Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
LPC23xx.h
00001 /* mbed Microcontroller Library - LPC23xx CMSIS-like structs 00002 * Copyright (C) 2009 ARM Limited. All rights reserved. 00003 * 00004 * An LPC23xx header file, based on the CMSIS LPC17xx.h and old LPC23xx.h 00005 */ 00006 00007 #ifndef __LPC23xx_H 00008 #define __LPC23xx_H 00009 00010 #ifdef __cplusplus 00011 extern "C" { 00012 #endif 00013 00014 /* 00015 * ========================================================================== 00016 * ---------- Interrupt Number Definition ----------------------------------- 00017 * ========================================================================== 00018 */ 00019 00020 typedef enum IRQn 00021 { 00022 /****** LPC23xx Specific Interrupt Numbers *******************************************************/ 00023 WDT_IRQn = 0, /*!< Watchdog Timer Interrupt */ 00024 00025 TIMER0_IRQn = 4, /*!< Timer0 Interrupt */ 00026 TIMER1_IRQn = 5, /*!< Timer1 Interrupt */ 00027 UART0_IRQn = 6, /*!< UART0 Interrupt */ 00028 UART1_IRQn = 7, /*!< UART1 Interrupt */ 00029 PWM1_IRQn = 8, /*!< PWM1 Interrupt */ 00030 I2C0_IRQn = 9, /*!< I2C0 Interrupt */ 00031 SPI_IRQn = 10, /*!< SPI Interrupt */ 00032 SSP0_IRQn = 10, /*!< SSP0 Interrupt */ 00033 SSP1_IRQn = 11, /*!< SSP1 Interrupt */ 00034 PLL0_IRQn = 12, /*!< PLL0 Lock (Main PLL) Interrupt */ 00035 RTC_IRQn = 13, /*!< Real Time Clock Interrupt */ 00036 EINT0_IRQn = 14, /*!< External Interrupt 0 Interrupt */ 00037 EINT1_IRQn = 15, /*!< External Interrupt 1 Interrupt */ 00038 EINT2_IRQn = 16, /*!< External Interrupt 2 Interrupt */ 00039 EINT3_IRQn = 17, /*!< External Interrupt 3 Interrupt */ 00040 ADC_IRQn = 18, /*!< A/D Converter Interrupt */ 00041 I2C1_IRQn = 19, /*!< I2C1 Interrupt */ 00042 BOD_IRQn = 20, /*!< Brown-Out Detect Interrupt */ 00043 ENET_IRQn = 21, /*!< Ethernet Interrupt */ 00044 USB_IRQn = 22, /*!< USB Interrupt */ 00045 CAN_IRQn = 23, /*!< CAN Interrupt */ 00046 MIC_IRQn = 24, /*!< Multimedia Interface Controler */ 00047 DMA_IRQn = 25, /*!< General Purpose DMA Interrupt */ 00048 TIMER2_IRQn = 26, /*!< Timer2 Interrupt */ 00049 TIMER3_IRQn = 27, /*!< Timer3 Interrupt */ 00050 UART2_IRQn = 28, /*!< UART2 Interrupt */ 00051 UART3_IRQn = 29, /*!< UART3 Interrupt */ 00052 I2C2_IRQn = 30, /*!< I2C2 Interrupt */ 00053 I2S_IRQn = 31, /*!< I2S Interrupt */ 00054 } IRQn_Type ; 00055 00056 /* 00057 * ========================================================================== 00058 * ----------- Processor and Core Peripheral Section ------------------------ 00059 * ========================================================================== 00060 */ 00061 00062 /* Configuration of the ARM7 Processor and Core Peripherals */ 00063 #define __MPU_PRESENT 0 /*!< MPU present or not */ 00064 #define __NVIC_PRIO_BITS 4 /*!< Number of Bits used for Priority Levels */ 00065 #define __Vendor_SysTickConfig 0 /*!< Set to 1 if different SysTick Config is used */ 00066 00067 00068 #include <core_arm7.h> 00069 #include "system_LPC23xx.h" /* System Header */ 00070 00071 00072 /******************************************************************************/ 00073 /* Device Specific Peripheral registers structures */ 00074 /******************************************************************************/ 00075 #if defined ( __CC_ARM ) 00076 #pragma anon_unions 00077 #endif 00078 00079 /*------------- Vector Interupt Controler (VIC) ------------------------------*/ 00080 typedef struct 00081 { 00082 __I uint32_t IRQStatus; 