Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
FileSystemLike.h
00001 /* mbed Microcontroller Library - FileSystemLike 00002 * Copyright (c) 2008-2009 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_FILESYSTEMLIKE_H 00006 #define MBED_FILESYSTEMLIKE_H 00007 00008 #ifdef __ARMCC_VERSION 00009 # define O_RDONLY 0 00010 # define O_WRONLY 1 00011 # define O_RDWR 2 00012 # define O_CREAT 0x0200 00013 # define O_TRUNC 0x0400 00014 # define O_APPEND 0x0008 00015 typedef int mode_t; 00016 #else 00017 # include <sys/fcntl.h> 00018 #endif 00019 #include "Base.h" 00020 #include "FileHandle.h" 00021 #include "DirHandle.h" 00022 00023 namespace mbed { 00024 00025 /** A filesystem-like object is one that can be used to open files 00026 * though it by fopen("/name/filename", mode) 00027 * 00028 * Implementations must define at least open (the default definitions 00029 * of the rest of the functions just return error values). 00030 */ 00031 class FileSystemLike : public Base { 00032 00033 public: 00034 00035 /** FileSystemLike constructor 00036 * 00037 * @param name The name to use for the filesystem. 00038 */ 00039 FileSystemLike(const char *name) : Base(name) {} 00040 00041 /** Opens a file from the filesystem 00042 * 00043 * @param filename The name of the file to open. 00044 * @param flags One of O_RDONLY, O_WRONLY, or O_RDWR, OR'd with 00045 * zero or more of O_CREAT, O_TRUNC, or O_APPEND. 00046 * 00047 * @returns 00048 * A pointer to a FileHandle object representing the 00049 * file on success, or NULL on failure. 00050 */ 00051 virtual FileHandle *open(const char *filename, int flags) = 0; 00052 00053 /** Remove a file from the filesystem. 00054 * 00055 * @param filename the name of the file to remove. 00056 * @param returns 0 on success, -1 on failure. 00057 */ 00058 virtual int remove(const char *filename) { return -1; }; 00059 00060 /** Rename a file in the filesystem. 00061 * 00062 * @param oldname the name of the file to rename. 00063 * @param newname the name to rename it to. 00064 * 00065 * @returns 00066 * 0 on success, 00067 * -1 on failure. 00068 */ 00069 virtual int rename(const char *oldname, const char *newname) { return -1; }; 00070 00071 /** Opens a directory in the filesystem and returns a DirHandle 00072 * representing the directory stream. 00073 * 00074 * @param name The name of the directory to open. 00075 * 00076 * @returns 00077 * A DirHandle representing the directory stream, or 00078 * NULL on failure. 00079 */ 00080 virtual DirHandle *opendir(const char *name) { return NULL; }; 00081 00082 /** Creates a directory in the filesystem. 00083 * 00084 * @param name The name of the directory to create. 00085 * @param mode The permissions to create the directory with. 00086 * 00087 * @returns 00088 * 0 on success, 00089 * -1 on failure. 00090 */ 00091 virtual int mkdir(const char *name, mode_t mode) { return -1; } 00092 00093 // TODO other filesystem functions (mkdir, rm, rn, ls etc) 00094 00095 }; 00096 00097 } // namespace mbed 00098 00099 #endif
Generated on Tue Jul 12 2022 11:27:26 by
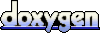