
ECE59500_ESP8266_K64_MQTT_Pub_Sub_client
Dependencies: mbed ESP8266Interface MbedJSONValue mbed-rtos MQTT JSON
rgbled.h
00001 #ifndef __RGBLED_H__ 00002 #define __RGBLED_H__ 00003 00004 // includes 00005 #include "mbed.h" 00006 00007 // defines 00008 enum color {none, red, yellow, green, cyan, blue, magenta}; 00009 00010 // rgb led 00011 class rgbled { 00012 private: 00013 DigitalOut _pin_red; 00014 DigitalOut _pin_green; 00015 DigitalOut _pin_blue; 00016 bool _on; 00017 bool _off; 00018 void _none(void); 00019 void _red(void); 00020 void _yellow(void); 00021 void _green(void); 00022 void _cyan(void); 00023 void _blue(void); 00024 void _magenta(void); 00025 public: 00026 rgbled(PinName pin_red, PinName pin_green, PinName pin_blue); 00027 void active(bool a); 00028 void set(color c); 00029 }; 00030 00031 #endif
Generated on Wed Jul 13 2022 19:17:17 by
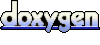