00083 __I uint32_t FIQStatus; 00084 __I uint32_t RawIntr; 00085 __IO uint32_t IntSelect; 00086 __IO uint32_t IntEnable; 00087 __O uint32_t IntEnClr; 00088 __IO uint32_t SoftInt; 00089 __O uint32_t SoftIntClr; 00090 __IO uint32_t Protection; 00091 __IO uint32_t SWPriorityMask; 00092 __IO uint32_t RESERVED0[54]; 00093 __IO uint32_t VectAddr[32]; 00094 __IO uint32_t RESERVED1[32]; 00095 __IO uint32_t VectPriority[32]; 00096 __IO uint32_t RESERVED2[800]; 00097 __IO uint32_t Address; 00098 } LPC_VIC_TypeDef; 00099 00100 /*------------- System Control (SC) ------------------------------------------*/ 00101 typedef struct 00102 { 00103 __IO uint32_t MAMCR; 00104 __IO uint32_t MAMTIM; 00105 uint32_t RESERVED0[14]; 00106 __IO uint32_t MEMMAP; 00107 uint32_t RESERVED1[15]; 00108 __IO uint32_t PLL0CON; /* Clocking and Power Control */ 00109 __IO uint32_t PLL0CFG; 00110 __I uint32_t PLL0STAT; 00111 __O uint32_t PLL0FEED; 00112 uint32_t RESERVED2[12]; 00113 __IO uint32_t PCON; 00114 __IO uint32_t PCONP; 00115 uint32_t RESERVED3[15]; 00116 __IO uint32_t CCLKCFG; 00117 __IO uint32_t USBCLKCFG; 00118 __IO uint32_t CLKSRCSEL; 00119 uint32_t RESERVED4[12]; 00120 __IO uint32_t EXTINT; /* External Interrupts */ 00121 __IO uint32_t INTWAKE; 00122 __IO uint32_t EXTMODE; 00123 __IO uint32_t EXTPOLAR; 00124 uint32_t RESERVED6[12]; 00125 __IO uint32_t RSID; /* Reset */ 00126 __IO uint32_t CSPR; 00127 __IO uint32_t AHBCFG1; 00128 __IO uint32_t AHBCFG2; 00129 uint32_t RESERVED7[4]; 00130 __IO uint32_t SCS; /* Syscon Miscellaneous Registers */ 00131 __IO uint32_t IRCTRIM; /* Clock Dividers */ 00132 __IO uint32_t PCLKSEL0; 00133 __IO uint32_t PCLKSEL1; 00134 uint32_t RESERVED8[4]; 00135 __IO uint32_t USBIntSt; /* USB Device/OTG Interrupt Register */ 00136 uint32_t RESERVED9; 00137 // __IO uint32_t CLKOUTCFG; /* Clock Output Configuration */ 00138 } LPC_SC_TypeDef; 00139 00140 /*------------- Pin Connect Block (PINCON) -----------------------------------*/ 00141 typedef struct 00142 { 00143 __IO uint32_t PINSEL0; 00144 __IO uint32_t PINSEL1; 00145 __IO uint32_t PINSEL2; 00146 __IO uint32_t PINSEL3; 00147 __IO uint32_t PINSEL4; 00148 __IO uint32_t PINSEL5; 00149 __IO uint32_t PINSEL6; 00150 __IO uint32_t PINSEL7; 00151 __IO uint32_t PINSEL8; 00152 __IO uint32_t PINSEL9; 00153 __IO uint32_t PINSEL10; 00154 uint32_t RESERVED0[5]; 00155 __IO uint32_t PINMODE0; 00156 __IO uint32_t PINMODE1; 00157 __IO uint32_t PINMODE2; 00158 __IO uint32_t PINMODE3; 00159 __IO uint32_t PINMODE4; 00160 __IO uint32_t PINMODE5; 00161 __IO uint32_t PINMODE6; 00162 __IO uint32_t PINMODE7; 00163 __IO uint32_t PINMODE8; 00164 __IO uint32_t PINMODE9; 00165 __IO uint32_t PINMODE_OD0; 00166 __IO uint32_t PINMODE_OD1; 00167 __IO uint32_t PINMODE_OD2; 00168 __IO uint32_t PINMODE_OD3; 00169 __IO uint32_t PINMODE_OD4; 00170 } LPC_PINCON_TypeDef; 00171 00172 /*------------- General Purpose Input/Output (GPIO) --------------------------*/ 00173 typedef struct 00174 { 00175 __IO uint32_t FIODIR; 00176 uint32_t RESERVED0[3]; 00177 __IO uint32_t FIOMASK; 00178 __IO uint32_t FIOPIN; 00179 __IO uint32_t FIOSET; 00180 __O uint32_t FIOCLR; 00181 } LPC_GPIO_TypeDef; 00182 00183 typedef struct 00184 { 00185 __I uint32_t IntStatus; 00186 __I uint32_t IO0IntStatR; 00187 __I uint32_t IO0IntStatF; 00188 __O uint32_t IO0IntClr; 00189 __IO uint32_t IO0IntEnR; 00190 __IO uint32_t IO0IntEnF; 00191 uint32_t RESERVED0[3]; 00192 __I uint32_t IO2IntStatR; 00193 __I uint32_t IO2IntStatF; 00194 __O uint32_t IO2IntClr; 00195 __IO uint32_t IO2IntEnR; 00196 __IO uint32_t IO2IntEnF; 00197 } LPC_GPIOINT_TypeDef; 00198 00199 /*------------- Timer (TIM) --------------------------------------------------*/ 00200 typedef struct 00201 { 00202 __IO uint32_t IR; 00203 __IO uint32_t TCR; 00204 __IO uint32_t TC; 00205 __IO uint32_t PR; 00206 __IO uint32_t PC; 00207 __IO uint32_t MCR; 00208 __IO uint32_t MR0; 00209 __IO uint32_t MR1; 00210 __IO uint32_t MR2; 00211 __IO uint32_t MR3; 00212 __IO uint32_t CCR; 00213 __I uint32_t CR0; 00214 __I uint32_t CR1; 00215 uint32_t RESERVED0[2]; 00216 __IO uint32_t EMR; 00217 uint32_t RESERVED1[12]; 00218 __IO uint32_t CTCR; 00219 } LPC_TIM_TypeDef; 00220 00221 /*------------- Pulse-Width Modulation (PWM) ---------------------------------*/ 00222 typedef struct 00223 { 00224 __IO uint32_t IR; 00225 __IO uint32_t TCR; 00226 __IO uint32_t TC; 00227 __IO uint32_t PR; 00228 __IO uint32_t PC; 00229 __IO uint32_t MCR; 00230 __IO uint32_t MR0; 00231 __IO uint32_t MR1; 00232 __IO uint32_t MR2; 00233 __IO uint32_t MR3; 00234 __IO uint32_t CCR; 00235 __I uint32_t CR0; 00236 __I uint32_t CR1; 00237 __I uint32_t CR2; 00238 __I uint32_t CR3; 00239 uint32_t RESERVED0; 00240 __IO uint32_t MR4; 00241 __IO uint32_t MR5; 00242 __IO uint32_t MR6; 00243 __IO uint32_t PCR; 00244 __IO uint32_t LER; 00245 uint32_t RESERVED1[7]; 00246 __IO uint32_t CTCR; 00247 } LPC_PWM_TypeDef; 00248 00249 /*------------- Universal Asynchronous Receiver Transmitter (UART) -----------*/ 00250 typedef struct 00251 { 00252 union { 00253 __I uint8_t RBR; 00254 __O uint8_t THR; 00255 __IO uint8_t DLL; 00256 uint32_t RESERVED0; 00257 }; 00258 union { 00259 __IO uint8_t DLM; 00260 __IO uint32_t IER; 00261 }; 00262 union { 00263 __I uint32_t IIR; 00264 __O uint8_t FCR; 00265 }; 00266 __IO uint8_t LCR; 00267 uint8_t RESERVED1[7]; 00268 __IO uint8_t LSR; 00269 uint8_t RESERVED2[7]; 00270 __IO uint8_t SCR; 00271 uint8_t RESERVED3[3]; 00272 __IO uint32_t ACR; 00273 __IO uint8_t ICR; 00274 uint8_t RESERVED4[3]; 00275 __IO uint8_t FDR; 00276 uint8_t RESERVED5[7]; 00277 __IO uint8_t TER; 00278 uint8_t RESERVED6[27]; 00279 __IO uint8_t RS485CTRL; 00280 uint8_t RESERVED7[3]; 00281 __IO uint8_t ADRMATCH; 00282 } LPC_UART_TypeDef; 00283 00284 typedef struct 00285 { 00286 union { 00287 __I uint8_t RBR; 00288 __O uint8_t THR; 00289 __IO uint8_t DLL; 00290 uint32_t RESERVED0; 00291 }; 00292 union { 00293 __IO uint8_t DLM; 00294 __IO uint32_t IER; 00295 }; 00296 union { 00297 __I uint32_t IIR; 00298 __O uint8_t FCR; 00299 }; 00300 __IO uint8_t LCR; 00301 uint8_t RESERVED1[3]; 00302 __IO uint8_t MCR; 00303 uint8_t RESERVED2[3]; 00304 __IO uint8_t LSR; 00305 uint8_t RESERVED3[3]; 00306 __IO uint8_t MSR; 00307 uint8_t RESERVED4[3]; 00308 __IO uint8_t SCR; 00309 uint8_t RESERVED5[3]; 00310 __IO uint32_t ACR; 00311 uint32_t RESERVED6; 00312 __IO uint32_t FDR; 00313 uint32_t RESERVED7; 00314 __IO uint8_t TER; 00315 uint8_t RESERVED8[27]; 00316 __IO uint8_t RS485CTRL; 00317 uint8_t RESERVED9[3]; 00318 __IO uint8_t ADRMATCH; 00319 uint8_t RESERVED10[3]; 00320 __IO uint8_t RS485DLY; 00321 } LPC_UART1_TypeDef; 00322 00323 /*------------- Serial Peripheral Interface (SPI) ----------------------------*/ 00324 typedef struct 00325 { 00326 __IO uint32_t SPCR; 00327 __I uint32_t SPSR; 00328 __IO uint32_t SPDR; 00329 __IO uint32_t SPCCR; 00330 uint32_t RESERVED0[3]; 00331 __IO uint32_t SPINT; 00332 } LPC_SPI_TypeDef; 00333 00334 /*------------- Synchronous Serial Communication (SSP) -----------------------*/ 00335 