Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
FunctionPointer.h
00001 /* mbed Microcontroller Library - FunctionPointer 00002 * Copyright (c) 2007-2009 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_FUNCTIONPOINTER_H 00006 #define MBED_FUNCTIONPOINTER_H 00007 00008 #include <string.h> 00009 00010 namespace mbed { 00011 00012 /** A class for storing and calling a pointer to a static or member void function 00013 */ 00014 class FunctionPointer { 00015 00016 public: 00017 00018 /** Create a FunctionPointer, attaching a static function 00019 * 00020 * @param function The void static function to attach (default is none) 00021 */ 00022 FunctionPointer(void (*function)(void) = 0); 00023 00024 /** Create a FunctionPointer, attaching a member function 00025 * 00026 * @param object The object pointer to invoke the member function on (i.e. the this pointer) 00027 * @param function The address of the void member function to attach 00028 */ 00029 template<typename T> 00030 FunctionPointer(T *object, void (T::*member)(void)) { 00031 attach(object, member); 00032 } 00033 00034 /** Attach a static function 00035 * 00036 * @param function The void static function to attach (default is none) 00037 */ 00038 void attach(void (*function)(void) = 0); 00039 00040 /** Attach a member function 00041 * 00042 * @param object The object pointer to invoke the member function on (i.e. the this pointer) 00043 * @param function The address of the void member function to attach 00044 */ 00045 template<typename T> 00046 void attach(T *object, void (T::*member)(void)) { 00047 _object = static_cast<void*>(object); 00048 memcpy(_member, (char*)&member, sizeof(member)); 00049 _membercaller = &FunctionPointer::membercaller<T>; 00050 _function = 0; 00051 } 00052 00053 /** Call the attached static or member function 00054 */ 00055 void call(); 00056 00057 private: 00058 00059 template<typename T> 00060 static void membercaller(void *object, char *member) { 00061 T* o = static_cast<T*>(object); 00062 void (T::*m)(void); 00063 memcpy((char*)&m, member, sizeof(m)); 00064 (o->*m)(); 00065 } 00066 00067 /** Static function pointer - 0 if none attached 00068 */ 00069 void (*_function)(void); 00070 00071 /** Object this pointer - 0 if none attached 00072 */ 00073 void *_object; 00074 00075 /** Raw member function pointer storage - converted back by registered _membercaller 00076 */ 00077 char _member[16]; 00078 00079 /** Registered membercaller function to convert back and call _member on _object 00080 */ 00081 void (*_membercaller)(void*, char*); 00082 00083 }; 00084 00085 } // namespace mbed 00086 00087 #endif
Generated on Tue Jul 12 2022 11:27:26 by
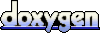