
An mbed implementation of IEC 61850-9-2LE Sample Values. Creating using the rapid61850 library, available at: https://github.com/stevenblair/rapid61850.
svPacketData.h
00001 /** 00002 * Rapid-prototyping protection schemes with IEC 61850 00003 * 00004 * Copyright (c) 2012 Steven Blair 00005 * 00006 * This program is free software; you can redistribute it and/or 00007 * modify it under the terms of the GNU General Public License 00008 * as published by the Free Software Foundation; either version 2 00009 * of the License, or (at your option) any later version. 00010 00011 * This program is distributed in the hope that it will be useful, 00012 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00013 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00014 * GNU General Public License for more details. 00015 00016 * You should have received a copy of the GNU General Public License 00017 * along with this program; if not, write to the Free Software 00018 * Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA. 00019 */ 00020 00021 #ifndef SV_PACKET_DATA_H 00022 #define SV_PACKET_DATA_H 00023 00024 #include <stdlib.h> 00025 #include <string.h> 00026 #include "ctypes.h" 00027 00028 #define SV_USE_VLAN 0 // set to "1" to insert VLAN tag into SV packets 00029 #define SV_OPTIONAL_SUPPORTED 0 // set to "1" to enable output of optional items in SV packets (Wireshark does not support these) 00030 #define SV_FIXED_SMPCNT_CONFREV_SIZE 1 // set to "1" to force smpCnt and confRev field to be fixed size, rather than BER encoded 00031 00032 #define SV_MAX_DATASET_SIZE 512//1024 00033 00034 struct ASDU { 00035 unsigned char *svID; 00036 unsigned char *datset; // optional 00037 CTYPE_INT16U smpCnt; 00038 CTYPE_INT32U confRev; 00039 //struct UtcTime refrTm; // optional 00040 CTYPE_TIMESTAMP refrTm; // optional 00041 CTYPE_BOOLEAN smpSynch; 00042 CTYPE_INT16U smpRate; // optional 00043 int showDatset; 00044 int showRefrTm; 00045 int showSmpRate; 00046 struct data { 00047 unsigned char data[SV_MAX_DATASET_SIZE]; 00048 CTYPE_INT32U size; 00049 } data; 00050 }; 00051 00052 struct svControl { 00053 struct ethHeaderData ethHeaderData; 00054 short noASDU; 00055 struct ASDU *ASDU; 00056 CTYPE_INT16U ASDUCount; // stores present ASDU count; transmit a packet when equals "noASDU" 00057 CTYPE_INT16U sampleCountMaster; 00058 int (*update)(unsigned char *buf); // function pointer to save next ASDU, and possible send SV packet 00059 }; 00060 00061 #endif
Generated on Sat Jul 23 2022 01:15:10 by
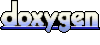