typedef struct 00336 { 00337 __IO uint32_t CR0; 00338 __IO uint32_t CR1; 00339 __IO uint32_t DR; 00340 __I uint32_t SR; 00341 __IO uint32_t CPSR; 00342 __IO uint32_t IMSC; 00343 __IO uint32_t RIS; 00344 __IO uint32_t MIS; 00345 __IO uint32_t ICR; 00346 __IO uint32_t DMACR; 00347 } LPC_SSP_TypeDef; 00348 00349 /*------------- Inter-Integrated Circuit (I2C) -------------------------------*/ 00350 typedef struct 00351 { 00352 __IO uint32_t I2CONSET; 00353 __I uint32_t I2STAT; 00354 __IO uint32_t I2DAT; 00355 __IO uint32_t I2ADR0; 00356 __IO uint32_t I2SCLH; 00357 __IO uint32_t I2SCLL; 00358 __O uint32_t I2CONCLR; 00359 __IO uint32_t MMCTRL; 00360 __IO uint32_t I2ADR1; 00361 __IO uint32_t I2ADR2; 00362 __IO uint32_t I2ADR3; 00363 __I uint32_t I2DATA_BUFFER; 00364 __IO uint32_t I2MASK0; 00365 __IO uint32_t I2MASK1; 00366 __IO uint32_t I2MASK2; 00367 __IO uint32_t I2MASK3; 00368 } LPC_I2C_TypeDef; 00369 00370 /*------------- Inter IC Sound (I2S) -----------------------------------------*/ 00371 typedef struct 00372 { 00373 __IO uint32_t I2SDAO; 00374 __I uint32_t I2SDAI; 00375 __O uint32_t I2STXFIFO; 00376 __I uint32_t I2SRXFIFO; 00377 __I uint32_t I2SSTATE; 00378 __IO uint32_t I2SDMA1; 00379 __IO uint32_t I2SDMA2; 00380 __IO uint32_t I2SIRQ; 00381 __IO uint32_t I2STXRATE; 00382 __IO uint32_t I2SRXRATE; 00383 __IO uint32_t I2STXBITRATE; 00384 __IO uint32_t I2SRXBITRATE; 00385 __IO uint32_t I2STXMODE; 00386 __IO uint32_t I2SRXMODE; 00387 } LPC_I2S_TypeDef; 00388 00389 /*------------- Real-Time Clock (RTC) ----------------------------------------*/ 00390 typedef struct 00391 { 00392 __IO uint8_t ILR; 00393 uint8_t RESERVED0[3]; 00394 __IO uint8_t CTC; 00395 uint8_t RESERVED1[3]; 00396 __IO uint8_t CCR; 00397 uint8_t RESERVED2[3]; 00398 __IO uint8_t CIIR; 00399 uint8_t RESERVED3[3]; 00400 __IO uint8_t AMR; 00401 uint8_t RESERVED4[3]; 00402 __I uint32_t CTIME0; 00403 __I uint32_t CTIME1; 00404 __I uint32_t CTIME2; 00405 __IO uint8_t SEC; 00406 uint8_t RESERVED5[3]; 00407 __IO uint8_t MIN; 00408 uint8_t RESERVED6[3]; 00409 __IO uint8_t HOUR; 00410 uint8_t RESERVED7[3]; 00411 __IO uint8_t DOM; 00412 uint8_t RESERVED8[3]; 00413 __IO uint8_t DOW; 00414 uint8_t RESERVED9[3]; 00415 __IO uint16_t DOY; 00416 uint16_t RESERVED10; 00417 __IO uint8_t MONTH; 00418 uint8_t RESERVED11[3]; 00419 __IO uint16_t YEAR; 00420 uint16_t RESERVED12; 00421 __IO uint32_t CALIBRATION; 00422 __IO uint32_t GPREG0; 00423 __IO uint32_t GPREG1; 00424 __IO uint32_t GPREG2; 00425 __IO uint32_t GPREG3; 00426 __IO uint32_t GPREG4; 00427 __IO uint8_t WAKEUPDIS; 00428 uint8_t RESERVED13[3]; 00429 __IO uint8_t PWRCTRL; 00430 uint8_t RESERVED14[3]; 00431 __IO uint8_t ALSEC; 00432 uint8_t RESERVED15[3]; 00433 __IO uint8_t ALMIN; 00434 uint8_t RESERVED16[3]; 00435 __IO uint8_t ALHOUR; 00436 uint8_t RESERVED17[3]; 00437 __IO uint8_t ALDOM; 00438 uint8_t RESERVED18[3]; 00439 __IO uint8_t ALDOW; 00440 uint8_t RESERVED19[3]; 00441 __IO uint16_t ALDOY; 00442 uint16_t RESERVED20; 00443 __IO uint8_t ALMON; 00444 uint8_t RESERVED21[3]; 00445 __IO uint16_t ALYEAR; 00446 uint16_t RESERVED22; 00447 } LPC_RTC_TypeDef; 00448 00449 /*------------- Watchdog Timer (WDT) -----------------------------------------*/ 00450 typedef struct 00451 { 00452 __IO uint8_t WDMOD; 00453 uint8_t RESERVED0[3]; 00454 __IO uint32_t WDTC; 00455 __O uint8_t WDFEED; 00456 uint8_t RESERVED1[3]; 00457 __I uint32_t WDTV; 00458 __IO uint32_t WDCLKSEL; 00459 } LPC_WDT_TypeDef; 00460 00461 /*------------- Analog-to-Digital Converter (ADC) ----------------------------*/ 00462 typedef struct 00463 { 00464 __IO uint32_t ADCR; 00465 __IO uint32_t ADGDR; 00466 uint32_t RESERVED0; 00467 __IO uint32_t ADINTEN; 00468 __I uint32_t ADDR0; 00469 __I uint32_t ADDR1; 00470 __I uint32_t ADDR2; 00471 __I uint32_t ADDR3; 00472 __I uint32_t ADDR4; 00473 __I uint32_t ADDR5; 00474 __I uint32_t ADDR6; 00475 __I uint32_t ADDR7; 00476 __I uint32_t ADSTAT; 00477 __IO uint32_t ADTRM; 00478 } LPC_ADC_TypeDef; 00479 00480 /*------------- Digital-to-Analog Converter (DAC) ----------------------------*/ 00481 typedef struct 00482 { 00483 __IO uint32_t DACR; 00484 __IO uint32_t DACCTRL; 00485 __IO uint16_t DACCNTVAL; 00486 } LPC_DAC_TypeDef; 00487 00488 /*------------- Multimedia Card Interface (MCI) ------------------------------*/ 00489 typedef struct 00490 { 00491 __IO uint32_t MCIPower; /* Power control */ 00492 __IO uint32_t MCIClock; /* Clock control */ 00493 __IO uint32_t MCIArgument; 00494 __IO uint32_t MMCCommand; 00495 __I uint32_t MCIRespCmd; 00496 __I uint32_t MCIResponse0; 00497 __I uint32_t MCIResponse1; 00498 __I uint32_t MCIResponse2; 00499 __I uint32_t MCIResponse3; 00500 __IO uint32_t MCIDataTimer; 00501 __IO uint32_t MCIDataLength; 00502 __IO uint32_t MCIDataCtrl; 00503 __I uint32_t MCIDataCnt; 00504 } LPC_MCI_TypeDef; 00505 00506 /*------------- Controller Area Network (CAN) --------------------------------*/ 00507 typedef struct 00508 { 00509 __IO uint32_t mask[512]; /* ID Masks */ 00510 } LPC_CANAF_RAM_TypeDef; 00511 00512 typedef struct /* Acceptance Filter Registers */ 00513 { 00514 __IO uint32_t AFMR; 00515 __IO uint32_t SFF_sa; 00516 __IO uint32_t SFF_GRP_sa; 00517 __IO uint32_t EFF_sa; 00518 __IO uint32_t EFF_GRP_sa; 00519 __IO uint32_t ENDofTable; 00520 __I uint32_t LUTerrAd; 00521 __I uint32_t LUTerr; 00522 } LPC_CANAF_TypeDef; 00523 00524 typedef struct /* Central Registers */ 00525 { 00526 __I uint32_t CANTxSR; 00527 __I uint32_t CANRxSR; 00528 __I uint32_t CANMSR; 00529 } LPC_CANCR_TypeDef; 00530 00531 typedef struct /* Controller Registers */ 00532 { 00533 __IO uint32_t MOD; 00534 __O uint32_t CMR; 00535 __IO uint32_t GSR; 00536 __I uint32_t ICR; 00537 __IO uint32_t IER; 00538 __IO uint32_t BTR; 00539 __IO uint32_t EWL; 00540 __I uint32_t SR; 00541 __IO uint32_t RFS; 00542 __IO uint32_t RID; 00543 __IO uint32_t RDA; 00544 __IO uint32_t RDB; 00545 __IO uint32_t TFI1; 00546 __IO uint32_t TID1; 00547 __IO uint32_t TDA1; 00548 __IO uint32_t TDB1; 00549 __IO uint32_t TFI2; 00550 __IO uint32_t TID2; 00551 __IO uint32_t TDA2; 00552 __IO uint32_t TDB2; 00553 __IO uint32_t TFI3; 00554 __IO uint32_t TID3; 00555 __IO uint32_t TDA3; 00556 __IO uint32_t TDB3; 00557 } LPC_CAN_TypeDef; 00558 00559 /*------------- General Purpose Direct Memory Access (GPDMA) -----------------*/ 00560 typedef struct /* Common Registers */ 00561 { 00562 __I uint32_t DMACIntStat; 00563 __I uint32_t DMACIntTCStat; 00564 __O uint32_t DMACIntTCClear; 00565 __I uint32_t DMACIntErrStat; 00566 __O uint32_t DMACIntErrClr; 00567 __I uint32_t DMACRawIntTCStat; 00568 __I uint32_t DMACRawIntErrStat; 00569 __I uint32_t DMACEnbldChns; 00570 __IO uint32_t DMACSoftBReq; 00571 __IO uint32_t DMACSoftSReq; 00572 __IO uint32_t DMACSoftLBReq; 00573 __IO uint32_t DMACSoftLSReq; 00574 __IO uint32_t DMACConfig; 00575 __IO uint32_t DMACSync; 00576 } LPC_GPDMA_TypeDef; 00577 00578 typedef struct /* Channel Registers */ 00579 { 00580 __IO uint32_t DMACCSrcAddr; 00581 __IO uint32_t DMACCDestAddr; 00582 __IO uint32_t DMACCLLI; 