
smoothie port to mbed online compiler (smoothieware.org)
Embed:
(wiki syntax)
Show/hide line numbers
Laser.cpp
00001 /* 00002 This file is part of Smoothie (http://smoothieware.org/). The motion control part is heavily based on Grbl (https://github.com/simen/grbl). 00003 Smoothie is free software: you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation, either version 3 of the License, or (at your option) any later version. 00004 Smoothie is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details. 00005 You should have received a copy of the GNU General Public License along with Smoothie. If not, see <http://www.gnu.org/licenses/>. 00006 */ 00007 00008 #include "libs/Module.h" 00009 #include "libs/Kernel.h" 00010 #include "modules/communication/utils/Gcode.h" 00011 #include "modules/robot/Stepper.h" 00012 #include "Laser.h" 00013 #include "libs/nuts_bolts.h" 00014 00015 Laser::Laser(PinName pin) : laser_pin(pin){ 00016 this->laser_pin.period_us(10); 00017 } 00018 00019 void Laser::on_module_loaded() { 00020 if( !this->kernel->config->value( laser_module_enable_checksum )->by_default(false)->as_bool() ){ return; } 00021 this->register_for_event(ON_GCODE_EXECUTE); 00022 this->register_for_event(ON_SPEED_CHANGE); 00023 this->register_for_event(ON_PLAY); 00024 this->register_for_event(ON_PAUSE); 00025 this->register_for_event(ON_BLOCK_BEGIN); 00026 this->register_for_event(ON_BLOCK_END); 00027 } 00028 00029 // Turn laser off laser at the end of a move 00030 void Laser::on_block_end(void* argument){ 00031 this->laser_pin = 0; 00032 } 00033 00034 // Set laser power at the beginning of a block 00035 void Laser::on_block_begin(void* argument){ 00036 this->set_proportional_power(); 00037 } 00038 00039 // When the play/pause button is set to pause, or a module calls the ON_PAUSE event 00040 void Laser::on_pause(void* argument){ 00041 this->laser_pin = 0; 00042 } 00043 00044 // When the play/pause button is set to play, or a module calls the ON_PLAY event 00045 void Laser::on_play(void* argument){ 00046 this->set_proportional_power(); 00047 } 00048 00049 // Turn laser on/off depending on received GCodes 00050 void Laser::on_gcode_execute(void* argument){ 00051 Gcode* gcode = static_cast<Gcode*>(argument); 00052 this->laser_on = false; 00053 if( gcode->has_letter('G' )){ 00054 int code = gcode->get_value('G'); 00055 if( code == 0 ){ // G0 00056 this->laser_pin = 0; 00057 this->laser_on = false; 00058 }else if( code >= 1 && code <= 3 ){ // G1, G2, G3 00059 this->laser_on = true; 00060 } 00061 } 00062 } 00063 00064 // We follow the stepper module here, so speed must be proportional 00065 void Laser::on_speed_change(void* argument){ 00066 this->set_proportional_power(); 00067 } 00068 00069 void Laser::set_proportional_power(){ 00070 if( this->laser_on && this->kernel->stepper->current_block ){ 00071 this->laser_pin = double(this->kernel->stepper->trapezoid_adjusted_rate)/double(this->kernel->stepper->current_block->nominal_rate); 00072 } 00073 }
Generated on Tue Jul 12 2022 14:14:40 by
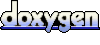