
smoothie port to mbed online compiler (smoothieware.org)
Embed:
(wiki syntax)
Show/hide line numbers
Digipot.h
00001 #ifndef DIGIPOT_H 00002 #define DIGIPOT_H 00003 00004 #include "libs/Kernel.h" 00005 #include "I2C.h" // mbed.h lib 00006 #include "libs/utils.h" 00007 #include <string> 00008 #include <math.h> 00009 00010 class Digipot{ 00011 public: 00012 Digipot(){ } 00013 00014 char current_to_wiper( double current ){ 00015 return char(ceil(double((113.33*current)))); 00016 } 00017 00018 void i2c_send( char first, char second, char third ){ 00019 this->i2c->start(); 00020 this->i2c->write(first); 00021 this->i2c->write(second); 00022 this->i2c->write(third); 00023 this->i2c->stop(); 00024 } 00025 00026 void set_current( int channel, double current ){ 00027 00028 current = min( max( current, 0.0L ), 2.0L ); 00029 00030 // I2C com 00031 this->i2c = new mbed::I2C(p9, p10); 00032 00033 // Initial setup 00034 this->i2c_send( 0x58, 0x40, 0xff ); 00035 this->i2c_send( 0x58, 0xA0, 0xff ); 00036 00037 // Set actual wiper value 00038 char adresses[4] = { 0x00, 0x10, 0x60, 0x70 }; 00039 this->i2c_send( 0x58, adresses[channel], this->current_to_wiper(current) ); 00040 00041 } 00042 00043 mbed::I2C* i2c; 00044 }; 00045 00046 00047 #endif
Generated on Tue Jul 12 2022 14:14:40 by
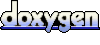