Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
DigitalInOut.h
00001 /* mbed Microcontroller Library - DigitalInOut 00002 * Copyright (c) 2006-2011 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_DIGITALINOUT_H 00006 #define MBED_DIGITALINOUT_H 00007 00008 #include "platform.h" 00009 #include "PinNames.h" 00010 #include "PeripheralNames.h" 00011 #include "Base.h" 00012 00013 namespace mbed { 00014 00015 /** A digital input/output, used for setting or reading a bi-directional pin 00016 */ 00017 class DigitalInOut : public Base { 00018 00019 public: 00020 00021 /** Create a DigitalInOut connected to the specified pin 00022 * 00023 * @param pin DigitalInOut pin to connect to 00024 */ 00025 DigitalInOut(PinName pin, const char* name = NULL); 00026 00027 /** Set the output, specified as 0 or 1 (int) 00028 * 00029 * @param value An integer specifying the pin output value, 00030 * 0 for logical 0, 1 (or any other non-zero value) for logical 1 00031 */ 00032 void write(int value) { 00033 #if defined(TARGET_LPC1768) || defined(TARGET_LPC2368) 00034 00035 if(value) { 00036 _gpio->FIOSET = _mask; 00037 } else { 00038 _gpio->FIOCLR = _mask; 00039 } 00040 00041 #elif defined(TARGET_LPC11U24) 00042 00043 if(value) { 00044 LPC_GPIO->SET[_index] = _mask; 00045 } else { 00046 LPC_GPIO->CLR[_index] = _mask; 00047 } 00048 #endif 00049 } 00050 00051 /** Return the output setting, represented as 0 or 1 (int) 00052 * 00053 * @returns 00054 * an integer representing the output setting of the pin if it is an output, 00055 * or read the input if set as an input 00056 */ 00057 int read() { 00058 #if defined(TARGET_LPC1768) || defined(TARGET_LPC2368) 00059 00060 return ((_gpio->FIOPIN & _mask) ? 1 : 0); 00061 #elif defined(TARGET_LPC11U24) 00062 return ((LPC_GPIO->PIN[_index] & _mask) ? 1 : 0); 00063 #endif 00064 } 00065 00066 00067 /** Set as an output 00068 */ 00069 void output(); 00070 00071 /** Set as an input 00072 */ 00073 void input(); 00074 00075 /** Set the input pin mode 00076 * 00077 * @param mode PullUp, PullDown, PullNone, OpenDrain 00078 */ 00079 void mode(PinMode pull); 00080 00081 #ifdef MBED_OPERATORS 00082 /** A shorthand for write() 00083 */ 00084 DigitalInOut& operator= (int value) { 00085 write(value); 00086 return *this; 00087 } 00088 00089 DigitalInOut& operator= (DigitalInOut& rhs) { 00090 write(rhs.read()); 00091 return *this; 00092 } 00093 00094 /** A shorthand for read() 00095 */ 00096 operator int() { 00097 return read(); 00098 } 00099 #endif 00100 00101 #ifdef MBED_RPC 00102 virtual const struct rpc_method *get_rpc_methods(); 00103 static struct rpc_class *get_rpc_class(); 00104 #endif 00105 00106 protected: 00107 00108 PinName _pin; 00109 00110 #if defined(TARGET_LPC1768) || defined(TARGET_LPC2368) 00111 LPC_GPIO_TypeDef *_gpio; 00112 #elif defined(TARGET_LPC11U24) 00113 int _index; 00114 #endif 00115 00116 uint32_t _mask; 00117 00118 }; 00119 00120 } // namespace mbed 00121 00122 #endif
Generated on Tue Jul 12 2022 11:27:26 by
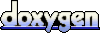