Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
AnalogOut.h
00001 /* mbed Microcontroller Library - AnalogOut 00002 * Copyright (c) 2006-2011 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_ANALOGOUT_H 00006 #define MBED_ANALOGOUT_H 00007 00008 #include "device.h" 00009 00010 #if DEVICE_ANALOGOUT 00011 00012 #include "platform.h" 00013 #include "PinNames.h" 00014 #include "PeripheralNames.h" 00015 #include "Base.h" 00016 00017 namespace mbed { 00018 00019 /** An analog output, used for setting the voltage on a pin 00020 * 00021 * Example: 00022 * @code 00023 * // Make a sawtooth output 00024 * 00025 * #include "mbed.h" 00026 * 00027 * AnalogOut tri(p18); 00028 * int main() { 00029 * while(1) { 00030 * tri = tri + 0.01; 00031 * wait_us(1); 00032 * if(tri == 1) { 00033 * tri = 0; 00034 * } 00035 * } 00036 * } 00037 * @endcode 00038 */ 00039 class AnalogOut : public Base { 00040 00041 public: 00042 00043 /** Create an AnalogOut connected to the specified pin 00044 * 00045 * @param AnalogOut pin to connect to (18) 00046 */ 00047 AnalogOut(PinName pin, const char *name = NULL); 00048 00049 /** Set the output voltage, specified as a percentage (float) 00050 * 00051 * @param value A floating-point value representing the output voltage, 00052 * specified as a percentage. The value should lie between 00053 * 0.0f (representing 0v / 0%) and 1.0f (representing 3.3v / 100%). 00054 * Values outside this range will be saturated to 0.0f or 1.0f. 00055 */ 00056 void write(float value); 00057 00058 /** Set the output voltage, represented as an unsigned short in the range [0x0, 0xFFFF] 00059 * 00060 * @param value 16-bit unsigned short representing the output voltage, 00061 * normalised to a 16-bit value (0x0000 = 0v, 0xFFFF = 3.3v) 00062 */ 00063 void write_u16(unsigned short value); 00064 00065 /** Return the current output voltage setting, measured as a percentage (float) 00066 * 00067 * @returns 00068 * A floating-point value representing the current voltage being output on the pin, 00069 * measured as a percentage. The returned value will lie between 00070 * 0.0f (representing 0v / 0%) and 1.0f (representing 3.3v / 100%). 00071 * 00072 * @note 00073 * This value may not match exactly the value set by a previous write(). 00074 */ 00075 float read(); 00076 00077 00078 #ifdef MBED_OPERATORS 00079 /** An operator shorthand for write() 00080 */ 00081 AnalogOut& operator= (float percent); 00082 AnalogOut& operator= (AnalogOut& rhs); 00083 00084 /** An operator shorthand for read() 00085 */ 00086 operator float(); 00087 #endif 00088 00089 #ifdef MBED_RPC 00090 virtual const struct rpc_method *get_rpc_methods(); 00091 static struct rpc_class *get_rpc_class(); 00092 #endif 00093 00094 protected: 00095 00096 DACName _dac; 00097 00098 }; 00099 00100 } // namespace mbed 00101 00102 #endif 00103 00104 #endif
Generated on Tue Jul 12 2022 11:27:25 by
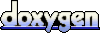