Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
FileHandle.h
00001 /* mbed Microcontroller Library - FileHandler 00002 * Copyright (c) 2007-2009 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_FILEHANDLE_H 00006 #define MBED_FILEHANDLE_H 00007 00008 typedef int FILEHANDLE; 00009 00010 #include <stdio.h> 00011 #ifdef __ARMCC_VERSION 00012 typedef int ssize_t; 00013 typedef long off_t; 00014 #else 00015 #include <sys/types.h> 00016 #endif 00017 00018 namespace mbed { 00019 00020 /** An OO equivalent of the internal FILEHANDLE variable 00021 * and associated _sys_* functions. 00022 * 00023 * FileHandle is an abstract class, needing at least sys_write and 00024 * sys_read to be implmented for a simple interactive device. 00025 * 00026 * No one ever directly tals to/instanciates a FileHandle - it gets 00027 * created by FileSystem, and wrapped up by stdio. 00028 */ 00029 class FileHandle { 00030 00031 public: 00032 00033 /** Write the contents of a buffer to the file 00034 * 00035 * @param buffer the buffer to write from 00036 * @param length the number of characters to write 00037 * 00038 * @returns 00039 * The number of characters written (possibly 0) on success, -1 on error. 00040 */ 00041 virtual ssize_t write(const void* buffer, size_t length) = 0; 00042 00043 /** Close the file 00044 * 00045 * @returns 00046 * Zero on success, -1 on error. 00047 */ 00048 virtual int close() = 0; 00049 00050 /** Function read 00051 * Reads the contents of the file into a buffer 00052 * 00053 * @param buffer the buffer to read in to 00054 * @param length the number of characters to read 00055 * 00056 * @returns 00057 * The number of characters read (zero at end of file) on success, -1 on error. 00058 */ 00059 virtual ssize_t read(void* buffer, size_t length) = 0; 00060 00061 /** Check if the handle is for a interactive terminal device. 00062 * If so, line buffered behaviour is used by default 00063 * 00064 * @returns 00065 * 1 if it is a terminal, 00066 * 0 otherwise 00067 */ 00068 virtual int isatty() = 0 ; 00069 00070 /** Move the file position to a given offset from a given location. 00071 * 00072 * @param offset The offset from whence to move to 00073 * @param whence SEEK_SET for the start of the file, SEEK_CUR for the 00074 * current file position, or SEEK_END for the end of the file. 00075 * 00076 * @returns 00077 * new file position on success, 00078 * -1 on failure or unsupported 00079 */ 00080 virtual off_t lseek(off_t offset, int whence) = 0; 00081 00082 /** Flush any buffers associated with the FileHandle, ensuring it 00083 * is up to date on disk 00084 * 00085 * @returns 00086 * 0 on success or un-needed, 00087 * -1 on error 00088 */ 00089 virtual int fsync() = 0; 00090 00091 virtual off_t flen() { 00092 /* remember our current position */ 00093 off_t pos = lseek(0, SEEK_CUR); 00094 if(pos == -1) return -1; 00095 /* seek to the end to get the file length */ 00096 off_t res = lseek(0, SEEK_END); 00097 /* return to our old position */ 00098 lseek(pos, SEEK_SET); 00099 return res; 00100 } 00101 00102 }; 00103 00104 } // namespace mbed 00105 00106 #endif 00107
Generated on Tue Jul 12 2022 11:27:26 by
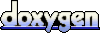