
Stop watch to demo mod buttons and timer class.
Fork of kl46z_slider_v1 by
main.cpp
00001 #include "mbed.h" 00002 #include <math.h> 00003 #include "SLCD.h" 00004 00005 #define LEDON false 00006 #define LEDOFF true 00007 #define NUMBUTS 2 00008 #define LBUT PTC12 // port addresses for buttons 00009 #define RBUT PTC3 00010 #define STOPPEDSTATE 0 00011 #define TIMINGSTATE 1 00012 #define RESETTINGSTATE 0 00013 #define PRINTDELTA 0.01 00014 #define LCDCHARLEN 10 00015 #define BUTTONTIME 0.2 00016 #define FULLMINUTE 60 //seconds 00017 #define PROGNAME "kl46z_stop_watch_v1\n\r" 00018 #define LCDTITLE "STPW" 00019 #define TITLEWAIT 2.0 00020 00021 //#define PRINTDBUG // uncomment if printing to serial port desired 00022 00023 SLCD slcd; //define LCD display 00024 Serial pc(USBTX, USBRX); 00025 00026 Timer ButtonTimer; // for reading button states 00027 DigitalIn buttons[NUMBUTS] = {RBUT, LBUT}; 00028 int displayState = STOPPEDSTATE; 00029 00030 00031 00032 void initialize_global_vars(){ 00033 pc.printf(PROGNAME); 00034 // set up DAQ timer 00035 // set up DAQ timers 00036 ButtonTimer.start(); 00037 ButtonTimer.reset(); 00038 } 00039 00040 void LCDMess(char *lMess){ 00041 slcd.Home(); 00042 slcd.clear(); 00043 slcd.printf(lMess); 00044 } 00045 void showTitle(){ 00046 LCDMess(LCDTITLE); 00047 wait(TITLEWAIT); 00048 return; 00049 } 00050 int main(void) { 00051 int i; 00052 char lcdData[LCDCHARLEN]; 00053 PwmOut gled(LED_GREEN); 00054 PwmOut rled(LED_RED); 00055 pc.printf(PROGNAME); 00056 float secondsCount = 0.0; 00057 int minutesCount; // for displaying mininuts 00058 int seconds; // 00059 int fifthSeconds; 00060 bool statetoggle = false; //was in stopped state. 00061 00062 initialize_global_vars(); 00063 showTitle(); 00064 00065 while (true) { 00066 if (ButtonTimer > BUTTONTIME){ 00067 for (i=0; i<NUMBUTS; i++){ // index will be 0 or 1 00068 if(!buttons[i]) { // a buttton is pressed 00069 displayState = i; 00070 switch (displayState){ // this keeps things generic 00071 case TIMINGSTATE: { 00072 statetoggle = !statetoggle; 00073 break; 00074 } 00075 case RESETTINGSTATE :{ 00076 break; 00077 } 00078 } 00079 } // if ! buttons 00080 }// for loop to look at buttons 00081 ButtonTimer.reset(); 00082 switch (displayState){ 00083 /* this goes away 00084 case STOPPEDSTATE : { 00085 rled = 0.0; 00086 gled = 1.0; 00087 break; 00088 } 00089 */ 00090 case RESETTINGSTATE: 00091 if (!statetoggle){ 00092 secondsCount = 0; 00093 displayState = TIMINGSTATE; 00094 } 00095 rled = 0.0; 00096 gled = 1.0; 00097 break; 00098 00099 00100 case TIMINGSTATE : { 00101 if(statetoggle){ 00102 secondsCount = secondsCount + BUTTONTIME; 00103 } 00104 rled = 1.0; 00105 gled = 0.0; 00106 break; 00107 } 00108 } 00109 00110 // Parse the seconds 00111 seconds = (int)secondsCount; // make seconds "mask" 00112 fifthSeconds = (int)((secondsCount - (float)seconds) * 10); // the 0.2 seconds 00113 minutesCount = seconds / FULLMINUTE; 00114 seconds = seconds % FULLMINUTE; 00115 sprintf (lcdData,"%1d.%02d.%1d",minutesCount,seconds,fifthSeconds); 00116 LCDMess(lcdData); 00117 }// end Button timer 00118 } 00119 }
Generated on Thu Jul 14 2022 19:41:25 by
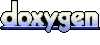