00583 __IO uint32_t DMACCControl; 00584 __IO uint32_t DMACCConfig; 00585 } LPC_GPDMACH_TypeDef; 00586 00587 /*------------- Universal Serial Bus (USB) -----------------------------------*/ 00588 typedef struct 00589 { 00590 __I uint32_t HcRevision; /* USB Host Registers */ 00591 __IO uint32_t HcControl; 00592 __IO uint32_t HcCommandStatus; 00593 __IO uint32_t HcInterruptStatus; 00594 __IO uint32_t HcInterruptEnable; 00595 __IO uint32_t HcInterruptDisable; 00596 __IO uint32_t HcHCCA; 00597 __I uint32_t HcPeriodCurrentED; 00598 __IO uint32_t HcControlHeadED; 00599 __IO uint32_t HcControlCurrentED; 00600 __IO uint32_t HcBulkHeadED; 00601 __IO uint32_t HcBulkCurrentED; 00602 __I uint32_t HcDoneHead; 00603 __IO uint32_t HcFmInterval; 00604 __I uint32_t HcFmRemaining; 00605 __I uint32_t HcFmNumber; 00606 __IO uint32_t HcPeriodicStart; 00607 __IO uint32_t HcLSTreshold; 00608 __IO uint32_t HcRhDescriptorA; 00609 __IO uint32_t HcRhDescriptorB; 00610 __IO uint32_t HcRhStatus; 00611 __IO uint32_t HcRhPortStatus1; 00612 __IO uint32_t HcRhPortStatus2; 00613 uint32_t RESERVED0[40]; 00614 __I uint32_t Module_ID; 00615 00616 __I uint32_t OTGIntSt; /* USB On-The-Go Registers */ 00617 __IO uint32_t OTGIntEn; 00618 __O uint32_t OTGIntSet; 00619 __O uint32_t OTGIntClr; 00620 __IO uint32_t OTGStCtrl; 00621 __IO uint32_t OTGTmr; 00622 uint32_t RESERVED1[58]; 00623 00624 __I uint32_t USBDevIntSt; /* USB Device Interrupt Registers */ 00625 __IO uint32_t USBDevIntEn; 00626 __O uint32_t USBDevIntClr; 00627 __O uint32_t USBDevIntSet; 00628 00629 __O uint32_t USBCmdCode; /* USB Device SIE Command Registers */ 00630 __I uint32_t USBCmdData; 00631 00632 __I uint32_t USBRxData; /* USB Device Transfer Registers */ 00633 __O uint32_t USBTxData; 00634 __I uint32_t USBRxPLen; 00635 __O uint32_t USBTxPLen; 00636 __IO uint32_t USBCtrl; 00637 __O uint32_t USBDevIntPri; 00638 00639 __I uint32_t USBEpIntSt; /* USB Device Endpoint Interrupt Regs */ 00640 __IO uint32_t USBEpIntEn; 00641 __O uint32_t USBEpIntClr; 00642 __O uint32_t USBEpIntSet; 00643 __O uint32_t USBEpIntPri; 00644 00645 __IO uint32_t USBReEp; /* USB Device Endpoint Realization Reg*/ 00646 __O uint32_t USBEpInd; 00647 __IO uint32_t USBMaxPSize; 00648 00649 __I uint32_t USBDMARSt; /* USB Device DMA Registers */ 00650 __O uint32_t USBDMARClr; 00651 __O uint32_t USBDMARSet; 00652 uint32_t RESERVED2[9]; 00653 __IO uint32_t USBUDCAH; 00654 __I uint32_t USBEpDMASt; 00655 __O uint32_t USBEpDMAEn; 00656 __O uint32_t USBEpDMADis; 00657 __I uint32_t USBDMAIntSt; 00658 __IO uint32_t USBDMAIntEn; 00659 uint32_t RESERVED3[2]; 00660 __I uint32_t USBEoTIntSt; 00661 __O uint32_t USBEoTIntClr; 00662 __O uint32_t USBEoTIntSet; 00663 __I uint32_t USBNDDRIntSt; 00664 __O uint32_t USBNDDRIntClr; 00665 __O uint32_t USBNDDRIntSet; 00666 __I uint32_t USBSysErrIntSt; 00667 __O uint32_t USBSysErrIntClr; 00668 __O uint32_t USBSysErrIntSet; 00669 uint32_t RESERVED4[15]; 00670 00671 __I uint32_t I2C_RX; /* USB OTG I2C Registers */ 00672 __O uint32_t I2C_WO; 00673 __I uint32_t I2C_STS; 00674 __IO uint32_t I2C_CTL; 00675 __IO uint32_t I2C_CLKHI; 00676 __O uint32_t I2C_CLKLO; 00677 uint32_t RESERVED5[823]; 00678 00679 union { 00680 __IO uint32_t USBClkCtrl; /* USB Clock Control Registers */ 00681 __IO uint32_t OTGClkCtrl; 00682 }; 00683 union { 00684 __I uint32_t USBClkSt; 00685 __I uint32_t OTGClkSt; 00686 }; 00687 } LPC_USB_TypeDef; 00688 00689 /*------------- Ethernet Media Access Controller (EMAC) ----------------------*/ 00690 typedef struct 