 2008-2009 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_LOCALFILESYSTEM_H 00006 #define MBED_LOCALFILESYSTEM_H 00007 00008 #include "FileSystemLike.h" 00009 00010 namespace mbed { 00011 00012 FILEHANDLE local_file_open(const char* name, int flags); 00013 00014 class LocalFileHandle : public FileHandle { 00015 00016 public: 00017 LocalFileHandle(FILEHANDLE fh); 00018 00019 virtual int close(); 00020 00021 virtual ssize_t write(const void *buffer, size_t length); 00022 00023 virtual ssize_t read(void *buffer, size_t length); 00024 00025 virtual int isatty(); 00026 00027 virtual off_t lseek(off_t position, int whence); 00028 00029 virtual int fsync(); 00030 00031 virtual off_t flen(); 00032 00033 protected: 00034 FILEHANDLE _fh; 00035 int pos; 00036 }; 00037 00038 /** A filesystem for accessing the local mbed Microcontroller USB disk drive 00039 * 00040 * This allows programs to read and write files on the same disk drive that is used to program the 00041 * mbed Microcontroller. Once created, the standard C file access functions are used to open, 00042 * read and write files. 00043 * 00044 * Example: 00045 * @code 00046 * #include "mbed.h" 00047 * 00048 * LocalFileSystem local("local"); // Create the local filesystem under the name "local" 00049 * 00050 * int main() { 00051 * FILE *fp = fopen("/local/out.txt", "w"); // Open "out.txt" on the local file system for writing 00052 * fprintf(fp, "Hello World!"); 00053 * fclose(fp); 00054 * remove("/local/out.txt"); // Removes the file "out.txt" from the local file system 00055 * 00056 * DIR *d = opendir("/local"); // Opens the root directory of the local file system 00057 * struct dirent *p; 00058 * while((p = readdir(d)) != NULL) { // Print the names of the files in the local file system 00059 * printf("%s\n", p->d_name); // to stdout. 00060 * } 00061 * closedir(d); 00062 * } 00063 * @endcode 00064 * 00065 * @note 00066 * If the microcontroller program makes an access to the local drive, it will be marked as "removed" 00067 * on the Host computer. This means it is no longer accessible from the Host Computer. 00068 * 00069 * The drive will only re-appear when the microcontroller program exists. Note that if the program does 00070 * not exit, you will need to hold down reset on the mbed Microcontroller to be able to see the drive again! 00071 */ 00072 class LocalFileSystem : public FileSystemLike { 00073 00074 public: 00075 00076 LocalFileSystem(const char* n) : FileSystemLike(n) { 00077 00078 } 00079 00080 virtual FileHandle *open(const char* name, int flags); 00081 virtual int remove(const char *filename); 00082 virtual DirHandle *opendir(const char *name); 00083 }; 00084 00085 } // namespace mbed 00086 00087 #endif
Generated on Tue Jul 12 2022 11:27:26 by
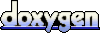