Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
BusIn.h
00001 /* mbed Microcontroller Library - DigitalIn 00002 * Copyright (c) 2007-2009 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_BUSIN_H 00006 #define MBED_BUSIN_H 00007 00008 #include "platform.h" 00009 #include "PinNames.h" 00010 #include "PeripheralNames.h" 00011 #include "Base.h" 00012 #include "DigitalIn.h" 00013 00014 namespace mbed { 00015 00016 /** A digital input bus, used for reading the state of a collection of pins 00017 */ 00018 class BusIn : public Base { 00019 00020 public: 00021 00022 /** Create an BusIn, connected to the specified pins 00023 * 00024 * @param <n> DigitalIn pin to connect to bus bit <n> (p5-p30, NC) 00025 * 00026 * @note 00027 * It is only required to specify as many pin variables as is required 00028 * for the bus; the rest will default to NC (not connected) 00029 */ 00030 BusIn(PinName p0, PinName p1 = NC, PinName p2 = NC, PinName p3 = NC, 00031 PinName p4 = NC, PinName p5 = NC, PinName p6 = NC, PinName p7 = NC, 00032 PinName p8 = NC, PinName p9 = NC, PinName p10 = NC, PinName p11 = NC, 00033 PinName p12 = NC, PinName p13 = NC, PinName p14 = NC, PinName p15 = NC, 00034 const char *name = NULL); 00035 00036 BusIn(PinName pins[16], const char *name = NULL); 00037 00038 virtual ~BusIn(); 00039 00040 /** Read the value of the input bus 00041 * 00042 * @returns 00043 * An integer with each bit corresponding to the value read from the associated DigitalIn pin 00044 */ 00045 int read(); 00046 00047 #ifdef MBED_OPERATORS 00048 /** A shorthand for read() 00049 */ 00050 operator int(); 00051 #endif 00052 00053 #ifdef MBED_RPC 00054 virtual const struct rpc_method *get_rpc_methods(); 00055 static struct rpc_class *get_rpc_class(); 00056 #endif 00057 00058 protected: 00059 00060 DigitalIn* _pin[16]; 00061 00062 #ifdef MBED_RPC 00063 static void construct(const char *arguments, char *res); 00064 #endif 00065 00066 }; 00067 00068 } // namespace mbed 00069 00070 #endif 00071
Generated on Tue Jul 12 2022 11:27:25 by
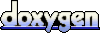