
ECE59500_ESP8266_K64_MQTT_Pub_Sub_client
Dependencies: mbed ESP8266Interface MbedJSONValue mbed-rtos MQTT JSON
main.cpp
00001 #include "MQTTESP8266.h" 00002 #include "MQTTClient.h" 00003 #include "string.h" 00004 #include "rgbled.h" 00005 #include "MbedJSONValue.h" 00006 char buf[100]; 00007 00008 MQTTESP8266 ipstack(D1, D0, D10, "Sidsap10","Arduino111"); // change to match your wifi access point 00009 float version = 0.47; 00010 char* topic = "k64"; 00011 char* topic2 = "k64rec"; 00012 char* topic3 = "bulb"; 00013 char* key2 = "key"; 00014 char* hostname = "192.168.43.87"; // Ip 00015 int port = 1883; 00016 int rc = ipstack.connect(hostname, port); 00017 unsigned int previousTime = 0; // will store last time LED was updated 00018 00019 // constants won't change: 00020 const long interval = 20; 00021 int flag =0; 00022 MQTT::Client<MQTTESP8266, Countdown> client = MQTT::Client<MQTTESP8266, Countdown>(ipstack); 00023 //MbedJSONValue demo; 00024 int arrivedcount = 0; 00025 //using namespace std; 00026 std::string my_str; 00027 std::string my_str2; 00028 std::string someString; 00029 std::string clearsky ("clear"); 00030 std::string clouds ("few clouds"); 00031 std::string snow ("light snow"); 00032 std::string automatic ("automatic"); 00033 std::string manual ("manual"); 00034 int j=0; 00035 rgbled rgb(PTB22, PTE26, PTB21); // create rgb object with pin definitions for FRDM-K64F board 00036 00037 // callback for subscribe topic 00038 00039 void subscribeCallback(MQTT::MessageData& md) 00040 { 00041 MQTT::Message &message = md.message; 00042 printf("Message received: qos %d, retained %d, dup %d, packetid %d\r\n", message.qos, message.retained, message.dup, message.id); 00043 00044 printf("%s",(char*)message.payload); 00045 printf("\r\n"); 00046 00047 printf("Payload %.*s\n", message.payloadlen, (char*)message.payload); 00048 printf("nonedited payload is %s and payload is %d\r\n",(char*)message.payload,message.payloadlen); 00049 00050 // int f = int(message.payload); 00051 00052 std::string someString((char*)message.payload); 00053 someString.resize (message.payloadlen); 00054 printf("Edited payload iss %s \r\n",someString.c_str()); 00055 //j= someString.find(clouds); 00056 //printf("j = %d \r\n", j); 00057 00058 if ( !(someString.find(clouds)) ) 00059 { 00060 sprintf(buf, "dCglDR-G9WoQUiig0XMNhOV4ozIEIVu3rWdBh5IlQ7d,cloud %s\n", key2); 00061 message.payload = (void*)buf; 00062 message.payloadlen = strlen(buf)+1; 00063 rc = client.publish(topic3, message); 00064 printf("if condition checked, its cloudy\r\n"); 00065 //rgb.set(red); 00066 00067 } 00068 else if ( !(someString.find(clearsky)) ) 00069 { 00070 sprintf(buf, "dCglDR-G9WoQUiig0XMNhOV4ozIEIVu3rWdBh5IlQ7d,clearsky %s\r\n", key2); 00071 message.payload = (void*)buf; 00072 message.payloadlen = strlen(buf)+1; 00073 rc = client.publish(topic3, message); 00074 printf("if condition checked, its clear\r\n"); 00075 00076 //rgb.set(yellow); 00077 } 00078 else if ( !(someString.find(snow)) ) 00079 { 00080 00081 sprintf(buf, "dCglDR-G9WoQUiig0XMNhOV4ozIEIVu3rWdBh5IlQ7d,snow %s\r\n",key2); 00082 message.payload = (void*)buf; 00083 message.payloadlen = strlen(buf)+1; 00084 rc = client.publish(topic3, message); 00085 printf("if condition checked, its snowy\r\n"); 00086 00087 // rgb.set(blue); 00088 } 00089 else if ( !(someString.find(automatic)) ) 00090 { 00091 00092 printf("Automatic, i.e Decide based on weather \r\n"); 00093 flag = 0; 00094 // rgb.set(blue); 00095 } 00096 else if ( !