Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
I2CSlave.h
00001 /* mbed Microcontroller Library - I2CSlave 00002 * Copyright (c) 2007-2011 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_I2C_SLAVE_H 00006 #define MBED_I2C_SLAVE_H 00007 00008 #include "device.h" 00009 00010 #if DEVICE_I2CSLAVE 00011 00012 #include "platform.h" 00013 #include "PinNames.h" 00014 #include "PeripheralNames.h" 00015 #include "Base.h" 00016 00017 namespace mbed { 00018 00019 /** An I2C Slave, used for communicating with an I2C Master device 00020 * 00021 * Example: 00022 * @code 00023 * // Simple I2C responder 00024 * #include <mbed.h> 00025 * 00026 * I2CSlave slave(p9, p10); 00027 * 00028 * int main() { 00029 * char buf[10]; 00030 * char msg[] = "Slave!"; 00031 * 00032 * slave.address(0xA0); 00033 * while (1) { 00034 * int i = slave.receive(); 00035 * switch (i) { 00036 * case I2CSlave::ReadAddressed: 00037 * slave.write(msg, strlen(msg) + 1); // Includes null char 00038 * break; 00039 * case I2CSlave::WriteGeneral: 00040 * slave.read(buf, 10); 00041 * printf("Read G: %s\n", buf); 00042 * break; 00043 * case I2CSlave::WriteAddressed: 00044 * slave.read(buf, 10); 00045 * printf("Read A: %s\n", buf); 00046 * break; 00047 * } 00048 * for(int i = 0; i < 10; i++) buf[i] = 0; // Clear buffer 00049 * } 00050 * } 00051 * @endcode 00052 */ 00053 class I2CSlave : public Base { 00054 00055 public: 00056 00057 enum RxStatus { 00058 NoData = 0 00059 , ReadAddressed = 1 00060 , WriteGeneral = 2 00061 , WriteAddressed = 3 00062 }; 00063 00064 /** Create an I2C Slave interface, connected to the specified pins. 00065 * 00066 * @param sda I2C data line pin 00067 * @param scl I2C clock line pin 00068 */ 00069 I2CSlave(PinName sda, PinName scl, const char *name = NULL); 00070 00071 /** Set the frequency of the I2C interface 00072 * 00073 * @param hz The bus frequency in hertz 00074 */ 00075 void frequency(int hz); 00076 00077 /** Checks to see if this I2C Slave has been addressed. 00078 * 00079 * @returns 00080 * A status indicating if the device has been addressed, and how 00081 * - NoData - the slave has not been addressed 00082 * - ReadAddressed - the master has requested a read from this slave 00083 * - WriteAddressed - the master is writing to this slave 00084 * - WriteGeneral - the master is writing to all slave 00085 */ 00086 int receive(void); 00087 00088 /** Read from an I2C master. 00089 * 00090 * @param data pointer to the byte array to read data in to 00091 * @param length maximum number of bytes to read 00092 * 00093 * @returns 00094 * 0 on success, 00095 * non-0 otherwise 00096 */ 00097 int read(char *data, int length); 00098 00099 /** Read a single byte from an I2C master. 00100 * 00101 * @returns 00102 * the byte read 00103 */ 00104 int read(void); 00105 00106 /** Write to an I2C master. 00107 * 00108 * @param data pointer to the byte array to be transmitted 00109 * @param length the number of bytes to transmite 00110 * 00111 * @returns 00112 * 0 on success, 00113 * non-0 otherwise 00114 */ 00115 int write(const char *data, int length); 00116 00117 /** Write a single byte to an I2C master. 00118 * 00119 * @data the byte to write 00120 * 00121 * @returns 00122 * '1' if an ACK was received, 00123 * '0' otherwise 00124 */ 00125 int write(int data); 00126 00127 /** Sets the I2C slave address. 00128 * 00129 * @param address The address to set for the slave (ignoring the least 00130 * signifcant bit). If set to 0, the slave will only respond to the 00131 * general call address. 00132 */ 00133 void address(int address); 00134 00135 /** Reset the I2C slave back into the known ready receiving state. 00136 */ 00137 void stop(void); 00138 00139 protected: 00140 00141 I2CName _i2c; 00142 }; 00143 00144 } // namespace mbed 00145 00146 #endif 00147 00148 #endif 00149
Generated on Tue Jul 12 2022 11:27:26 by
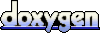