window
Fork of httpServer by
Embed:
(wiki syntax)
Show/hide line numbers
FsHandler.cpp
00001 /* FsHandler.cpp */ 00002 #include "mbed.h" 00003 #include "FsHandler.h" 00004 //#define DEBUG 00005 #include "hl_debug.h" 00006 00007 00008 00009 DigitalIn din(PC_14); 00010 int mode2 = 0; 00011 00012 static int matchstrings(const char* one, const char* two) 00013 { 00014 int m = 0; 00015 00016 for (m = 0; m < min(strlen(one), strlen(two)) ; m++) { 00017 if (one[m] != two[m]) 00018 return m; 00019 } 00020 return m; 00021 } 00022 00023 std::map<const char*, const char*> HTTPFsRequestHandler::m_fsMap; 00024 00025 HTTPFsRequestHandler::HTTPFsRequestHandler(const char* rootPath, const char* localPath, HTTPConnection::HTTPMessage& Msg, TCPSocketConnection& Tcp) 00026 : HTTPRequestHandler(Msg, Tcp) 00027 { 00028 m_rootPath = rootPath; 00029 m_localPath = localPath; 00030 00031 string myPath = m_rootPath + m_localPath; 00032 00033 // Now replace the virtual root path with a mounted device path 00034 std::map<const char*, const char*>::iterator it; 00035 const char *bestMatch = NULL; 00036 const char *bestMatchExchange = NULL; 00037 int match_ind = -1; 00038 for (it = m_fsMap.begin() ; it != m_fsMap.end() ; it++) { 00039 // find best match (if the given logical path is containted in the root 00040 int s = matchstrings(myPath.c_str(), it->second); 00041 INFO("Matching Root %s with handler %s results in %d identical characters\n", myPath.c_str(), it->second, s); 00042 if ((s == strlen(it->second)) && (s > match_ind)) { 00043 match_ind = s; 00044 bestMatch = it->first; 00045 bestMatchExchange = it->second; 00046 } 00047 } 00048 00049 if (bestMatch != NULL) { 00050 m_rootPath = bestMatch; 00051 m_localPath = string(myPath).substr(strlen(bestMatchExchange)); 00052 } 00053 00054 handleRequest(); 00055 } 00056 00057 HTTPFsRequestHandler::~HTTPFsRequestHandler() 00058 { 00059 } 00060 00061 int HTTPFsRequestHandler::handleGetRequest() 00062 { 00063 HTTPHeaders headers; 00064 int retval = 0; //success 00065 uint8_t pin_state; 00066 00067 if( std::string::npos != msg.uri.find("get_dio14.cgi") ) 00068 { 00069 if(din) 00070 pin_state = 1; 00071 else 00072 pin_state = 0; 00073 00074 /* 00075 *len = sprintf((char *)buf, "DioCallback({\"dio_p\":\"14\",\ 00076 \"dio_s\":\"%d\"\ 00077 });", 00078 pin_state // Digital io status 00079 ); 00080 00081 00082 Tcp. 00083 */ 00084 } 00085 else //read html pages 00086 { 00087 if (m_localPath.length() > 4) 00088 getStandardHeaders(headers, m_localPath.substr(m_localPath.length()-4).c_str()); 00089 else 00090 getStandardHeaders(headers); 00091 00092 INFO("Handling Get Request (root = %s, local = %s).", m_rootPath.c_str(), m_localPath.c_str()); 00093 00094 std::string reqPath; 00095 00096 // Check if we received a directory with the local bath 00097 if ((m_localPath.length() == 0) || (m_localPath.substr( m_localPath.length()-1, 1) == "/")) { 00098 // yes, we shall append the default page name 00099 m_localPath += "dio_page.html"; 00100 } 00101 00102 reqPath = m_rootPath + m_localPath; 00103 00104 INFO("Mapping \"%s\" to \"%s\"", msg.uri.c_str(), reqPath.c_str()); 00105 00106 FILE *fp = fopen(reqPath.c_str(), "r"); 00107 if (fp != NULL) { 00108 char * pBuffer = NULL; 00109 int sz = 8192; 00110 while( pBuffer == NULL) { 00111 sz /= 2; 00112 pBuffer = (char*)malloc(sz); 00113 if (sz < 128) 00114 error ("OutOfMemory"); 00115 } 00116 00117 // File was found and can be returned 00118 00119 // first determine the size 00120 fseek(fp, 0, SEEK_END); 00121 long size = ftell(fp); 00122 fseek(fp, 0, SEEK_SET); 00123 00124 startResponse(200, size); 00125 while(!feof(fp) && !ferror(fp)) { 00126 int cnt = fread(pBuffer, 1, sz , fp); 00127 if (cnt < 0) 00128 cnt = 0; 00129 processResponse(cnt, pBuffer); 00130 } 00131 delete pBuffer; 00132 endResponse(); 00133 fclose(fp); 00134 } 00135 else { 00136 retval = 404; 00137 ERR("Requested file was not found !"); 00138 } 00139 } 00140 00141 return retval; 00142 } 00143 00144 int HTTPFsRequestHandler::handlePostRequest() 00145 { 00146 00147 int pin = 0; 00148 00149 if( std::string::npos != msg.uri.find("set_dio.cgi") ) 00150 { 00151 pin = get_http_param_value("pin"); 00152 00153 00154 if(pin == 9) 00155 { 00156 if(get_http_param_value("val") == 0){ 00157 DigitalOut(D9,0); 00158 DigitalOut(D8,1); 00159 wait(0.87); 00160 DigitalOut(D9,0); 00161 DigitalOut(D8,0); 00162 }// opened status 00163 else{ 00164 DigitalOut(D9,1); 00165 DigitalOut(D8,0); 00166 wait(0.8); 00167 DigitalOut(D9,0); 00168 DigitalOut(D8,0); 00169 //closed status 00170 } 00171 00172 } 00173 else if(pin == 10) 00174 { 00175 if(get_http_param_value("val") == 0){ 00176 DigitalOut(D13,1); 00177 }// turn on 00178 else{ 00179 DigitalOut(D13,0); 00180 00181 }//turn off 00182 00183 } 00184 00185 else 00186 { 00187 WARN("Wrong pin number"); 00188 } 00189 00190 return 0; 00191 } 00192 else 00193 { 00194 return 404; 00195 } 00196 } 00197 00198 int HTTPFsRequestHandler::handlePutRequest() 00199 { 00200 return 404; 00201 } 00202 00203 uint32_t HTTPFsRequestHandler::get_http_param_value(char* param_name) 00204 { 00205 uint8_t * name = 0; 00206 uint8_t * pos2; 00207 uint16_t len = 0; 00208 char value[10]; 00209 uint32_t ret = 0; 00210 00211 //msg.attri 00212 if((name = (uint8_t *)strstr(msg.attri, param_name))) 00213 { 00214 name += strlen(param_name) + 1; 00215 pos2 = (uint8_t*)strstr((char*)name, "&"); 00216 if(!pos2) 00217 { 00218 pos2 = name + strlen((char*)name); 00219 } 00220 len = pos2 - name; 00221 00222 if(len) 00223 { 00224 strncpy(value, (char*)name, len); 00225 ret = atoi(value); 00226 } 00227 } 00228 return ret; 00229 } 00230
Generated on Mon Jul 18 2022 01:17:42 by
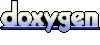