(someString.find(manual)) ) 00097 { 00098 00099 printf("Manual Flag set \r\n"); 00100 flag = 1; 00101 // rgb.set(blue); 00102 } 00103 else 00104 { 00105 printf("went into else\r\n"); 00106 } 00107 00108 } 00109 00110 int main(int argc, char* argv[]) 00111 { 00112 wait (1); 00113 printf("Starting\r\n"); 00114 00115 set_time(1256729737); 00116 time_t seconds = time(NULL); 00117 previousTime = (unsigned int)seconds; 00118 00119 00120 printf("Version is %f\r\n", version); 00121 00122 rgb.active(false); // set led output to active low 00123 rgb.set(none); // set led output of all off 00124 00125 00126 00127 if (rc != 0) 00128 printf("rc from TCP connect is %d\r\n", rc); 00129 00130 MQTTPacket_connectData data = MQTTPacket_connectData_initializer; 00131 //data.MQTTVersion = 3; 00132 //data.clientID.cstring = "mbed-clientID"; 00133 //data.username.cstring = "testuser"; 00134 //data.password.cstring = "testpassword"; 00135 00136 if ((rc = client.connect(data)) != 0) 00137 printf("rc from MQTT connect is %d\r\n", rc); 00138 00139 if ((rc = client.subscribe(topic2, MQTT::QOS1, subscribeCallback)) != 0) 00140 printf("Recv'd from MQTT subscribe is %d\r\n", rc); 00141 00142 MQTT::Message message; 00143 // QoS 0 00144 00145 00146 sprintf(buf, "fa02d31610ce9ea0de15b22bb2fe279d, %s\r\n", key2); 00147 message.qos = MQTT::QOS0; 00148 message.retained = false; 00149 message.dup = false; 00150 message.payload = (void*)buf; 00151 message.payloadlen = strlen(buf)+1; 00152 00153 00154 rc = client.publish(topic, message); 00155 wait(5); 00156 00157 /* 00158 sprintf(buf, "dCglDR-G9WoQUiig0XMNhOV4ozIEIVu3rWdBh5IlQ7d, %s\r\n", key2); 00159 message.payload = (void*)buf; 00160 message.payloadlen = strlen(buf)+1; 00161 rc = client.publish(topic2, message); 00162 */ 00163 00164 while (arrivedcount < 10) 00165 { client.yield(100); 00166 00167 time_t seconds = time(NULL); 00168 //printf("%u\r\n", (unsigned int)seconds); 00169 wait(1); 00170 if ( seconds - previousTime >= interval ) 00171 { 00172 if (flag ==0) 00173 { 00174 //printf("its been a few seconds \r\n"); 00175 printf("Checking the weather\r\n"); 00176 previousTime =seconds; 00177 sprintf(buf, "fa02d31610ce9ea0de15b22bb2fe279d, %s\r\n", key2); 00178 message.qos = MQTT::QOS0; 00179 message.retained = false; 00180 message.dup = false; 00181 message.payload = (void*)buf; 00182 message.payloadlen = strlen(buf)+1; 00183 rc = client.publish(topic, message); 00184 } 00185 } 00186 } 00187 00188 00189 /* 00190 // QoS 1 00191 sprintf(buf, "Hello World! QoS 1 message from app version %f\r\n", version); 00192 message.qos = MQTT::QOS1; 00193 message.payloadlen = strlen(buf)+1; 00194 rc = client.publish(topic, message); 00195 while (arrivedcount < 10) 00196 client.yield(100); 00197 00198 // QoS 2 00199 sprintf(buf, "Hello World! QoS 2 message from app version %f\r\n", version); 00200 message.qos = MQTT::QOS2; 00201 message.payloadlen = strlen(buf)+1; 00202 rc = client.publish(topic, message); 00203 while (arrivedcount < 3) 00204 client.yield(100); 00205 00206 // n * QoS 2 00207 00208 for (int i = 1; i <= 10; ++i) { 00209 sprintf(buf, "Hello World! QoS 2 message number %d from app version %f\r\n", i, version); 00210 message.qos = MQTT::QOS2; 00211 message.payloadlen = strlen(buf)+1; 00212 rc = client.publish(topic, message); 00213 while (arrivedcount < i + 3) 00214 client.yield(100); 00215 } 00216 */ 00217 00218 if ((rc = client.unsubscribe(topic)) != 0) 00219 printf("rc from unsubscribe was %d\r\n", rc); 00220 00221 if ((rc = client.disconnect()) != 0) 00222 printf("rc from disconnect was %d\r\n", rc); 00223 00224 //ipstack.disconnect(); 00225 //printf("Finishing with %d messages received\r\n", arrivedcount); 00226 00227 return 0; 00228 }
Generated on Wed Jul 13 2022 19:17:17 by
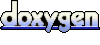