Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
DigitalOut.h
00001 /* mbed Microcontroller Library - DigitalOut 00002 * Copyright (c) 2006-2011 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_DIGITALOUT_H 00006 #define MBED_DIGITALOUT_H 00007 00008 #include "platform.h" 00009 #include "PinNames.h" 00010 #include "PeripheralNames.h" 00011 #include "Base.h" 00012 00013 namespace mbed { 00014 00015 /** A digital output, used for setting the state of a pin 00016 * 00017 * Example: 00018 * @code 00019 * // Toggle a LED 00020 * #include "mbed.h" 00021 * 00022 * DigitalOut led(LED1); 00023 * 00024 * int main() { 00025 * while(1) { 00026 * led = !led; 00027 * wait(0.2); 00028 * } 00029 * } 00030 * @endcode 00031 */ 00032 class DigitalOut : public Base { 00033 00034 public: 00035 00036 /** Create a DigitalOut connected to the specified pin 00037 * 00038 * @param pin DigitalOut pin to connect to 00039 */ 00040 DigitalOut(PinName pin, const char* name = NULL); 00041 00042 /** Set the output, specified as 0 or 1 (int) 00043 * 00044 * @param value An integer specifying the pin output value, 00045 * 0 for logical 0, 1 (or any other non-zero value) for logical 1 00046 */ 00047 void write(int value) { 00048 00049 #if defined(TARGET_LPC1768) || defined(TARGET_LPC2368) 00050 00051 if(value) { 00052 _gpio->FIOSET = _mask; 00053 } else { 00054 _gpio->FIOCLR = _mask; 00055 } 00056 00057 #elif defined(TARGET_LPC11U24) 00058 00059 if(value) { 00060 LPC_GPIO->SET[_index] = _mask; 00061 } else { 00062 LPC_GPIO->CLR[_index] = _mask; 00063 } 00064 #endif 00065 00066 } 00067 00068 /** Return the output setting, represented as 0 or 1 (int) 00069 * 00070 * @returns 00071 * an integer representing the output setting of the pin, 00072 * 0 for logical 0, 1 for logical 1 00073 */ 00074 int read() { 00075 #if defined(TARGET_LPC1768) || defined(TARGET_LPC2368) 00076 return ((_gpio->FIOPIN & _mask) ? 1 : 0); 00077 #elif defined(TARGET_LPC11U24) 00078 return ((LPC_GPIO->PIN[_index] & _mask) ? 1 : 0); 00079 #endif 00080 00081 } 00082 00083 00084 #ifdef MBED_OPERATORS 00085 /** A shorthand for write() 00086 */ 00087 DigitalOut& operator= (int value) { 00088 write(value); 00089 return *this; 00090 } 00091 00092 DigitalOut& operator= (DigitalOut& rhs) { 00093 write(rhs.read()); 00094 return *this; 00095 } 00096 00097 00098 /** A shorthand for read() 00099 */ 00100 operator int() { 00101 return read(); 00102 } 00103 00104 #endif 00105 00106 #ifdef MBED_RPC 00107 virtual const struct rpc_method *get_rpc_methods(); 00108 static struct rpc_class *get_rpc_class(); 00109 #endif 00110 00111 protected: 00112 00113 PinName _pin; 00114 00115 #if defined(TARGET_LPC1768) || defined(TARGET_LPC2368) 00116 LPC_GPIO_TypeDef *_gpio; 00117 #elif defined(TARGET_LPC11U24) 00118 int _index; 00119 #endif 00120 00121 uint32_t _mask; 00122 00123 00124 }; 00125 00126 } // namespace mbed 00127 00128 #endif
Generated on Tue Jul 12 2022 11:27:26 by
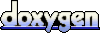