Fork of the official mbed C/C++ SDK provides the software platform and libraries to build your applications. The fork has the documentation converted to Doxygen format
Dependents: NervousPuppySprintOne NervousPuppySprint2602 Robot WarehouseBot1 ... more
Fork of mbed by
AnalogIn.h
00001 /* mbed Microcontroller Library - AnalogIn 00002 * Copyright (c) 2006-2011 ARM Limited. All rights reserved. 00003 */ 00004 00005 #ifndef MBED_ANALOGIN_H 00006 #define MBED_ANALOGIN_H 00007 00008 #include "device.h" 00009 00010 #if DEVICE_ANALOGIN 00011 00012 #include "platform.h" 00013 #include "PinNames.h" 00014 #include "PeripheralNames.h" 00015 #include "Base.h" 00016 00017 namespace mbed { 00018 00019 /** An analog input, used for reading the voltage on a pin 00020 * 00021 * Example: 00022 * @code 00023 * // Print messages when the AnalogIn is greater than 50% 00024 * 00025 * #include "mbed.h" 00026 * 00027 * AnalogIn temperature(p20); 00028 * 00029 * int main() { 00030 * while(1) { 00031 * if(temperature > 0.5) { 00032 * printf("Too hot! (%f)", temperature.read()); 00033 * } 00034 * } 00035 * } 00036 * @endcode 00037 */ 00038 class AnalogIn : public Base { 00039 00040 public: 00041 00042 /** Create an AnalogIn, connected to the specified pin 00043 * 00044 * @param pin AnalogIn pin to connect to 00045 * @param name (optional) A string to identify the object 00046 */ 00047 AnalogIn(PinName pin, const char *name = NULL); 00048 00049 /** Read the input voltage, represented as a float in the range [0.0, 1.0] 00050 * 00051 * @returns A floating-point value representing the current input voltage, measured as a percentage 00052 */ 00053 float read(); 00054 00055 /** Read the input voltage, represented as an unsigned short in the range [0x0, 0xFFFF] 00056 * 00057 * @returns 00058 * 16-bit unsigned short representing the current input voltage, normalised to a 16-bit value 00059 */ 00060 unsigned short read_u16(); 00061 00062 #ifdef MBED_OPERATORS 00063 /** An operator shorthand for read() 00064 * 00065 * The float() operator can be used as a shorthand for read() to simplify common code sequences 00066 * 00067 * Example: 00068 * @code 00069 * float x = volume.read(); 00070 * float x = volume; 00071 * 00072 * if(volume.read() > 0.25) { ... } 00073 * if(volume > 0.25) { ... } 00074 * @endcode 00075 */ 00076 operator float(); 00077 #endif 00078 00079 #ifdef MBED_RPC 00080 virtual const struct rpc_method *get_rpc_methods(); 00081 static struct rpc_class *get_rpc_class(); 00082 #endif 00083 00084 protected: 00085 00086 ADCName _adc; 00087 00088 }; 00089 00090 } // namespace mbed 00091 00092 #endif 00093 00094 #endif
Generated on Tue Jul 12 2022 11:27:25 by
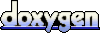