Assert updated with the returned status code instead of URL
Dependencies: ublox-at-cellular-interface
main.cpp
00001 #include "mbed.h" 00002 #include "greentea-client/test_env.h" 00003 #include "unity.h" 00004 #include "utest.h" 00005 #include "UbloxATCellularInterfaceExt.h" 00006 #include "UDPSocket.h" 00007 #ifdef FEATURE_COMMON_PAL 00008 #include "mbed_trace.h" 00009 #define TRACE_GROUP "TEST" 00010 #else 00011 #define tr_debug(format, ...) debug(format "\n", ## __VA_ARGS__) 00012 #define tr_info(format, ...) debug(format "\n", ## __VA_ARGS__) 00013 #define tr_warn(format, ...) debug(format "\n", ## __VA_ARGS__) 00014 #define tr_error(format, ...) debug(format "\n", ## __VA_ARGS__) 00015 #endif 00016 00017 using namespace utest::v1; 00018 00019 // ---------------------------------------------------------------- 00020 // COMPILE-TIME MACROS 00021 // ---------------------------------------------------------------- 00022 00023 // These macros can be overridden with an mbed_app.json file and 00024 // contents of the following form: 00025 // 00026 //{ 00027 // "config": { 00028 // "apn": { 00029 // "value": "\"my_apn\"" 00030 // }, 00031 // "run-tcp-server-test": { 00032 // "value": 1 00033 // }, 00034 // "mga-token": { 00035 // "value": "\"my_token\"" 00036 // } 00037 //} 00038 00039 // Whether debug trace is on 00040 #ifndef MBED_CONF_APP_DEBUG_ON 00041 # define MBED_CONF_APP_DEBUG_ON false 00042 #endif 00043 00044 // The credentials of the SIM in the board. 00045 #ifndef MBED_CONF_APP_DEFAULT_PIN 00046 // Note: if PIN is enabled on your SIM, you must define the PIN 00047 // for your SIM jere (e.g. using mbed_app.json to do so). 00048 # define MBED_CONF_APP_DEFAULT_PIN "0000" 00049 #endif 00050 00051 // Network credentials. 00052 #ifndef MBED_CONF_APP_APN 00053 # define MBED_CONF_APP_APN NULL 00054 #endif 00055 #ifndef MBED_CONF_APP_USERNAME 00056 # define MBED_CONF_APP_USERNAME NULL 00057 #endif 00058 #ifndef MBED_CONF_APP_PASSWORD 00059 # define MBED_CONF_APP_PASSWORD NULL 00060 #endif 00061 00062 #ifndef MBED_CONF_APP_RUN_CELL_LOCATE_TCP_SERVER_TEST 00063 # define MBED_CONF_APP_RUN_CELL_LOCATE_TCP_SERVER_TEST 0 00064 #endif 00065 00066 // The authentication token for TCP access to the MGA server. 00067 #if MBED_CONF_APP_RUN_CELL_LOCATE_TCP_SERVER_TEST 00068 # ifndef MBED_CONF_APP_CELL_LOCATE_MGA_TOKEN 00069 # error "You must have a token for MGA server access to run Cell Locate with a TCP server" 00070 # endif 00071 #endif 00072 00073 // The type of response requested 00074 #ifndef MBED_CONF_APP_RESP_TYPE 00075 # define MBED_CONF_APP_RESP_TYPE 1 // CELL_DETAILED 00076 #endif 00077 00078 // The maximum number of hypotheses requested 00079 #ifndef MBED_CONF_APP_CELL_LOCATE_MAX_NUM_HYPOTHESIS 00080 # if MBED_CONF_APP_RESP_TYPE == 2 // CELL_MULTIHYP 00081 # define MBED_CONF_APP_CELL_LOCATE_MAX_NUM_HYPOTHESIS 16 00082 # else 00083 # define MBED_CONF_APP_CELL_LOCATE_MAX_NUM_HYPOTHESIS 1 00084 # endif 00085 #endif 00086 00087 #ifndef MBED_CONF_APP_CELL_LOCATE_MGA_TOKEN 00088 # define MBED_CONF_APP_CELL_LOCATE_MGA_TOKEN "0" 00089 #endif 00090 00091 // ---------------------------------------------------------------- 00092 // PRIVATE VARIABLES 00093 // ---------------------------------------------------------------- 00094 00095 #ifdef FEATURE_COMMON_PAL 00096 // Lock for debug prints 00097 static Mutex mtx; 00098 #endif 00099 00100 // Power up GNSS 00101 #ifdef TARGET_UBLOX_C030 00102 static DigitalInOut gnssEnable(GNSSEN, PIN_OUTPUT, PushPullNoPull, 1); 00103 #elif TARGET_UBLOX_C027 00104 static DigitalOut gnssEnable(GPSEN, 1); 00105 #endif 00106 00107 // An instance of the cellular interface 00108 static UbloxATCellularInterfaceExt *pDriver = 00109 new UbloxATCellularInterfaceExt(MDMTXD, MDMRXD, 00110 MBED_CONF_UBLOX_CELL_BAUD_RATE, 00111 MBED_CONF_APP_DEBUG_ON); 00112 00113 // ---------------------------------------------------------------- 00114 // PRIVATE FUNCTIONS 00115 // ---------------------------------------------------------------- 00116 00117 #ifdef FEATURE_COMMON_PAL 00118 // Locks for debug prints 00119 static void lock() 00120 { 00121 mtx.lock(); 00122 } 00123 00124 static void unlock() 00125 { 00126 mtx.unlock(); 00127 } 00128 #endif 00129 00130 static void printCellLocateData(UbloxATCellularInterfaceExt::CellLocData *pData) 00131 { 00132 char timeString[25]; 00133 00134 tr_debug("Cell Locate data:"); 00135 if (strftime(timeString, sizeof(timeString), "%F %T", (const tm *) &(pData->time)) > 0) { 00136 tr_debug(" time: %s", timeString); 00137 } 00138 tr_debug(" longitude: %.6f", pData->longitude); 00139 tr_debug(" latitude: %.6f", pData->latitude); 00140 tr_debug(" altitude: %d metre(s)", pData->altitude); 00141 switch (pData->sensor) { 00142 case UbloxATCellularInterfaceExt::CELL_LAST: 00143 tr_debug(" sensor type: last"); 00144 break; 00145 case UbloxATCellularInterfaceExt::CELL_GNSS: 00146 tr_debug(" sensor type: GNSS"); 00147 break; 00148 case UbloxATCellularInterfaceExt::CELL_LOCATE: 00149 tr_debug(" sensor type: Cell Locate"); 00150 break; 00151 case UbloxATCellularInterfaceExt::CELL_HYBRID: 00152 tr_debug(" sensor type: hybrid"); 00153 break; 00154 default: 00155 tr_debug(" sensor type: unknown"); 00156 break; 00157 } 00158 tr_debug(" uncertainty: %d metre(s)", pData->uncertainty); 00159 tr_debug(" speed: %d metre(s)/second", pData->speed); 00160 tr_debug(" direction: %d degree(s)", pData->direction); 00161 tr_debug(" vertical accuracy: %d metre(s)/second", pData->speed); 00162 tr_debug(" satellite(s) used: %d", pData->svUsed); 00163 tr_debug("I am here: " 00164 "https://maps.google.com/?q=%.5f,%.5f", pData->latitude, pData->longitude); 00165 } 00166 00167 // ---------------------------------------------------------------- 00168 // TESTS 00169 // ---------------------------------------------------------------- 00170 00171 // Test Cell Locate talking to a UDP server 00172 void test_udp_server() { 00173 int numRes = 0; 00174 UbloxATCellularInterfaceExt::CellLocData data; 00175 00176 memset(&data, 0, sizeof(data)); 00177 TEST_ASSERT(pDriver->connect(MBED_CONF_APP_DEFAULT_PIN, MBED_CONF_APP_APN, 00178 MBED_CONF_APP_USERNAME, MBED_CONF_APP_PASSWORD) == 0); 00179 00180 TEST_ASSERT(pDriver->cellLocSrvUdp()); 00181 TEST_ASSERT(pDriver->cellLocConfig(1)); 00182 TEST_ASSERT(pDriver->cellLocRequest(UbloxATCellularInterfaceExt::CELL_HYBRID, 10, 100, 00183 (UbloxATCellularInterfaceExt::CellRespType) MBED_CONF_APP_RESP_TYPE, 00184 MBED_CONF_APP_CELL_LOCATE_MAX_NUM_HYPOTHESIS)); 00185 00186 for (int x = 0; (numRes == 0) && (x < 10); x++) { 00187 numRes = pDriver->cellLocGetRes(); 00188 } 00189 00190 TEST_ASSERT(numRes > 0); 00191 TEST_ASSERT(pDriver->cellLocGetData(&data)); 00192 00193 TEST_ASSERT(data.validData); 00194 00195 printCellLocateData(&data); 00196 00197 TEST_ASSERT(pDriver->disconnect() == 0); 00198 // Wait for printfs to leave the building or the test result string gets messed up 00199 wait_ms(500); 00200 } 00201 00202 // Test Cell Locate talking to a TCP server 00203 void test_tcp_server() { 00204 int numRes = 0; 00205 UbloxATCellularInterfaceExt::CellLocData data; 00206 00207 memset(&data, 0, sizeof(data)); 00208 TEST_ASSERT(pDriver->connect(MBED_CONF_APP_DEFAULT_PIN, MBED_CONF_APP_APN, 00209 MBED_CONF_APP_USERNAME, MBED_CONF_APP_PASSWORD) == 0); 00210 00211 TEST_ASSERT(pDriver->cellLocSrvTcp(MBED_CONF_APP_CELL_LOCATE_MGA_TOKEN)); 00212 TEST_ASSERT(pDriver->cellLocConfig(1)); 00213 TEST_ASSERT(pDriver->cellLocRequest(UbloxATCellularInterfaceExt::CELL_HYBRID, 10, 100, 00214 (UbloxATCellularInterfaceExt::CellRespType) MBED_CONF_APP_RESP_TYPE, 00215 MBED_CONF_APP_CELL_LOCATE_MAX_NUM_HYPOTHESIS)); 00216 00217 for (int x = 0; (numRes == 0) && (x < 10); x++) { 00218 numRes = pDriver->cellLocGetRes(); 00219 } 00220 00221 TEST_ASSERT(numRes > 0); 00222 TEST_ASSERT(pDriver->cellLocGetData(&data)); 00223 00224 TEST_ASSERT(data.validData); 00225 00226 printCellLocateData(&data); 00227 00228 TEST_ASSERT(pDriver->disconnect() == 0); 00229 // Wait for printfs to leave the building or the test result string gets messed up 00230 wait_ms(500); 00231 } 00232 00233 // Tidy up after testing so as not to screw with the test output strings 00234 void test_tidy_up() { 00235 pDriver->deinit(); 00236 } 00237 00238 // ---------------------------------------------------------------- 00239 // TEST ENVIRONMENT 00240 // ---------------------------------------------------------------- 00241 00242 // Setup the test environment 00243 utest::v1::status_t test_setup(const size_t number_of_cases) { 00244 // Setup Greentea with a timeout 00245 GREENTEA_SETUP(540, "default_auto"); 00246 return verbose_test_setup_handler(number_of_cases); 00247 } 00248 00249 // Test cases 00250 Case cases[] = { 00251 Case("Cell Locate with UDP server", test_udp_server), 00252 #if MBED_CONF_APP_RUN_CELL_LOCATE_TCP_SERVER_TEST 00253 Case("Cell Locate with TCP server", test_tcp_server), 00254 #endif 00255 Case("Tidy up", test_tidy_up) 00256 }; 00257 00258 Specification specification(test_setup, cases); 00259 00260 // ---------------------------------------------------------------- 00261 // MAIN 00262 // ---------------------------------------------------------------- 00263 00264 int main() { 00265 00266 #ifdef FEATURE_COMMON_PAL 00267 mbed_trace_init(); 00268 00269 mbed_trace_mutex_wait_function_set(lock); 00270 mbed_trace_mutex_release_function_set(unlock); 00271 #endif 00272 00273 // Run tests 00274 return !Harness::run(specification); 00275 } 00276 00277 // End Of File 00278
Generated on Fri Jul 15 2022 22:48:28 by
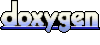