00691 { 00692 __IO uint32_t MAC1; /* MAC Registers */ 00693 __IO uint32_t MAC2; 00694 __IO uint32_t IPGT; 00695 __IO uint32_t IPGR; 00696 __IO uint32_t CLRT; 00697 __IO uint32_t MAXF; 00698 __IO uint32_t SUPP; 00699 __IO uint32_t TEST; 00700 __IO uint32_t MCFG; 00701 __IO uint32_t MCMD; 00702 __IO uint32_t MADR; 00703 __O uint32_t MWTD; 00704 __I uint32_t MRDD; 00705 __I uint32_t MIND; 00706 uint32_t RESERVED0[2]; 00707 __IO uint32_t SA0; 00708 __IO uint32_t SA1; 00709 __IO uint32_t SA2; 00710 uint32_t RESERVED1[45]; 00711 __IO uint32_t Command; /* Control Registers */ 00712 __I uint32_t Status; 00713 __IO uint32_t RxDescriptor; 00714 __IO uint32_t RxStatus; 00715 __IO uint32_t RxDescriptorNumber; 00716 __I uint32_t RxProduceIndex; 00717 __IO uint32_t RxConsumeIndex; 00718 __IO uint32_t TxDescriptor; 00719 __IO uint32_t TxStatus; 00720 __IO uint32_t TxDescriptorNumber; 00721 __IO uint32_t TxProduceIndex; 00722 __I uint32_t TxConsumeIndex; 00723 uint32_t RESERVED2[10]; 00724 __I uint32_t TSV0; 00725 __I uint32_t TSV1; 00726 __I uint32_t RSV; 00727 uint32_t RESERVED3[3]; 00728 __IO uint32_t FlowControlCounter; 00729 __I uint32_t FlowControlStatus; 00730 uint32_t RESERVED4[34]; 00731 __IO uint32_t RxFilterCtrl; /* Rx Filter Registers */ 00732 __IO uint32_t RxFilterWoLStatus; 00733 __IO uint32_t RxFilterWoLClear; 00734 uint32_t RESERVED5; 00735 __IO uint32_t HashFilterL; 00736 __IO uint32_t HashFilterH; 00737 uint32_t RESERVED6[882]; 00738 __I uint32_t IntStatus; /* Module Control Registers */ 00739 __IO uint32_t IntEnable; 00740 __O uint32_t IntClear; 00741 __O uint32_t IntSet; 00742 uint32_t RESERVED7; 00743 __IO uint32_t PowerDown; 00744 uint32_t RESERVED8; 00745 __IO uint32_t Module_ID; 00746 } LPC_EMAC_TypeDef; 00747 00748 #if defined ( __CC_ARM ) 00749 #pragma no_anon_unions 00750 #endif 00751 00752 /******************************************************************************/ 00753 /* Peripheral memory map */ 00754 /******************************************************************************/ 00755 /* Base addresses */ 00756 00757 /* AHB Peripheral # 0 */ 00758 00759 /* 00760 #define FLASH_BASE (0x00000000UL) 00761 #define RAM_BASE (0x10000000UL) 00762 #define GPIO_BASE (0x2009C000UL) 00763 #define APB0_BASE (0x40000000UL) 00764 #define APB1_BASE (0x40080000UL) 00765 #define AHB_BASE (0x50000000UL) 00766 #define CM3_BASE (0xE0000000UL) 00767 */ 00768 00769 // TODO - #define VIC_BASE_ADDR 0xFFFFF000 00770 00771 #define LPC_WDT_BASE (0xE0000000) 00772 #define LPC_TIM0_BASE (0xE0004000) 00773 #define LPC_TIM1_BASE (0xE0008000) 00774 #define LPC_UART0_BASE (0xE000C000) 00775 #define LPC_UART1_BASE (0xE0010000) 00776 #define LPC_PWM1_BASE (0xE0018000) 00777 #define LPC_I2C0_BASE (0xE001C000) 00778 #define LPC_SPI_BASE (0xE0020000) 00779 #define LPC_RTC_BASE (0xE0024000) 00780 #define LPC_GPIOINT_BASE (0xE0028080) 00781 #define LPC_PINCON_BASE (0xE002C000) 00782 #define LPC_SSP1_BASE (0xE0030000) 00783 #define LPC_ADC_BASE (0xE0034000) 00784 #define LPC_CANAF_RAM_BASE (0xE0038000) 00785 #define LPC_CANAF_BASE (0xE003C000) 00786 #define LPC_CANCR_BASE (0xE0040000) 00787 #define LPC_CAN1_BASE (0xE0044000) 00788 #define LPC_CAN2_BASE (0xE0048000) 00789 #define LPC_I2C1_BASE (0xE005C000) 00790 #define LPC_SSP0_BASE (0xE0068000) 00791 #define LPC_DAC_BASE (0xE006C000) 00792 #define LPC_TIM2_BASE (0xE0070000) 00793 #define LPC_TIM3_BASE (0xE0074000) 00794 #define LPC_UART2_BASE (0xE0078000) 00795 #define LPC_UART3_BASE (0xE007C000) 00796 #define