Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
DirHandle.h
00001 /* mbed Microcontroller Library - DirHandler 00002 * Copyright (c) 2008-2009 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_DIRHANDLE_H 00006 #define MBED_DIRHANDLE_H 00007 00008 #ifdef __ARMCC_VERSION 00009 # define NAME_MAX 255 00010 typedef int mode_t; 00011 #else 00012 # include <sys/syslimits.h> 00013 #endif 00014 #include "FileHandle.h" 00015 00016 struct dirent { 00017 char d_name[NAME_MAX+1]; 00018 }; 00019 00020 namespace mbed { 00021 00022 /** Represents a directory stream. Objects of this type are returned 00023 * by a FileSystemLike's opendir method. Implementations must define 00024 * at least closedir, readdir and rewinddir. 00025 * 00026 * If a FileSystemLike class defines the opendir method, then the 00027 * directories of an object of that type can be accessed by 00028 * DIR *d = opendir("/example/directory") (or opendir("/example") 00029 * to open the root of the filesystem), and then using readdir(d) etc. 00030 * 00031 * The root directory is considered to contain all FileLike and 00032 * FileSystemLike objects, so the DIR* returned by opendir("/") will 00033 * reflect this. 00034 */ 00035 class DirHandle { 00036 00037 public: 00038 /** Closes the directory. 00039 * 00040 * @returns 00041 * 0 on success, 00042 * -1 on error. 00043 */ 00044 virtual int closedir()=0; 00045 00046 /** Return the directory entry at the current position, and 00047 * advances the position to the next entry. 00048 * 00049 * @returns 00050 * A pointer to a dirent structure representing the 00051 * directory entry at the current position, or NULL on reaching 00052 * end of directory or error. 00053 */ 00054 virtual struct dirent *readdir()=0; 00055 00056 /** Resets the position to the beginning of the directory. 00057 */ 00058 virtual void rewinddir()=0; 00059 00060 /** Returns the current position of the DirHandle. 00061 * 00062 * @returns 00063 * the current position, 00064 * -1 on error. 00065 */ 00066 virtual off_t telldir() { return -1; } 00067 00068 /** Sets the position of the DirHandle. 00069 * 00070 * @param location The location to seek to. Must be a value returned by telldir. 00071 */ 00072 virtual void seekdir(off_t location) { } 00073 00074 }; 00075 00076 } // namespace mbed 00077 00078 typedef mbed::DirHandle DIR; 00079 00080 extern "C" { 00081 DIR *opendir(const char*); 00082 struct dirent *readdir(DIR *); 00083 int closedir(DIR*); 00084 void rewinddir(DIR*); 00085 long telldir(DIR*); 00086 void seekdir(DIR*, long); 00087 int mkdir(const char *name, mode_t n); 00088 }; 00089 00090 #endif /* MBED_DIRHANDLE_H */
Generated on Tue Jul 12 2022 11:27:26 by
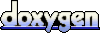