Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
DigitalIn.h
00001 /* mbed Microcontroller Library - DigitalIn 00002 * Copyright (c) 2006-2011 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_DIGITALIN_H 00006 #define MBED_DIGITALIN_H 00007 00008 #include "platform.h" 00009 #include "PinNames.h" 00010 #include "PeripheralNames.h" 00011 #include "Base.h" 00012 00013 namespace mbed { 00014 00015 /** A digital input, used for reading the state of a pin 00016 * 00017 * Example: 00018 * @code 00019 * // Flash an LED while a DigitalIn is true 00020 * 00021 * #include "mbed.h" 00022 * 00023 * DigitalIn enable(p5); 00024 * DigitalOut led(LED1); 00025 * 00026 * int main() { 00027 * while(1) { 00028 * if(enable) { 00029 * led = !led; 00030 * } 00031 * wait(0.25); 00032 * } 00033 * } 00034 * @endcode 00035 */ 00036 class DigitalIn : public Base { 00037 00038 public: 00039 00040 /** Create a DigitalIn connected to the specified pin 00041 * 00042 * @param pin DigitalIn pin to connect to 00043 * @param name (optional) A string to identify the object 00044 */ 00045 DigitalIn(PinName pin, const char *name = NULL); 00046 00047 /** Read the input, represented as 0 or 1 (int) 00048 * 00049 * @returns 00050 * An integer representing the state of the input pin, 00051 * 0 for logical 0, 1 for logical 1 00052 */ 00053 int read() { 00054 #if defined(TARGET_LPC1768) || defined(TARGET_LPC2368) 00055 return ((_gpio->FIOPIN & _mask) ? 1 : 0); 00056 #elif defined(TARGET_LPC11U24) 00057 return ((LPC_GPIO->PIN[_index] & _mask) ? 1 : 0); 00058 #endif 00059 } 00060 00061 00062 /** Set the input pin mode 00063 * 00064 * @param mode PullUp, PullDown, PullNone, OpenDrain 00065 */ 00066 void mode(PinMode pull); 00067 00068 #ifdef MBED_OPERATORS 00069 /** An operator shorthand for read() 00070 */ 00071 operator int() { 00072 return read(); 00073 } 00074 00075 #endif 00076 00077 #ifdef MBED_RPC 00078 virtual const struct rpc_method *get_rpc_methods(); 00079 static struct rpc_class *get_rpc_class(); 00080 #endif 00081 00082 protected: 00083 00084 PinName _pin; 00085 #if defined(TARGET_LPC1768) || defined(TARGET_LPC2368) 00086 LPC_GPIO_TypeDef *_gpio; 00087 #elif defined(TARGET_LPC11U24) 00088 int _index; 00089 #endif 00090 uint32_t _mask; 00091 00092 }; 00093 00094 } // namespace mbed 00095 00096 #endif 00097
Generated on Tue Jul 12 2022 11:27:26 by
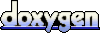