
NMHU example for light sensor SSD 341
Fork of lightsense_kl46z_states by
main.cpp
00001 #include "mbed.h" 00002 #include "SLCD.h" 00003 00004 00005 // An State Machine solution to HW 4.1 Q3 00006 // using wait() for high and low limits. 00007 //#define PRINTDEBUG 00008 #define PROGNAME "blink_kl46z_states nowait v1\n\r" 00009 #define LEDON false 00010 #define LEDOFF true 00011 #define LEDTMESS "TRUE" 00012 #define LEDFMESS "FALS" 00013 #define BLINKDWELL 400 // milliseconds 00014 #define LIMITSTAYON 1000 00015 #define DFDELTA 0.01 00016 #define PWMTIME 1 // ms (kHz 00017 #define LCDLEN 10 00018 #define NUMSTATES 2 00019 #define LOWLIMIT 0.1 00020 #define HILIMIT 0.85 00021 #define ONTIME 1.0 00022 00023 00024 enum blinkStates {IDLESTATE, NEWBLINK, LOWLITE, HILITE}; // define the states 00025 00026 char logicalString [NUMSTATES][LCDLEN] = {LEDFMESS, LEDTMESS}; 00027 SLCD slcd; //define LCD display globally define 00028 Serial pc(USBTX, USBRX); 00029 00030 void LCDMess(char *lMess){ 00031 slcd.Home(); 00032 slcd.clear(); 00033 slcd.printf(lMess); 00034 } 00035 00036 int main() { 00037 DigitalOut greenColor(LED_GREEN); 00038 DigitalOut redColor(LED_RED); 00039 AnalogIn LightSensor(PTE22); 00040 float lightData; 00041 char lcdData[LCDLEN]; 00042 Timer LEDTimer; // time till next PWM values is to change. 00043 blinkStates PGMState = IDLESTATE; // work till timer transitions 00044 int ledState = LEDON; 00045 00046 00047 int timeToChangeDF = BLINKDWELL; 00048 // set up timer for next step of Duty Factor timing 00049 LEDTimer.start(); 00050 LEDTimer.reset(); 00051 pc.printf(PROGNAME); 00052 00053 00054 00055 while(true) { 00056 switch (PGMState){ 00057 case IDLESTATE: { 00058 if (LEDTimer.read_ms() > timeToChangeDF){ // check for timer time out transtion 00059 lightData = (1.0 - LightSensor.read()); 00060 sprintf(lcdData,"%4.3f",lightData); 00061 LCDMess(lcdData); 00062 timeToChangeDF = BLINKDWELL; 00063 if (lightData < LOWLIMIT){ // find appropriate state 00064 PGMState = LOWLITE; 00065 timeToChangeDF = LIMITSTAYON; 00066 } else if (lightData > HILIMIT){ 00067 PGMState = HILITE; 00068 timeToChangeDF = LIMITSTAYON; 00069 } else { 00070 PGMState = NEWBLINK; 00071 timeToChangeDF = BLINKDWELL; 00072 } 00073 00074 } 00075 break; 00076 } 00077 case NEWBLINK: { 00078 ledState = !ledState; 00079 redColor.write(ledState); 00080 greenColor.write(!ledState); 00081 // create string for display on LCD 00082 00083 LEDTimer.reset(); // reset the timer 00084 PGMState = IDLESTATE; // go idle state 00085 break; 00086 } 00087 case HILITE: { 00088 redColor.write(LEDON); 00089 greenColor.write(LEDOFF); 00090 // create string for display on LCD 00091 if (LEDTimer.read_ms() > timeToChangeDF){ 00092 LEDTimer.reset(); // reset the timer 00093 PGMState = IDLESTATE; // go idle state 00094 } 00095 break; 00096 } 00097 case LOWLITE: { 00098 redColor.write(LEDOFF); 00099 greenColor.write(LEDON); 00100 // create string for display on LCD 00101 if (LEDTimer.read_ms() > timeToChangeDF){ 00102 LEDTimer.reset(); // reset the timer 00103 PGMState = IDLESTATE; // go idle state 00104 } 00105 break; 00106 } 00107 } // end state machine 00108 00109 #ifdef PRINTDEBUG 00110 pc.printf("i= %d dutyfactor = %5.4f workingDelta %5.4f \n\r", i, dutyFactor, workingDelta); 00111 #endif 00112 }// emd while 00113 }
Generated on Mon Jul 25 2022 12:03:29 by
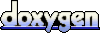