LPC_I2C2_BASE (0xE0080000) 00797 #define LPC_I2S_BASE (0xE0088000) 00798 #define LPC_MCI_BASE (0xE008C000) 00799 #define LPC_SC_BASE (0xE01FC000) 00800 #define LPC_EMAC_BASE (0xFFE00000) 00801 #define LPC_GPDMA_BASE (0xFFE04000) 00802 #define LPC_GPDMACH0_BASE (0xFFE04100) 00803 #define LPC_GPDMACH1_BASE (0xFFE04120) 00804 #define LPC_USB_BASE (0xFFE0C000) 00805 #define LPC_VIC_BASE (0xFFFFF000) 00806 00807 /* GPIOs */ 00808 #define LPC_GPIO0_BASE (0x3FFFC000) 00809 #define LPC_GPIO1_BASE (0x3FFFC020) 00810 #define LPC_GPIO2_BASE (0x3FFFC040) 00811 #define LPC_GPIO3_BASE (0x3FFFC060) 00812 #define LPC_GPIO4_BASE (0x3FFFC080) 00813 00814 00815 /******************************************************************************/ 00816 /* Peripheral declaration */ 00817 /******************************************************************************/ 00818 #define LPC_SC (( LPC_SC_TypeDef *) LPC_SC_BASE) 00819 #define LPC_GPIO0 (( LPC_GPIO_TypeDef *) LPC_GPIO0_BASE) 00820 #define LPC_GPIO1 (( LPC_GPIO_TypeDef *) LPC_GPIO1_BASE) 00821 #define LPC_GPIO2 (( LPC_GPIO_TypeDef *) LPC_GPIO2_BASE) 00822 #define LPC_GPIO3 (( LPC_GPIO_TypeDef *) LPC_GPIO3_BASE) 00823 #define LPC_GPIO4 (( LPC_GPIO_TypeDef *) LPC_GPIO4_BASE) 00824 #define LPC_WDT (( LPC_WDT_TypeDef *) LPC_WDT_BASE) 00825 #define LPC_TIM0 (( LPC_TIM_TypeDef *) LPC_TIM0_BASE) 00826 #define LPC_TIM1 (( LPC_TIM_TypeDef *) LPC_TIM1_BASE) 00827 #define LPC_TIM2 (( LPC_TIM_TypeDef *) LPC_TIM2_BASE) 00828 #define LPC_TIM3 (( LPC_TIM_TypeDef *) LPC_TIM3_BASE) 00829 #define LPC_UART0 (( LPC_UART_TypeDef *) LPC_UART0_BASE) 00830 #define LPC_UART1 (( LPC_UART1_TypeDef *) LPC_UART1_BASE) 00831 #define LPC_UART2 (( LPC_UART_TypeDef *) LPC_UART2_BASE) 00832 #define LPC_UART3 (( LPC_UART_TypeDef *) LPC_UART3_BASE) 00833 #define LPC_PWM1 (( LPC_PWM_TypeDef *) LPC_PWM1_BASE) 00834 #define LPC_I2C0 (( LPC_I2C_TypeDef *) LPC_I2C0_BASE) 00835 #define LPC_I2C1 (( LPC_I2C_TypeDef *) LPC_I2C1_BASE) 00836 #define LPC_I2C2 (( LPC_I2C_TypeDef *) LPC_I2C2_BASE) 00837 #define LPC_I2S (( LPC_I2S_TypeDef *) LPC_I2S_BASE) 00838 #define LPC_SPI (( LPC_SPI_TypeDef *) LPC_SPI_BASE) 00839 #define LPC_RTC (( LPC_RTC_TypeDef *) LPC_RTC_BASE) 00840 #define LPC_GPIOINT (( LPC_GPIOINT_TypeDef *) LPC_GPIOINT_BASE) 00841 #define LPC_PINCON (( LPC_PINCON_TypeDef *) LPC_PINCON_BASE) 00842 #define LPC_SSP0 (( LPC_SSP_TypeDef *) LPC_SSP0_BASE) 00843 #define LPC_SSP1 (( LPC_SSP_TypeDef *) LPC_SSP1_BASE) 00844 #define LPC_ADC (( LPC_ADC_TypeDef *) LPC_ADC_BASE) 00845 #define LPC_DAC (( LPC_DAC_TypeDef *) LPC_DAC_BASE) 00846 #define LPC_CANAF_RAM ((LPC_CANAF_RAM_TypeDef *) LPC_CANAF_RAM_BASE) 00847 #define LPC_CANAF (( LPC_CANAF_TypeDef *) LPC_CANAF_BASE) 00848 #define LPC_CANCR (( LPC_CANCR_TypeDef *) LPC_CANCR_BASE) 00849 #define LPC_CAN1 (( LPC_CAN_TypeDef *) LPC_CAN1_BASE) 00850 #define LPC_CAN2 (( LPC_CAN_TypeDef *) LPC_CAN2_BASE) 00851 #define LPC_MCI (( LPC_MCI_TypeDef *) LPC_MCI_BASE) 00852 #define LPC_EMAC (( LPC_EMAC_TypeDef *) LPC_EMAC_BASE) 00853 #define LPC_GPDMA (( LPC_GPDMA_TypeDef *) LPC_GPDMA_BASE) 00854 #define LPC_GPDMACH0 (( LPC_GPDMACH_TypeDef *) LPC_GPDMACH0_BASE) 00855 #define LPC_GPDMACH1 (( LPC_GPDMACH_TypeDef *) LPC_GPDMACH1_BASE) 00856 #define LPC_USB (( LPC_USB_TypeDef *) LPC_USB_BASE) 00857 #define LPC_VIC (( LPC_VIC_TypeDef *) LPC_VIC_BASE) 00858 00859 #ifdef __cplusplus 00860 } 00861 #endif 00862 00863 #endif // __LPC23xx_H 00864
Generated on Tue Jul 12 2022 11:27:26 by
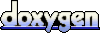