My fork of the HTTPServer (working)
Embed:
(wiki syntax)
Show/hide line numbers
HTTPStaticPage.h
00001 #ifndef HTTPSTATICPAGE_H 00002 #define HTTPSTATICPAGE_H 00003 00004 #include "HTTPServer.h" 00005 00006 /** 00007 * A datastorage helper for the HTTPStaticPage class. 00008 * Stores only the left upload size. 00009 */ 00010 class HTTPStaticPageData : public HTTPData { 00011 public: int left; 00012 }; 00013 00014 /** 00015 * This class Provide a Handler to send Static HTTP Pages from the bin file. 00016 */ 00017 class HTTPStaticPage : public HTTPHandler { 00018 public: 00019 /** 00020 * Constructer takes the pagename and the pagedata. 00021 * As long as the pagedata is NULL terminated you have not to tell the data length. 00022 * But if you want to send binary data you should tell us the size. 00023 */ 00024 HTTPStaticPage(const char *path, const char *page, int length = 0) 00025 : HTTPHandler(path), _page(page) { 00026 _size = (length)?length:strlen(_page); 00027 } 00028 00029 private: 00030 /** 00031 * A this Static Page is requested! 00032 * Prepare a datastorage helper "HTTPStaticPageHelper" to store the left data size. 00033 * And return HTTP_OK 00034 */ 00035 virtual HTTPStatus init(HTTPConnection *con) const { 00036 HTTPStaticPageData *data = new HTTPStaticPageData(); 00037 con->data = data; 00038 data->left = _size; 00039 con->setLength(_size); 00040 return HTTP_OK; 00041 } 00042 00043 /** 00044 * Send the maximum data out to the client. 00045 * If the file is complete transmitted close connection by returning HTTP_SuccessEnded 00046 */ 00047 virtual HTTPHandle send(HTTPConnection *con, int maximum) const { 00048 HTTPStaticPageData *data = static_cast<HTTPStaticPageData *>(con->data); 00049 int len = min(maximum, data->left); 00050 err_t err; 00051 00052 do { 00053 err = con->write((void*)&_page[_size - data->left], len, 1); 00054 if(err == ERR_MEM) { 00055 len >>= 1; 00056 } 00057 } while(err == ERR_MEM && len > 1); 00058 if(err == ERR_OK) { 00059 data->left -= len; 00060 } 00061 00062 return (data->left)? HTTP_Success : HTTP_SuccessEnded; 00063 } 00064 00065 /** Pointer to the page data */ 00066 const char *_page; 00067 00068 /** page data size*/ 00069 int _size; 00070 }; 00071 00072 #endif
Generated on Tue Jul 12 2022 19:24:05 by
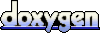