
smoothie port to mbed online compiler (smoothieware.org)
Embed:
(wiki syntax)
Show/hide line numbers
TemperatureControl.h
00001 /* 00002 this file is part of smoothie (http://smoothieware.org/). the motion control part is heavily based on grbl (https://github.com/simen/grbl). 00003 smoothie is free software: you can redistribute it and/or modify it under the terms of the gnu general public license as published by the free software foundation, either version 3 of the license, or (at your option) any later version. 00004 smoothie is distributed in the hope that it will be useful, but without any warranty; without even the implied warranty of merchantability or fitness for a particular purpose. see the gnu general public license for more details. 00005 you should have received a copy of the gnu general public license along with smoothie. if not, see <http://www.gnu.org/licenses/>. 00006 */ 00007 00008 #ifndef temperaturecontrol_h 00009 #define temperaturecontrol_h 00010 00011 #include "libs/Pin.h" 00012 #include <math.h> 00013 00014 #define UNDEFINED -1 00015 00016 #define thermistor_checksum 41045 00017 #define r0_checksum 5538 00018 #define readings_per_second_checksum 18645 00019 #define t0_checksum 6564 00020 #define beta_checksum 1181 00021 #define vadc_checksum 10911 00022 #define vcc_checksum 36157 00023 #define r1_checksum 5795 00024 #define r2_checksum 6052 00025 #define temperature_control_checksum 44054 00026 #define thermistor_pin_checksum 1788 00027 #define heater_pin_checksum 35619 00028 00029 class TemperatureControl : public Module { 00030 public: 00031 TemperatureControl(); 00032 TemperatureControl(uint16_t name); 00033 00034 virtual void on_module_loaded(); 00035 virtual void on_main_loop(void* argument); 00036 virtual void on_gcode_execute(void* argument); 00037 virtual void on_config_reload(void* argument); 00038 void set_desired_temperature(double desired_temperature); 00039 double get_temperature(); 00040 double adc_value_to_temperature(double adc_value); 00041 double temperature_to_adc_value(double temperature); 00042 uint32_t thermistor_read_tick(uint32_t dummy); 00043 double new_thermistor_reading(); 00044 double average_adc_reading(); 00045 00046 double desired_adc_value; 00047 double tail_adc_value; 00048 double head_adc_value; 00049 00050 // Thermistor computation settings 00051 double r0; 00052 double t0; 00053 double r1; 00054 double r2; 00055 double beta; 00056 double vadc; 00057 double vcc; 00058 double k; 00059 double vs; 00060 double rs; 00061 00062 double acceleration_factor; 00063 double readings_per_second; 00064 00065 RingBuffer<double,16> queue; // Queue of Blocks 00066 int error_count; 00067 00068 uint16_t name_checksum; 00069 00070 Pin* thermistor_pin; 00071 Pin* heater_pin; 00072 00073 bool waiting; 00074 00075 }; 00076 00077 #endif
Generated on Tue Jul 12 2022 14:14:42 by
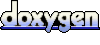