
smoothie port to mbed online compiler (smoothieware.org)
Embed:
(wiki syntax)
Show/hide line numbers
StepTicker.cpp
00001 /* 00002 This file is part of Smoothie (http://smoothieware.org/). The motion control part is heavily based on Grbl (https://github.com/simen/grbl). 00003 Smoothie is free software: you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation, either version 3 of the License, or (at your option) any later version. 00004 Smoothie is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details. 00005 You should have received a copy of the GNU General Public License along with Smoothie. If not, see <http://www.gnu.org/licenses/>. 00006 */ 00007 00008 00009 00010 using namespace std; 00011 #include <vector> 00012 #include "libs/nuts_bolts.h" 00013 #include "libs/Module.h" 00014 #include "libs/Kernel.h" 00015 #include "StepTicker.h" 00016 #include "system_LPC17xx.h" // mbed.h lib 00017 00018 00019 StepTicker* global_step_ticker; 00020 00021 StepTicker::StepTicker(){ 00022 global_step_ticker = this; 00023 LPC_TIM0->MR0 = 1000000; // Initial dummy value for Match Register 00024 LPC_TIM0->MCR = 11; // Match on MR0, reset on MR0, match on MR1 00025 LPC_TIM0->TCR = 1; // Enable interrupt 00026 NVIC_EnableIRQ(TIMER0_IRQn); // Enable interrupt handler 00027 } 00028 00029 void StepTicker::set_frequency( double frequency ){ 00030 this->frequency = frequency; 00031 LPC_TIM0->MR0 = int(floor((SystemCoreClock/4)/frequency)); // SystemCoreClock/4 = Timer increments in a second 00032 if( LPC_TIM0->TC > LPC_TIM0->MR0 ){ 00033 LPC_TIM0->TCR = 3; // Reset 00034 LPC_TIM0->TCR = 1; // Reset 00035 } 00036 } 00037 00038 void StepTicker::set_reset_delay( double seconds ){ 00039 LPC_TIM0->MR1 = int(floor(double(SystemCoreClock/4)*( seconds ))); // SystemCoreClock/4 = Timer increments in a second 00040 } 00041 00042 void StepTicker::tick(){ 00043 for (int i=0; i<this->hooks.size(); i++){ 00044 this->hooks.at(i)->call(); 00045 } 00046 } 00047 00048 void StepTicker::reset_tick(){ 00049 for (int i=0; i<this->reset_hooks.size(); i++){ 00050 this->reset_hooks.at(i)->call(); 00051 } 00052 } 00053 00054 extern "C" void TIMER0_IRQHandler (void){ 00055 if((LPC_TIM0->IR >> 0) & 1){ // If interrupt register set for MR0 00056 LPC_TIM0->IR |= 1 << 0; // Reset it 00057 global_step_ticker->tick(); 00058 } 00059 if((LPC_TIM0->IR >> 1) & 1){ // If interrupt register set for MR1 00060 LPC_TIM0->IR |= 1 << 1; // Reset it 00061 global_step_ticker->reset_tick(); 00062 } 00063 } 00064 00065
Generated on Tue Jul 12 2022 14:14:42 by
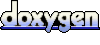