
smoothie port to mbed online compiler (smoothieware.org)
Embed:
(wiki syntax)
Show/hide line numbers
Pin.h
00001 #ifndef PIN_H 00002 #define PIN_H 00003 00004 #include <stdlib.h> 00005 #include "mbed.h" // smoothed mbed.h lib 00006 #include "libs/Kernel.h" 00007 #include "libs/utils.h" 00008 #include <string> 00009 00010 class Pin{ 00011 public: 00012 Pin(){ } 00013 00014 Pin* from_string(std::string value){ 00015 LPC_GPIO_TypeDef* gpios[5] ={LPC_GPIO0,LPC_GPIO1,LPC_GPIO2,LPC_GPIO3,LPC_GPIO4}; 00016 this->port_number = atoi(value.substr(0,1).c_str()); 00017 this->port = gpios[this->port_number]; 00018 this->inverting = ( value.find_first_of("!")!=string::npos ? true : false ); 00019 this->pin = atoi( value.substr(2, value.size()-2-(this->inverting?1:0)).c_str() ); 00020 return this; 00021 } 00022 00023 inline Pin* as_output(){ 00024 this->port->FIODIR |= 1<<this->pin; 00025 return this; 00026 } 00027 00028 inline Pin* as_input(){ 00029 this->port->FIODIR &= ~(1<<this->pin); 00030 return this; 00031 } 00032 00033 inline Pin* as_open_drain(){ 00034 if( this->port_number == 0 ){ LPC_PINCON->PINMODE_OD0 |= (1<<this->pin); } 00035 if( this->port_number == 1 ){ LPC_PINCON->PINMODE_OD1 |= (1<<this->pin); } 00036 if( this->port_number == 2 ){ LPC_PINCON->PINMODE_OD2 |= (1<<this->pin); } 00037 if( this->port_number == 3 ){ LPC_PINCON->PINMODE_OD3 |= (1<<this->pin); } 00038 if( this->port_number == 4 ){ LPC_PINCON->PINMODE_OD4 |= (1<<this->pin); } 00039 return this; 00040 } 00041 00042 inline bool get(){ 00043 return this->inverting ^ (( this->port->FIOPIN >> this->pin ) & 1); 00044 } 00045 00046 inline void set(bool value){ 00047 value = this->inverting ^ value; 00048 if( value ){ 00049 this->port->FIOSET = 1 << this->pin; 00050 }else{ 00051 this->port->FIOCLR = 1 << this->pin; 00052 } 00053 } 00054 00055 bool inverting; 00056 LPC_GPIO_TypeDef* port; 00057 char port_number; 00058 char pin; 00059 }; 00060 00061 00062 00063 00064 #endif
Generated on Tue Jul 12 2022 14:14:41 by
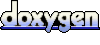