My fork of the HTTPServer (working)
Embed:
(wiki syntax)
Show/hide line numbers
mem.h
00001 /* 00002 * Copyright (c) 2001-2004 Swedish Institute of Computer Science. 00003 * All rights reserved. 00004 * 00005 * Redistribution and use in source and binary forms, with or without modification, 00006 * are permitted provided that the following conditions are met: 00007 * 00008 * 1. Redistributions of source code must retain the above copyright notice, 00009 * this list of conditions and the following disclaimer. 00010 * 2. Redistributions in binary form must reproduce the above copyright notice, 00011 * this list of conditions and the following disclaimer in the documentation 00012 * and/or other materials provided with the distribution. 00013 * 3. The name of the author may not be used to endorse or promote products 00014 * derived from this software without specific prior written permission. 00015 * 00016 * THIS SOFTWARE IS PROVIDED BY THE AUTHOR ``AS IS'' AND ANY EXPRESS OR IMPLIED 00017 * WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF 00018 * MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT 00019 * SHALL THE AUTHOR BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, 00020 * EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT 00021 * OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS 00022 * INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN 00023 * CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING 00024 * IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY 00025 * OF SUCH DAMAGE. 00026 * 00027 * This file is part of the lwIP TCP/IP stack. 00028 * 00029 * Author: Adam Dunkels <adam@sics.se> 00030 * 00031 */ 00032 #ifndef __LWIP_MEM_H__ 00033 #define __LWIP_MEM_H__ 00034 00035 #include "lwip/opt.h" 00036 00037 #ifdef __cplusplus 00038 extern "C" { 00039 #endif 00040 00041 #if MEM_LIBC_MALLOC 00042 00043 #include <stddef.h> /* for size_t */ 00044 00045 typedef size_t mem_size_t; 00046 00047 /* aliases for C library malloc() */ 00048 #define mem_init() 00049 /* in case C library malloc() needs extra protection, 00050 * allow these defines to be overridden. 00051 */ 00052 #ifndef mem_free 00053 #define mem_free free 00054 #endif 00055 #ifndef mem_malloc 00056 #define mem_malloc malloc 00057 #endif 00058 #ifndef mem_calloc 00059 #define mem_calloc calloc 00060 #endif 00061 #ifndef mem_realloc 00062 #define mem_realloc realloc 00063 #endif 00064 #else /* MEM_LIBC_MALLOC */ 00065 00066 /* MEM_SIZE would have to be aligned, but using 64000 here instead of 00067 * 65535 leaves some room for alignment... 00068 */ 00069 #if MEM_SIZE > 64000l 00070 typedef u32_t mem_size_t; 00071 #else 00072 typedef u16_t mem_size_t; 00073 #endif /* MEM_SIZE > 64000 */ 00074 00075 #if MEM_USE_POOLS 00076 /** mem_init is not used when using pools instead of a heap */ 00077 #define mem_init() 00078 /** mem_realloc is not used when using pools instead of a heap: 00079 we can't free part of a pool element and don't want to copy the rest */ 00080 #define mem_realloc(mem, size) (mem) 00081 #else /* MEM_USE_POOLS */ 00082 /* lwIP alternative malloc */ 00083 void mem_init(void); 00084 void *mem_realloc(void *mem, mem_size_t size); 00085 #endif /* MEM_USE_POOLS */ 00086 void *mem_malloc(mem_size_t size); 00087 void *mem_calloc(mem_size_t count, mem_size_t size); 00088 void mem_free(void *mem); 00089 #endif /* MEM_LIBC_MALLOC */ 00090 00091 #ifndef LWIP_MEM_ALIGN_SIZE 00092 #define LWIP_MEM_ALIGN_SIZE(size) (((size) + MEM_ALIGNMENT - 1) & ~(MEM_ALIGNMENT-1)) 00093 #endif 00094 00095 #ifndef LWIP_MEM_ALIGN // v-- XXX: was void * 00096 #define LWIP_MEM_ALIGN(addr) ((u8_t *)(((mem_ptr_t)(addr) + MEM_ALIGNMENT - 1) & ~(mem_ptr_t)(MEM_ALIGNMENT-1))) 00097 #endif 00098 00099 #ifdef __cplusplus 00100 } 00101 #endif 00102 00103 #endif /* __LWIP_MEM_H__ */
Generated on Tue Jul 12 2022 19:24:05 by
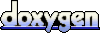